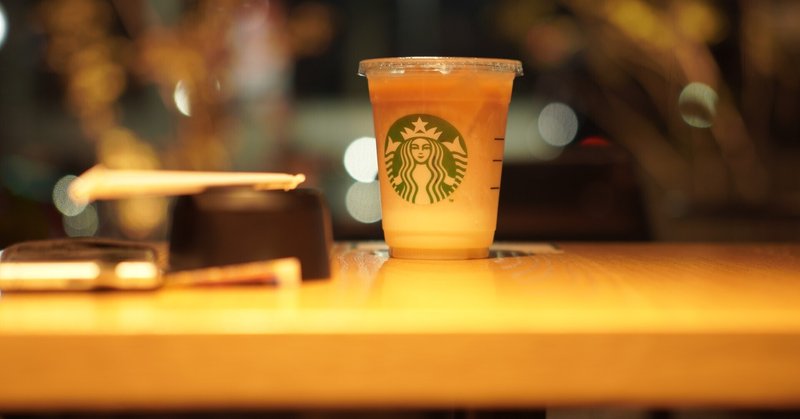
超初心者用:Javaプログラミング勉強会
ぶっちゃけ、メモです。
自分は結城さんの本でJavaを覚えました。
という事で、この本をベースで勉強会が出来たらいいなぁ。
学習内容とか進めるペースとかはやりながら考えるとして。
対象者
Java言語の完全初心者。
学習方式
勉強会方式とします。
理由としては、誰かに教わるのではなく本を基礎として自分達で学習できる経験を体験して欲しいので。
やりながら徐々に方式をブラッシュアップしていきたいです。
修了時
「Java言語でプログラム書けます」と言える。
課題本
事前準備
開発環境の準備
Eclipseのインストール(開発環境)
・Eclipseのバージョン:Pleiades All in One Eclipse/2022/Ultimate/Full Edition
・ファイルサイズが大きいので結構時間がかかります。余裕をもって準備しましょう。
学習内容
第1回 第0章から第1章 2022/05/11
プログラムはプログラム言語で書くんですよ。
IDE(総合開発環境)Eclipseを使ってJavaファイルを作成する。
・Projectの作成
・Javaファイルの作成
演習問題の実施
・Javaで標準出力に文字を出力する。
・Javaで簡単な文字列操作を実施する。
問題1-3
public class Kantan {
public static void main(String[] args) {
// TODO 自動生成されたメソッド・スタブ
System.out.println("Javaって意外と簡単だ。");
System.out.println("Javaって意外と簡単だ。");
System.out.println("Javaって意外と簡単だ。");
}
}
問題1-4
public class Aisatsu {
public static void main(String[] args) {
System.out.println(
"おはよう。" + System.lineSeparator()
+ "こんにちわ。" + System.lineSeparator()
+ "こんばんわ。"
);
}
}
振り返り
・40分は意外と短いので、時間配分をしっかりやろう。
・やった感というか、充実感を出す為にはどうやったら良いのかを考える。
第2回 第2章 2022/05/18
Javaで四則演算をやってみましょう。
問題2-2
package section2;
public class Calc2 {
public static void main(String[] args) {
System.out.println( 1 * 1);
System.out.println( 2 * 2);
System.out.println( 3 * 3);
System.out.println( 4 * 4);
System.out.println( 5 * 5);
System.out.println( 6 * 6);
System.out.println( 7 * 7);
System.out.println( 8 * 8);
System.out.println( 9 * 9);
System.out.println(10 * 10);
}
}
問題2-3
package section2;
public class Calc3 {
public static void main(String[] args) {
// 台形の面積を求める
System.out.println(
((2+3)*4)/2
);
}
}
問題2-4
package section2;
public class Calc4 {
public static void main(String[] args) {
System.out.println(100000 * 100000 );
System.out.println(100000L * 100000L);
}
}
振り返り
・Eclipseは「Ctrl+Space」で保管されます。
困ったら「Ctrl+Space」を押そう!
第3回 第3章 変数と型 2022/05/25
前半は授業形式。
後半は演習。
さあ、やっとプログラム作ってる気になれるぞ!
良く使うページ。https://codic.jp/engine
問題3-1
package section3;
import java.math.BigDecimal;
public class Question1 {
public static void main(String[] args) {
// 光の速さ[km/sec]
final BigDecimal lightSpeed = new BigDecimal(30);
// 1日(秒)
final BigDecimal day = new BigDecimal(24*60*60);
// 計算
final BigDecimal result = lightSpeed.multiply(day);
System.out.println(result.longValue() + " 万km/day");
}
}
問題3-2
Java的に書いてみた。
package section3;
import java.math.BigDecimal;
public class Question2 {
public static void main(String[] args) {
try {
final PersonCollection persons = new PersonCollection();
// 一人目
persons.add();
// 二人目
persons.add();
// 平均を計算する
final BigDecimal ave = persons.getAverageAge();
System.out.println("平均の年齢は" + ave + "歳です。");
} catch (Exception e) {
e.printStackTrace();
}
}
}
package section3;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
public class PersonCollection {
final List<PersonValue> _collection = new ArrayList<>();
/**
* 人を登録します。
* @return
* @throws IOException
*/
public PersonCollection add() throws IOException {
_collection.add(new PersonValue());
return this;
}
/**
* 登録された人の年齢の平均を計算します。
* @return
*/
public BigDecimal getAverageAge() {
final BigDecimal size = new BigDecimal(_collection.size());
final BigDecimal sum = this.getSumAge();
final BigDecimal ret = sum.divide(size);
System.out.println(sum + "/" + size );
System.out.println("平均は" + ret + "歳です。");
return ret;
}
/**
* 登録された人の年齢の合計を計算します。
* @return
*/
public BigDecimal getSumAge() {
BigDecimal ret = BigDecimal.ZERO;
for (final PersonValue p : _collection) {
ret = ret.add(p.getAge());
}
System.out.println("合計は" + ret + "歳です。");
return ret;
}
}
package section3;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.math.BigDecimal;
public class PersonValue {
final BufferedReader reader =
new BufferedReader(new InputStreamReader(System.in));
String name = "";
BigDecimal age = BigDecimal.ZERO;
public PersonValue() throws IOException {
this
.setName()
.setAge();
}
private PersonValue setName() throws IOException {
System.out.println("名前を入力して下さい。");
this.name = this.readLine();
return this;
}
private PersonValue setAge() {
System.out.println("年齢を入力して下さい。");
try {
this.age = new BigDecimal(this.readLine());
} catch (Exception e) {
System.out.println("数値入れてね");
this.setAge();
}
return this;
}
public BigDecimal getAge() {
return this.age;
}
private String readLine() throws IOException {
return reader.readLine();
}
}
問題3-3
package section3;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Question3 {
public static void main(String[] args) throws IOException {
final String input = in("名前を入力して下さい。");
for (int i = 0; i < input.length(); i++) {
final char chara = input.charAt(i);
System.out.println(chara + "の文字コードは" + (int)chara + "です。");
}
}
private static final BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
private static String in(final String msg) throws IOException {
System.err.println(msg);
final String input = reader.readLine();
return input;
}
}
振り返り
・90分くらいやると結構満足。
・Objectの概念を伝えるのは難しい。どう伝えたら納得感がでるのか?
第4回 第4章から第5章 2022/6/1
IF文、Switch文
問題4-3
package section4;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class Question3 {
public static void main(String[] args) {
final BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
try {
final int key = Integer.parseInt(reader.readLine());
if (0 <= key && key <= 11) {
System.out.println("おはようございます");
} else if (key == 12) {
System.out.println("お昼です");
} else if (13 <= key && key <= 18) {
System.out.println("こんにちわ");
} else if (19 <= key && key <= 23) {
System.out.println("こんばんわ");
} else {
System.out.println("時刻の範囲を超えてます");
}
} catch (Exception e) {
System.out.println("時刻の範囲を超えてます");
}
}
}
問題5-6
package section5;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Question6 {
public static void main(String[] args) throws IOException {
System.out.println("飲み物は何が好きですか?");
System.out.println("あ:オレンジジュース");
System.out.println("い:コーヒーです。");
System.out.println("それ以外:どちらでもありません");
final BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
final String key = reader.readLine();
switch (key) {
case "あ": {
System.out.println("オレンジジュースです。");
break;
}
case "い": {
System.out.println("コーヒーです。");
break;
}
default:{
System.out.println("どちらでもありません");
}
}
}
}
振り返り
この回からみんなのコードを見る(発表?)するようにしました。
新しい発見があるので楽しい。
目から鱗というか、そういう書き方もあるのか、という発見。
第5回 第6章から第7章 2022/6/8
for文、While文
問題6-4は宿題で、7-3に集中する事にしました。
初の宿題。やってもやらなくても良いですよ。という宿題。
問題6-4
問題7-3
package section7;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Question3 {
public static void main(String[] args) throws IOException {
System.out.println("ファイルパスを入力して下さい。");
final String fileName = new BufferedReader(new InputStreamReader(System.in)).readLine();
final BufferedReader reader =
new BufferedReader(
new FileReader(new File(fileName))
);
System.out.println(
new StringBuilder().append(System.lineSeparator())
.append("<!DOCTYPE html>").append(System.lineSeparator())
.append("<html>").append(System.lineSeparator())
.append("<head>").append(System.lineSeparator())
.append("<title>My Page</title>").append(System.lineSeparator())
.append("</head>").append(System.lineSeparator())
.append("<body>").append(System.lineSeparator())
);
while (true) {
final String line = reader.readLine();
if(line == null) {
break;
}
final String prefix;
if(line.length() == 0) {
prefix = "";
}
else {
prefix = line.charAt(0) + "";
}
switch (prefix) {
case "■": {
System.out.println(
new StringBuilder()
.append("<h1>").append(line.substring(1)).append("</h1>")
);
break;
}
case "●": {
System.out.println(
new StringBuilder()
.append("<h2>").append(line.substring(1)).append("</h2>")
);
break;
}
default: {
System.out.println(
new StringBuilder()
.append("<p>").append(line).append("</p>")
);
break;
}
}
}
System.out.println(
new StringBuilder().append(System.lineSeparator())
.append("</body>").append(System.lineSeparator())
.append("</html>").append(System.lineSeparator())
);
}
}
振り返り
宿題は皆さんやってきたエライ。
コンパイルエラーがとれないとか色々。何処にどんな{}が足りないとかどうやったら簡単に判断できるのかな?って思った。
あと、Stringの判定はequalsメソッドを使おうとか。primitiveとObjectの話をしました。Objectはnewされるので、「==」だと判定出来ないですよ、とか。まだ早いけど。
あと、1文字目の判定はString.startsWith使うと確かに簡単に判定出来ますね。なるほど。
てか、ApacheのStringUtilsとかFileUtilesとか使いまくっているので、ライブラリ無いと迷っちゃいます。
第6回 第8章 2022/6/15
メソッド
前半は講義。
後半は演習。
問題8-2
問題8-5
振り返り
第7回 第9章から第10章 6/22
配列とオブジェクト指向に向けて
第11章
クラスとインスタンスの説明
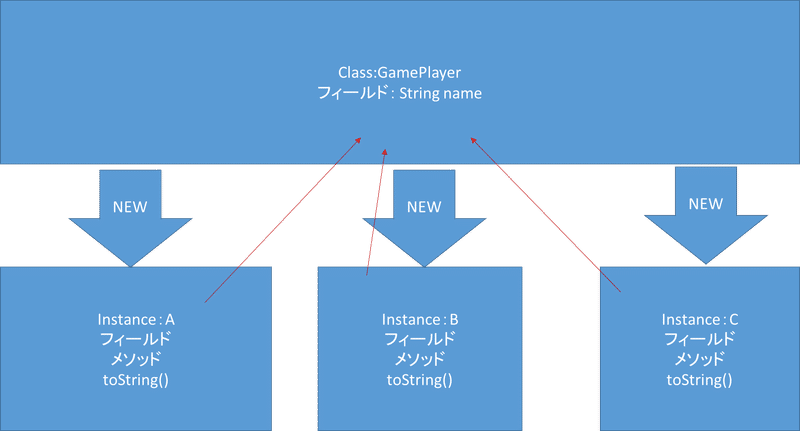
public class GamePlayer{
private static String playername = "";
private int score = 0;
public GamePlayer(final String name) {
playername = name;
}
@Override
public String toString() {
return "[playername=" + playername + " score=" + score + "]";
}
}
import java.util.ArrayList;
import java.util.List;
public class Test {
public static void main(String[] args) {
final List<GamePlayer> player = new ArrayList<>();
player.add(new GamePlayer("Mad Hatter"));
player.add(new GamePlayer("Alice"));
player.add(new GamePlayer("Hoge"));
for (GamePlayer gamePlayer : player) {
System.out.println(gamePlayer.toString());
}
}
}
余程の理由がない限り記事は無料です。読まれた方が何かしら刺激を受け、そして次の良いものが生まれてくれると嬉しいです。