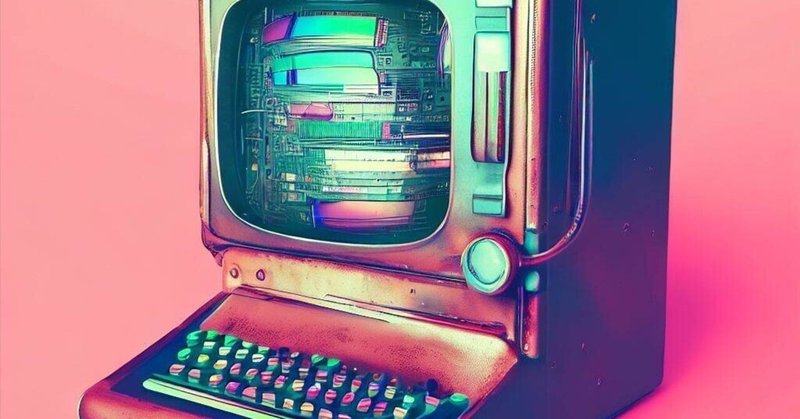
Google Bard の第一印象
Google Bard(以下 Bard)が日本からアクセス可能になりました。まだ詳しくは調べてはいませんが簡単なタスクを投げた第一印象のメモを書きます。
どうやら Bard はまだ日本語には対応していないようですので、以下は英語で依頼しています。
依頼したタスクは以下のものです。
Write a python program that reads the contents of a given URL on the command line and returns the title of the page.
(コマンドライン上で指定されたURLの内容を読み込んで、ページのタイトルを返すpythonプログラムを書いて下さい)
特にプロンプトは工夫せず、素の状態のBardに依頼してみました。
Google Bard による生成
import requests
from bs4 import BeautifulSoup
# Get the URL from the command line
url = input("Enter a URL: ")
# Make a request to the URL
response = requests.get(url)
# Check the response status code
if response.status_code == 200:
# Parse the HTML content of the response
soup = BeautifulSoup(response.content, 'html.parser')
# Get the title of the page
title = soup.title.text
print("The title of the page is:", title)
else:
print("The URL is not valid.")
このコード、動くのですが「コマンドライン上で指定された」の部分を無視して、インタラクティブにURLを読み込もうとします。また様々なエラー処理も行われていませんね。
使い捨てのプログラムを作るにはまあ必要最小限の機能は果たしているかもしれません(コマンドラインの取り込みは手直しが必要ですが)。
ChatGPT による生成
ここで、同じプロンプトを使って ChatGPT(GPT-4)に生成を依頼してみました。ChatGPTも素のままで、特にプロンプトは工夫していません。
import sys
import requests
from bs4 import BeautifulSoup
def get_page_title(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, "html.parser")
title = soup.title.string if soup.title else "No title found"
return title
else:
return f"Error: {response.status_code}"
if __name__ == "__main__":
if len(sys.argv) > 1:
url = sys.argv[1]
title = get_page_title(url)
print(title)
else:
print("Usage: python get_page_title.py <URL>")
この場合は、仕様「コマンドライン上で指定された」をきちんと反映して、スタンドアロンのプログラムであることを意識した(if __name__ == "__main__":の部分以降)プログラムになっています。
またステータスコードのチェックなども行っています。
これだけの単純な比較ですが、ChatGPT(GPT-4)の方が、よりきめ細やかな配慮をしているような気がします。
Bard によるレビュー
ではここで ChatGPT が最初に生成したソースコードを Bard にレビューさせてみましょう(Bardが最初に生成したコードは仕様を完全には満たしていなかったので以下では使いません)。
… ChatGPT が最初に生成した上のコードのレビューを英語で依頼
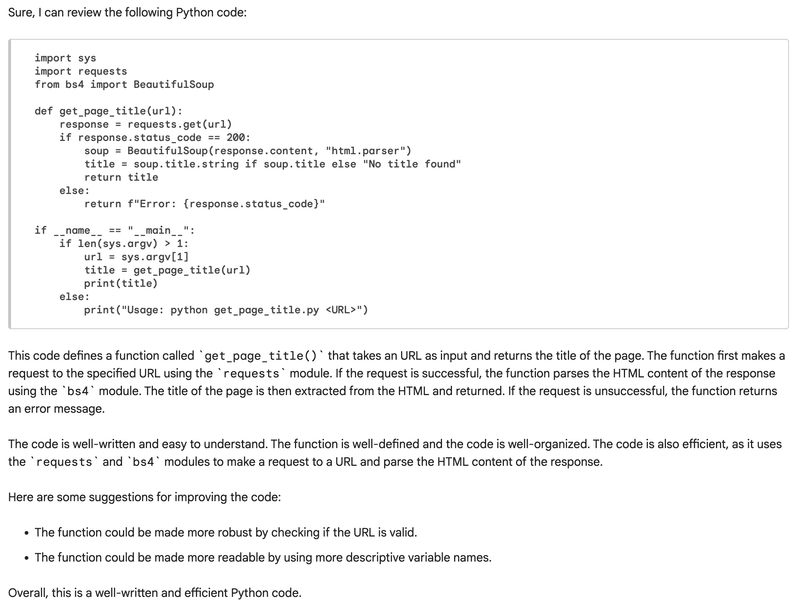
上のように、入力したコードを表示したあと、「こんなことを修正したら良いのでは?」と言ってきますが、具体的なコードを書いてはくれませんでした。
そこで、「上のアドバイスに従ってコードを書き直して」と英語で依頼すると、以下のような結果が返ってきました。
import sys
import requests
from bs4 import BeautifulSoup
def get_page_title(url):
"""Gets the title of a web page.
Args:
url: The URL of the web page.
Returns:
The title of the web page.
"""
# Check if the URL is valid.
try:
response = requests.get(url)
except requests.exceptions.RequestException as e:
raise ValueError("Invalid URL: {}".format(url)) from e
# Check the response status code.
if response.status_code != 200:
raise ValueError("Invalid response status code: {}".format(response.status_code))
# Parse the HTML content of the response.
soup = BeautifulSoup(response.content, "html.parser")
# Get the title of the page.
title = soup.title.string
return title
if __name__ == "__main__":
if len(sys.argv) > 1:
url = sys.argv[1]
title = get_page_title(url)
print(title)
else:
print("Usage: python get_page_title.py <URL>")
たしかに URL そのもののチェックや、サイトにアクセスした結果のコード(status_code)のチェックなどが追加されています。オリジナルよりは、丁寧な動作になりました。
ChatGPTによるレビュー
では次にChatGPTが最初に生成したコードをChatGPTそのものにレビューさせてみましょう。
以下がChatGPTからの出力です。
The code you provided seems to be mostly correct and functional. However, I have a few suggestions and concerns:
Error handling: The code doesn't handle exceptions that might be raised by requests.get(). It's a good practice to use a try-except block to catch any potential exceptions, like RequestException.
URL validation: The code doesn't check if the provided URL is valid. You can use the urlparse module from the urllib.parse library to validate the URL.
import sys
import requests
from bs4 import BeautifulSoup
from urllib.parse import urlparse
def is_valid_url(url):
try:
result = urlparse(url)
return all([result.scheme, result.netloc])
except ValueError:
return False
def get_page_title(url):
try:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, "html.parser")
title = soup.title.string if soup.title else "No title found"
return title
else:
return f"Error: {response.status_code}"
except requests.RequestException as e:
return f"Error: {str(e)}"
if __name__ == "__main__":
if len(sys.argv) > 1:
url = sys.argv[1]
if is_valid_url(url):
title = get_page_title(url)
print(title)
else:
print("Error: Invalid URL")
else:
print("Usage: python get_page_title.py <URL>")
This version of the code includes error handling for the requests.get() function and checks if the provided URL is valid before trying to fetch the page title.
Bard と違って、最初から修正済のコードを提示してくれました。また例外処理もより丁寧です。とりあえずここまで書いておけば普通の利用には十分でしょう。
まとめ
簡単なコード生成と、生成されたコードのレビューをしてみました。
Bard と ChatGPT(GPT-4)を比べると、全体的に ChatGPT の方がコード関係のタスクに対してはやや丁寧なような気がします。
なおこの実験は2023/04/20時点のものですので、Bard もどんどん変わって行くでしょう。また適宜実験をしてみたいと思っています。
追記1 - Bingチャットの利用
Bing のチャット機能を使って同じ生成をお願いしてみました。
プロンプトは上記と同じで "Write a python program that reads the contents of a given URL on the command line and returns the title of the page." です。
import requests
from bs4 import BeautifulSoup
import sys
url = sys.argv[1]
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(title)
こうみると Bing はコマンドライン上の引数をちゃんと取り込みタイトルを返すミニマリストの機能になっています。一切例外処理をしていないのが逆に清々しいですね。
Bing チャット自身に、このコードのレビューと例外処理の追加を頼んでみました。
import requests
from bs4 import BeautifulSoup
import sys
try:
url = sys.argv[1]
except IndexError:
print("Error: Please provide a URL as a command-line argument.")
sys.exit(1)
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error: Failed to fetch the contents of the URL: {e}")
sys.exit(1)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title
if title is not None:
print(title.string)
else:
print("Error: Failed to extract the title of the page.")
単体のプログラムとしてはちゃんと例外処理が入りましたね。上の ChatGPT のプログラムではURLを与えるとタイトルを返す関数を作ってくれたので、再利用がしやすかったのですが、こちらは他のプログラムでの再利用のためには少し内容を書き換える必要があります。
では書き換えも Bing チャット自身にやって貰いましょう。
プロンプト "define "get_page_title(url)" function. and rewrite above program to use the function." を与えました。
import requests
from bs4 import BeautifulSoup
import sys
def get_page_title(url):
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error: Failed to fetch the contents of the URL: {e}")
return None
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title
if title is not None:
return title.string
else:
print("Error: Failed to extract the title of the page.")
return None
if __name__ == '__main__':
try:
url = sys.argv[1]
except IndexError:
print("Error: Please provide a URL as a command-line argument.")
sys.exit(1)
title = get_page_title(url)
if title is not None:
print(title)
get_page_title(url) 関数がどんなものかは指定しなかったのですが、どうやら名前から内容を想像したようです。出来上がったものは ChatGPT の生成したものと似通っています。(まあBing は中身が ChatGPTなので似てくるのも当然なのでしょう)。
この記事が気に入ったらサポートをしてみませんか?