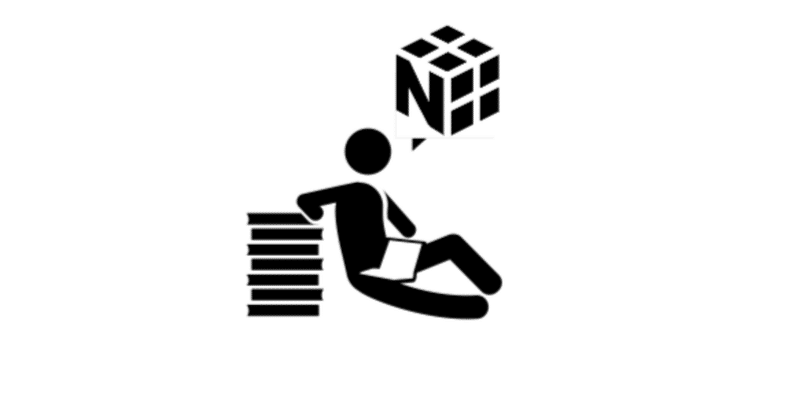
Trying DataScience(2) : Cramming Numpy
Hello, everyone!
Last time, I briefly covered the basics of Python for data science.
Today, I took the next step and studied NumPy, which is a linear algebra library for Python. It is a basic module for data science!
NumPy is usually used with other libraries like Pandas(data anaysis library) and Matplotlib(graphic package).
It is usually called like this.
import numpy as np
Let's get started.
1. arange
It returns evenly spaced values within a given interval.
In
np.arange(0,10)
Out
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
In
np.arange(0,11,2)
Out
array([ 0, 2, 4, 6, 8, 10])
2. linspace
It returns evenly spaced numbers over a specified interval.
In
np.linspace(0,10,3)
Out
array([ 0., 5., 10.])
3. eye
It creates an identity matrix. What is an identity matrix?
An identity matrix is a square matrix in which all the elements of the main diagonal are equal to 1, and all other elements are equal to 0.
In
np.eye(3)
Out
array([[ 1., 0., 0.],
[ 0., 1., 0.],
[ 0., 0., 1.]])
4. rand
It creates an array of the given shape and populate it with random samples.
In
np.random.rand(5,5)
Out
array([ 0.11570539, 0.35279769])
5. randint
It returns random integers from low (inclusive) to high (exclusive).
In
np.random.randint(1,100,10)
Out
array([13, 64, 27, 63, 46, 68, 92, 10, 58, 24])
6. reshape
It returns an array containing the same data with a new shape.
In
arr.reshape(5,5)
Out
array([[ 0, 1, 2, 3, 4],
[ 5, 6, 7, 8, 9],
[10, 11, 12, 13, 14],
[15, 16, 17, 18, 19],
[20, 21, 22, 23, 24]])
7. shape
It is an attribute that arrays have.
In
arr.reshape(1,25).shape
Out
(1, 25)
8. broadcasting ★
Numpy arrays differ from a normal Python list because of their ability to broadcast. It is a function that can perform operations by automatically adjusting the size of the array.
In
arr = np.arange(0,11)
arr
Out
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
In
#Setting values
arr[0:5]=100
arr
Out
array([100, 100, 100, 100, 100, 5, 6, 7, 8, 9, 10])
In
#Important notes on Slices
slice_of_arr = arr[0:6]
slice_of_arr
Out
array([0, 1, 2, 3, 4, 5])
In
#Change Slice
slice_of_arr[:]=99
slice_of_arr
Out
array([99, 99, 99, 99, 99, 99])
The changes occur in original array!
In
arr
Out
array([99, 99, 99, 99, 99, 99, 6, 7, 8, 9, 10])
[99, 99, 99, 99, 99, 99, 99] can be seen in the previous array.
9. indexing matrices
The general format is arr_2d[row][col] or arr_2d[row,col].
It is better to use the comma notation for clarity.
In
arr_2d = np.array(([5,10,15],[20,25,30],[35,40,45]))
arr_2d[1,0]
Out
20
In
arr_2d[:2,1:]
Out
array([[10, 15],
[25, 30]])
While studying, I summarized 9 points that I should remember. Especially, 'broadcasting', which is quite different from Python, was impressive. In the next session, I'm going to post about Pandas, a representative of the data analytics library.
エンジニアファーストの会社 株式会社CRE-CO
ソンさん
この記事が気に入ったらサポートをしてみませんか?