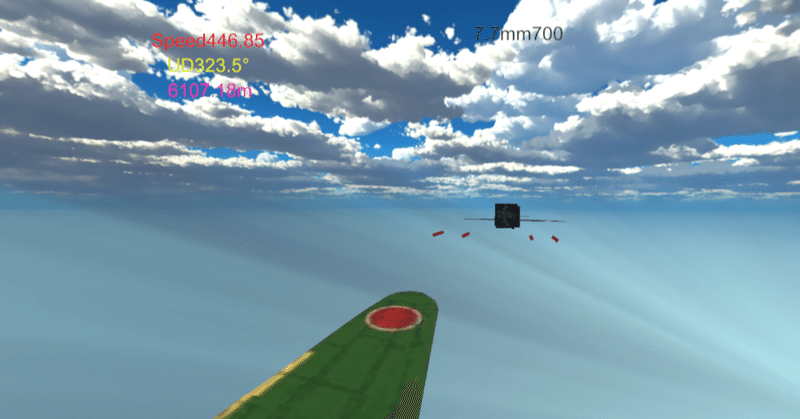
ゼロ戦で空中戦をしたい 武装する
零戦の武装は7.7mmと20mmがあるが、ここは7.7mmだけにする。20mmの弾数は50発で搭乗員も大型機にしか使わないので割愛する。Scriptは空のGameObject➡Script、空のGameObjectはScriptだけ欲しい場合によく使う。ゲームの中にある物は全て位置情報を持たなければならないからだ。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class ZeroShot : MonoBehaviour
{
public GameObject bulletPrefab;
public AudioClip shotSound;
public float shotSpeed;
public int shotCount = 700;
public Text shotLabel;
private float timeBetweenShot = 0.1f;
private float timer;
// ★火花を出す
public GameObject effectPrefab;
void Start()
{
shotLabel.text = "7.7mm" + shotCount;
}
void Update()
{
timer += Time.deltaTime;
{
if (Input.GetMouseButton(1) && timer > timeBetweenShot)
{
shotCount -= 1;
timer = 0.0f;
GameObject bullet = (GameObject)Instantiate(bulletPrefab, transform.position, Quaternion.identity);
Rigidbody bulletRb = bullet.GetComponent<Rigidbody>();
bulletRb.AddForce(transform.forward * shotSpeed);
Destroy(bullet, 1.0f);
AudioSource.PlayClipAtPoint(shotSound, Camera.main.transform.position);
// ★火花を出す
GameObject effect = (GameObject)Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.1f);
}
shotLabel.text = "7.7mm" + shotCount.ToString("0");
if (shotCount <= -1)
{
SceneManager.LoadScene("GameOver");
}
}
}
}
shotSoundとEffectが必要なければ行の先頭に//を付ければコンピューターは読み取らない決まりになっている。Scriptをアタッチしたら空のGameObjectの名前を変え発射したい場所に置けば良い。ただしこれは前方に飛ぶ設定になっている。Unityで前方はZ方向なので合わせる。7.7㎜の弾がなくなったらGameOverシーンに行くがこれはTextを含めて後日に説明する。Effectの作り方も後日に説明する。この部分はゲームがゲームらしく見えるのに大事だからだ。
BullitPrefabに入れる弾はGameObject➡Sphere、大きさは0.01でRigidbodyを付ける。真っ直ぐに飛ばす為に重力のチェックは外す、重さは少ない方が速く飛ぶ、shotSpeedはInspectorから好みで変えれる。
敵機の武装はRay(センサー)に反応したら撃つようにする。Scriptの中でRayの方向と距離を指定して、もしTagの名前に当たったら何をするかを教える。条件と行動を書くと簡単な人工智能になる。本来のRayCastは点だが高速で移動する物体に当たらないのでBoxの大きさを指定して当たりやすくしている。これでPlayerと言うTagを持つ物が300mに接近すればenemyShellが発射される。EnemyShellは7.7mmをDuplicate(複製)してTagの名前を変える。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyCubeRay : MonoBehaviour
{
public GameObject enemyShellPrefab;
public AudioClip shotSound;
public GameObject effectPrefab;
public float shotSpeed;
private float timeBetweenShot = 0.1f;
private float timer;
void Update()
{
RaycastHit hit;
if (Physics.BoxCast(transform.position, new Vector3(9.0f, 9.0f, 9.0f), transform.forward, out hit, Quaternion.identity, 300.0f))
if (hit.collider.tag == "Player")
{
GameObject enemyShell = (GameObject)Instantiate(enemyShellPrefab, transform.position, Quaternion.identity);
Rigidbody enemyShellRb = enemyShell.GetComponent<Rigidbody>();
enemyShellRb.AddForce(transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(enemyShell, 1.0f);
GameObject effect = (GameObject)Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.1f);
}
}
}
TagをInspectorで零戦はPlayer、敵機はEnemyに変える。7.7mmとEnemyShellのTagを変えるの忘れず正確に書く、大文字と小文字の違いだけでコンピューターは認識しない。
夢は100万の大軍を動かすゲームを作ることです。それまでには時間がかかりますが応援お願いします!