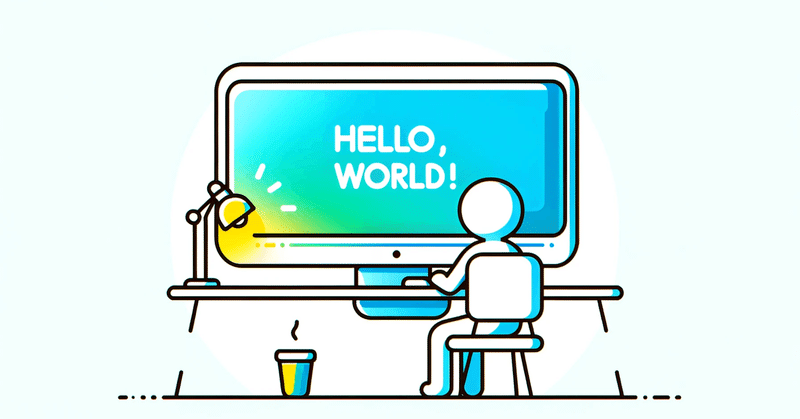
だったらテスト試験対策用アプリv1をJSで作っちゃえ
プログラマーでもないし、理系でもない僕がなんとなくWebアプリっぽいもの作たので共有するよ。
ただ、あまりクオリティが高くないし、バグがあっても修正も出来ないので、気楽に参考にしてね。
さて、受けるテストはVBAベーシック。
VBAでも簡単な方のテストね。
合格率は81%くらいなんだって。
前から気になっていたしVBAを仕事て少し使っているし、せっかくだからテスト受けてみようかな〜。なんて軽い気持ちで行動。
なかなか面白いし、テストに受かれば社内SEなんて道も……
まだまだ先ですね。
テストの勉強法
この教材を使ってテスト勉強
テキストもWebで使える演習問題もとても良い感じ。
ただWebアプリの演習問題は、問題回答
→回答終わったら答えと解説という流れ。
通常なんだけど少し僕にはまどろっこしい。
問題に回答したら、すぐに正誤と解説を知りたい。
クイズアプリで解決
じゃあまあ本題で、アプリを作ってみましょう。
気持ちはあるけど、VBAベーシックのテストを受けるくらいなので、もちろんプログラミングなんて素人。
どーするか。
Chat GPT4(有料版)の出番ですね。
本当は、バックエンドなんかもいじって「問題を作る」とか「間違えたところだけやり直す」みたいなボタンも実装したかったけど。
無理でした。
ChatGPT4に色々聞いてやっとそれらしく動いたんで、共有して行きますね。
見た目はこんな感じ
1 クイズを始めるシンプルすぎるボタン

2 問題と回答ボタン
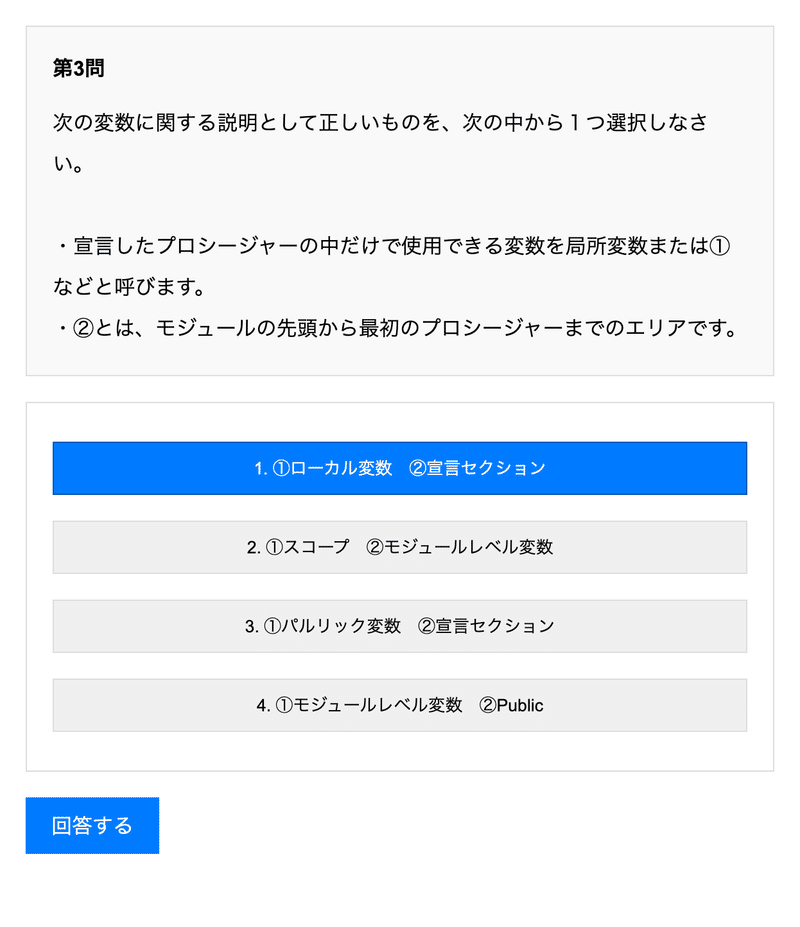
3 答えと解説
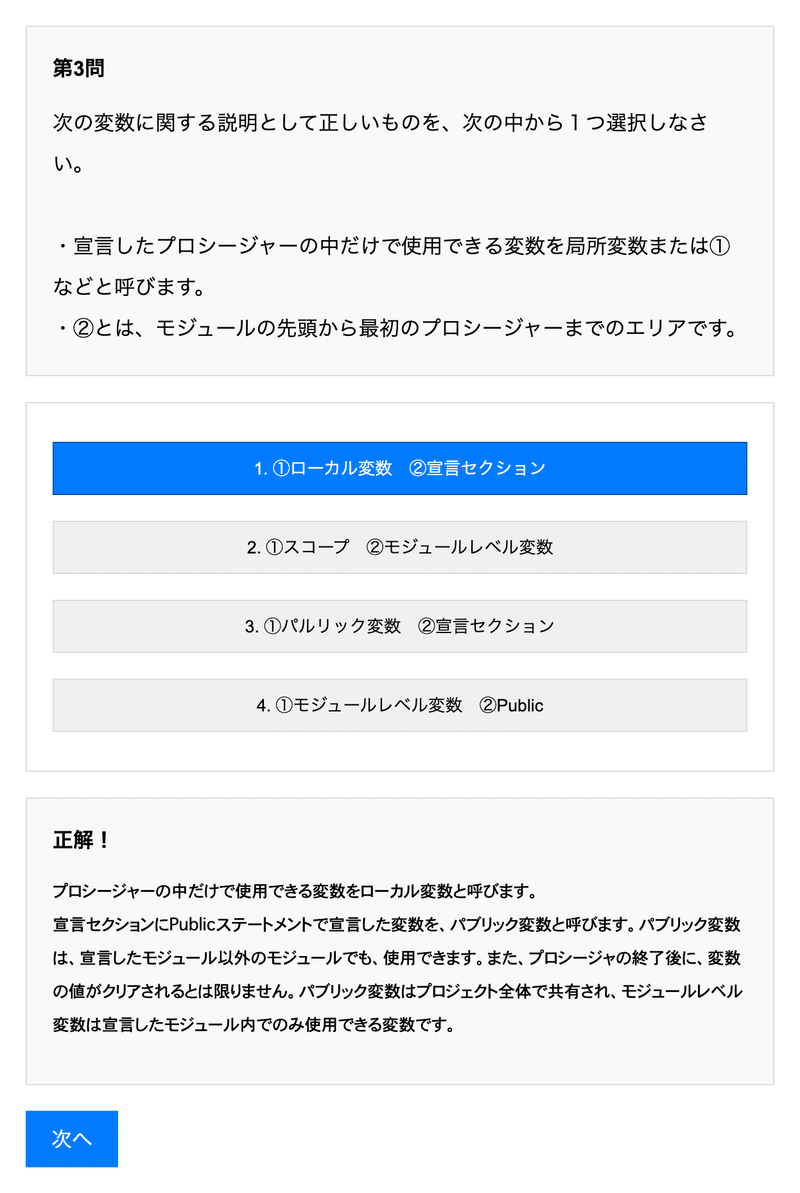
4 スコアと「ホームページに戻る」最初のページに戻ります。
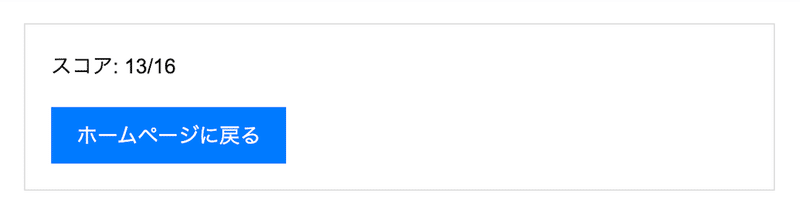
こんな感じの4択の構成ですね。
まずは環境ね
まずVScode使用
こんな感じの構成と名前を付けているよ。
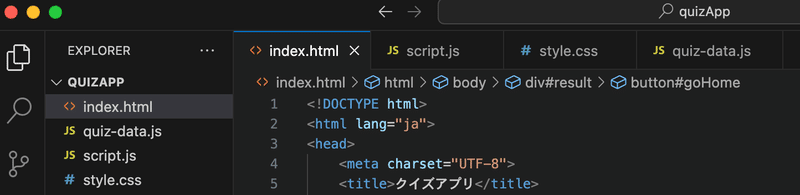
拡張機能は「Live Server」だけ入れているよ。
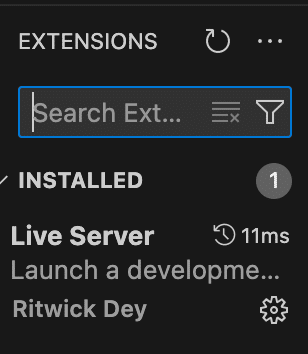
quiz-data.jsのところでクイズを追加修正していくよ。
コードを全て書いたら
index.htmlを開いておいて「Go Live」をクリックしてね。
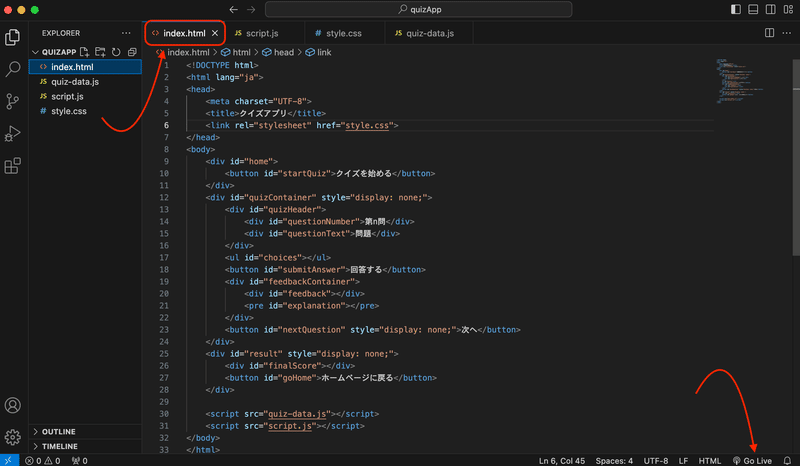
次はコード一覧ね
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>クイズアプリ</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="home">
<button id="startQuiz">クイズを始める</button>
</div>
<div id="quizContainer" style="display: none;">
<div id="quizHeader">
<div id="questionNumber">第n問</div>
<div id="questionText">問題</div>
</div>
<ul id="choices"></ul>
<button id="submitAnswer">回答する</button>
<div id="feedbackContainer">
<div id="feedback"></div>
<pre id="explanation"></pre>
</div>
<button id="nextQuestion" style="display: none;">次へ</button>
</div>
<div id="result" style="display: none;">
<div id="finalScore"></div>
<button id="goHome">ホームページに戻る</button>
</div>
<script src="quiz-data.js"></script>
<script src="script.js"></script>
</body>
</html>
script.js
let currentQuestionIndex = 0;
let score = 0;
let selectedChoice = ''; // 選択された選択肢を保存する変数
document.getElementById('startQuiz').addEventListener('click', function() {
document.getElementById('home').style.display = 'none';
document.getElementById('quizContainer').style.display = 'block';
showQuestion();
});
document.getElementById('submitAnswer').addEventListener('click', function() {
checkAnswer(selectedChoice);
document.getElementById('nextQuestion').style.display = 'block'; // 「次へ」ボタンを表示
document.getElementById('submitAnswer').style.display = 'none'; // 「回答する」ボタンを非表示
document.getElementById('feedbackContainer').style.display = 'block'; // 正誤と解説を表示
});
document.getElementById('nextQuestion').addEventListener('click', function() {
document.getElementById('feedbackContainer').style.display = 'none'; // 正誤と解説を非表示
document.getElementById('feedback').textContent = ''; // フィードバックをクリア
document.getElementById('explanation').textContent = ''; // 解説をクリア
if (currentQuestionIndex < quizData.length) {
showQuestion();
} else {
showResults();
}
document.getElementById('nextQuestion').style.display = 'none'; // 「次へ」ボタンを非表示
document.getElementById('submitAnswer').style.display = 'block'; // 「回答する」ボタンを再表示
});
function showQuestion() {
const question = quizData[currentQuestionIndex];
document.getElementById('questionNumber').textContent = `第${currentQuestionIndex + 1}問`;
document.getElementById('questionText').textContent = question.question;
const choicesContainer = document.getElementById('choices');
choicesContainer.innerHTML = ''; // 選択肢をクリア
question.choices.forEach(function(choice, index) {
const li = document.createElement('li');
const button = document.createElement('button');
button.textContent = `${index + 1}. ${choice}`;
button.addEventListener('click', function() {
// 他のすべての選択肢のアクティブスタイルを解除
const allChoices = document.querySelectorAll('#choices button');
allChoices.forEach(function(otherButton) {
otherButton.classList.remove('activeChoice');
});
// 現在の選択肢にアクティブスタイルを適用
button.classList.add('activeChoice');
// 選択された選択肢を保存
selectedChoice = choice;
});
li.appendChild(button);
choicesContainer.appendChild(li);
});
}
function checkAnswer(selectedChoice) {
const question = quizData[currentQuestionIndex];
const correctAnswerText = question.correctAnswer;
const correctChoiceIndex = question.choices.indexOf(correctAnswerText) + 1; // 正解の選択肢番号を取得
if (selectedChoice === correctAnswerText) {
score++;
document.getElementById('feedback').textContent = '正解!';
} else {
document.getElementById('feedback').textContent = `不正解。正解は${correctChoiceIndex}番です。`;
}
document.getElementById('explanation').textContent = question.explanation;
currentQuestionIndex++;
}
function showResults() {
document.getElementById('quizContainer').style.display = 'none';
document.getElementById('result').style.display = 'block';
document.getElementById('finalScore').textContent = 'スコア: ' + score + '/' + quizData.length;
}
document.getElementById('goHome').addEventListener('click', function() {
document.getElementById('result').style.display = 'none';
document.getElementById('home').style.display = 'block';
// クイズの状態をリセット
currentQuestionIndex = 0;
score = 0;
});
style.css
body {
font-family: Arial, sans-serif;
margin: 20px;
}
#quizHeader, #choices, #feedbackContainer, #result {
border: 1px solid #ddd;
padding: 20px;
margin-bottom: 20px;
}
#quizHeader {
background-color: #f9f9f9;
}
#questionNumber {
font-weight: bold;
margin-bottom: 15px;
}
#choices li {
list-style-type: none;
padding: 10px 0;
}
#choices button {
width: 100%;
padding: 10px;
background-color: #f0f0f0;
border: 1px solid #ddd;
cursor: pointer;
transition: background-color 0.3s ease; /* 背景色の変化を滑らかに */
}
#choices button:hover {
background-color: #e9e9e9; /* ホバー時の背景色 */
}
#choices button.activeChoice {
background-color: #007bff; /* アクティブ状態の背景色 */
color: white; /* アクティブ状態のテキスト色 */
border-color: #0056b3; /* アクティブ状態の境界線の色 */
}
#submitAnswer, #nextQuestion, #goHome {
padding: 10px 20px;
font-size: 16px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
display: block;
margin-top: 20px;
}
#submitAnswer:hover, #nextQuestion:hover, #goHome:hover {
background-color: #0056b3; /* ボタンホバー時の背景色 */
}
#feedbackContainer {
background-color: #f9f9f9;
}
#feedback {
font-weight: bold;
}
#explanation, #questionText {
margin-top: 15px;
white-space: pre-wrap; /* 長いテキストを自動的に折り返す */
word-wrap: break-word; /* 必要に応じて単語の途中でも折り返す */
line-height: 2; /* 行間を広げる */
}
quiz-data.js(クイズを格納するところね)
const quizData = [
{
question: "日本の首都はどこですか?",
choices: [
"大阪",
"札幌",
"東京",
"福岡"
],
correctAnswer: "東京",
explanation: "日本の首都は東京です。東京は日本の政治、経済の中心地であり、多くの企業の本社や政府機関が集中しています。"
},
{
question: "次のうち、ビタミンCが豊富に含まれている食品はどれですか?",
choices: [
"レモン",
"牛乳",
"大豆",
"牛肉"
],
correctAnswer: "レモン",
explanation: "レモンはビタミンCが豊富に含まれている食品の一つです。ビタミンCには抗酸化作用があり、免疫力の向上や美肌効果が期待できます。"
},
{
question: "世界で最も長い川はどれですか?",
choices: [
"アマゾン川",
"ナイル川",
"ミシシッピ川",
"長江"
],
correctAnswer: "ナイル川",
explanation: "世界で最も長い川はナイル川です。アフリカ大陸を流れ、地中海に注ぎます。古代エジプト文明の発展に大きく貢献した川としても知られています。"
}
];
このquiz-data.jsのところにクイズを追加していくと4択クイズがいっぱい作れるよ。
ちなみにquiz-data.jsの「question」「explanation」は/nで改行できますよ。
最後に
テストが3日後の3/15に行うことになってるので、結果を報告しますね。
受かったらVBAのスタンダードを受けてみようかな。
あと、テスト試験対策用アプリv2を作ったら、またここで共有するよ。
本当はnote書いている時間もテスト勉強した方がnいい気がするよね。
この記事が気に入ったらサポートをしてみませんか?