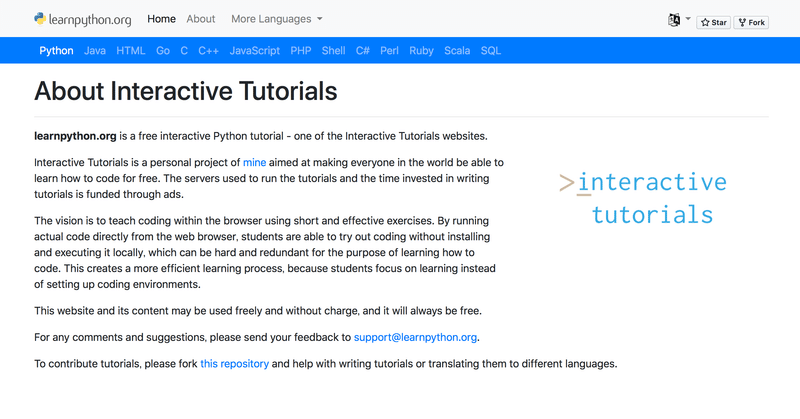
エンジニア採用担当がプログラミング頑張る話【34日目/2ヶ月間】
こんにちは、@hiroki_maekawaです。
2ヶ月プログラミング頑張る企画も残り2週間となりました。
そのため、2週間後に達成したいことを下記したいと思います。
・業務自動化のコード完成
・Dockerを使えるようにする
・プログラミングの文法理解
今日の目標
・AtCoderの問題を1時間解く
・Pythonのチュートリアルを英語で解く
1. AtCoderの問題を1時間解く
今日の問題はこちら。
package main
import (
"fmt"
)
func sum(n int) int {
var left int
for 0 < n {
left += n % 10
n /= 10
}
return left
}
func main() {
var N, A, B int
fmt.Scan(&N, &A, &B)
total := 0
for i := 1; i <= N; i++ {
Nsum := sum(i)
if A <= Nsum && Nsum <= B {
total += i
}
}
fmt.Println(total)
}
これで実行できました。①各行の和をどうやって求めるか、②それをどうループ処理するかという2つのコードが書ければOKです。
この問題を解いたことで、演算子を短く書く方法と、②のループ処理の中に①の結果をどう持ってくるか?という方法を学ぶことができました。
2. Pythonのチュートリアルを英語で解く
これまでGoを日本語で勉強してきましたが、エラー文や公式ドキュメント、また参考にする記事の多くが英語であるため、今回新しい試みでPythonを英語で勉強してみることにしました。
FunctionやArgumentなどのプログラミング用語を英語で覚えてしまおうという作戦です。
今日はLearn the Basicsの下記チュートリアル全てを行いました。
・Hello, World!
・Variables and Types
・Lists
・Basic Operators
・String Formatting
・Basic String Operations
・Conditions
・Loops
・Functions
・Classes and Objects
・Dictionaries
・Modules and Packages
分かりやすいし、全部無料です!
この中でいくつか解いた問題を紹介します。
Exercise1:
Loop through and print out all even numbers from the numbers list in the same order they are received. Don't print any numbers that come after 237 in the sequence.
numbers = [
951, 402, 984, 651, 360, 69, 408, 319, 601, 485, 980, 507, 725, 547, 544,
615, 83, 165, 141, 501, 263, 617, 865, 575, 219, 390, 984, 592, 236, 105, 942, 941,
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958, 609, 842, 451, 688, 753, 854, 685, 93, 857, 440, 380, 126, 721, 328, 753, 470,
743, 527
]
# your code goes here
for number in numbers:
if number == 237:
break
if number % 2 ==1:
continue
print (number)
(読みやすいし書きやすい・・!)
Exercise2:
We have a class defined for vehicles. Create two new vehicles called car1 and car2. Set car1 to be a red convertible worth $60,000.00 with a name of Fer, and car2 to be a blue van named Jump worth $10,000.00.
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
car1 = Vehicle()
car1.name = "Fer"
car1.color = "red"
car1.kind = "convertible"
car1.value = 60000.00
car2 = Vehicle()
car2.name = "Jump"
car2.color = "blue"
car2.kind = "van"
car2.value = 10000.00
# test code
print(car1.description())
print(car2.description())
(PythonのClassがGoでいう構造体なんですね。)
今日できるようになったこと
・短く演算子を記述する
さいごに
はじめプログラミングを勉強し始めた時はGoのチュートリアルの内容が全然分かりませんでしたが(笑)、ある程度Goを理解してきたので今回はPythonのチュートリアルをさくさく進めることができました。
早速明日からは実戦的にPythonを使っていきたいと思います。
それではまた!
この記事が気に入ったらサポートをしてみませんか?