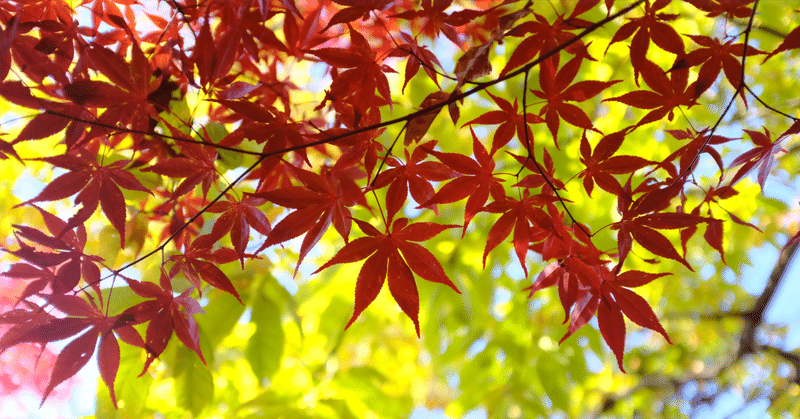
Photo by
masa_iwa10
MT5ボリンジャーバンド逆張りEAソースコード
通貨ペア ゴールド15分足
ロジック
・20BB3σの上下バンドタッチで逆張りエントリー
・ミドルラインタッチで決済
PineScript
//@version=5
strategy("Bollinger Bands Reversal Strategy", overlay=true)
// Bollinger Bands Parameters
length = 20
mult = 3.0
// Calculating the Bollinger Bands
basis = ta.sma(close, length)
dev = mult * ta.stdev(close, length)
upper = basis + dev
lower = basis - dev
// Strategy Entry Conditions
longCondition = close >= upper
if (longCondition)
strategy.entry("SELL", strategy.short)
shortCondition = close <= lower
if (shortCondition)
strategy.entry("BUY", strategy.long)
// Strategy Exit Conditions
exitLongCondition = close >= basis
exitShortCondition = close <= basis
if (exitLongCondition)
strategy.close("BUY")
if (exitShortCondition)
strategy.close("SELL")
// Plotting Bollinger Bands and Middle Line
plot(basis, "Basis", color=color.blue)
p1 = plot(upper, "Upper", color=color.red)
p2 = plot(lower, "Lower", color=color.green)
MT5EA
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
double getBollingerBand(int mode,int shift)
{
//mode == 0 Base Line
//mode == 1 Upper Band
//mode == 2 Lower Band
static int handle = -1;
// ボリンジャーバンド指標のハンドルを取得
if(handle == -1)
{
handle = iBands(_Symbol, PERIOD_CURRENT, 20, 0, 3.0, PRICE_CLOSE);
}
double Band[];
// バッファをコピーしてラインの値を取得
CopyBuffer(handle, mode, shift, 1, Band); // 基本線
return Band[0];
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void OnTick()
{
double upperBand = getBollingerBand(1, 0); // 上部バンド
double lowerBand = getBollingerBand(2, 0); // 下部バンド
double basis = getBollingerBand(0, 0); // 中心バンド
double lastClose = iClose(NULL,PERIOD_CURRENT,0); // 最後の終値
// 買いエントリーの条件
if(lastClose <= lowerBand && position_count(ORDER_TYPE_BUY) == 0)
{
position_entry(ORDER_TYPE_BUY);
}
// 売りエントリーの条件
if(lastClose >= upperBand && position_count(ORDER_TYPE_SELL) == 0)
{
position_entry(ORDER_TYPE_SELL);
}
// 決済条件
if(position_count(ORDER_TYPE_BUY) > 0 && lastClose >= basis)
{
position_all_close(ORDER_TYPE_BUY);
}
if(position_count(ORDER_TYPE_SELL) > 0 && lastClose <= basis)
{
position_all_close(ORDER_TYPE_SELL);
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_all_close(ENUM_ORDER_TYPE side)
{
for(int i=PositionsTotal()-1; i>=0; i--)
{
if("" != PositionGetSymbol(i))
{
if(PositionGetInteger(POSITION_TYPE)==side)
{
if(Symbol()==PositionGetString(POSITION_SYMBOL))
{
if(PositionGetInteger(POSITION_MAGIC)==MagicNumber)
{
position_close(side,PositionGetInteger(POSITION_TICKET));
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_close(ENUM_ORDER_TYPE order_type,ulong position_ticket)
{
MqlTradeRequest req = {};
MqlTradeResult res = {};
order_type = order_type==ORDER_TYPE_BUY?ORDER_TYPE_SELL:ORDER_TYPE_BUY;
req.action = TRADE_ACTION_DEAL; // 成行注文
req.symbol = Symbol();
req.volume = lots; // 注文数量
req.type = order_type; // 売買方向 ORDER_TYPE_BUY or ORDER_TYPE_SELL
req.deviation = slippage; // スリップページ
req.magic = MagicNumber; // マジックナンバー
req.comment = ""; // コメント
req.position = position_ticket; //決済するポジションを指定する
bool order_result = OrderSend(req, res);
if(order_result == false)
{
Print(res.retcode);
}
}
int MagicNumber = 123; // マジックナンバー
double lots = 0.01; // ロットサイズ
int slippage = 10; // スリッページ
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_entry(ENUM_ORDER_TYPE order_type)
{
MqlTradeRequest req = {};
MqlTradeResult res = {};
req.action = TRADE_ACTION_DEAL; // 成行注文
req.symbol = Symbol();
req.volume = lots; // 注文数量
req.type = order_type; // 売買方向 ORDER_TYPE_BUY or ORDER_TYPE_SELL
req.deviation = slippage; // スリップページ
req.magic = MagicNumber; // マジックナンバー
req.comment = ""; // コメント
bool order_result = OrderSend(req, res);
if(order_result == false)
{
Print(res.retcode);
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int order_count()
{
int count =0;
for(int i=OrdersTotal()-1; i>=0; i--)
{
if(OrderSelect(OrderGetTicket(i)))
{
if(Symbol()==OrderGetString(ORDER_SYMBOL))
{
if(OrderGetInteger(ORDER_MAGIC)==MagicNumber)
{
count++;
}
}
}
}
return count ;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int position_count(ENUM_ORDER_TYPE order_type)
{
int count =0;
for(int i=PositionsTotal()-1; i>=0; i--)
{
if("" != PositionGetSymbol(i))
{
if(PositionGetInteger(POSITION_TYPE)==order_type)
{
if(Symbol()==PositionGetString(POSITION_SYMBOL))
{
if(PositionGetInteger(POSITION_MAGIC)==MagicNumber)
{
count++;
}
}
}
}
}
return count ;
}
//+------------------------------------------------------------------+
この記事が気に入ったらサポートをしてみませんか?