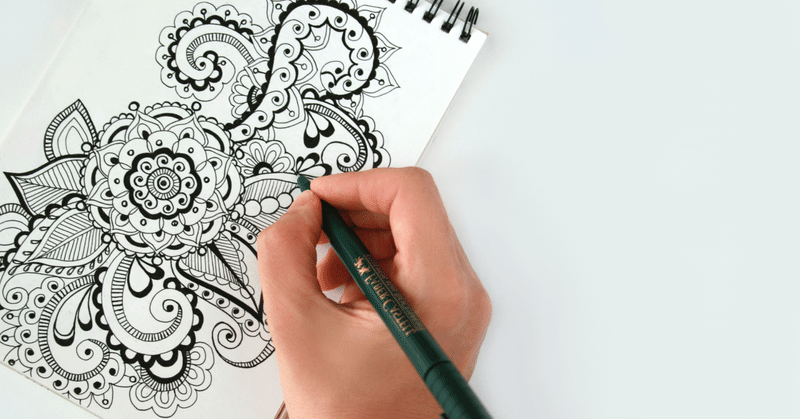
[Python] GoogleドライブのフォルダにGoogleスプレッドシートを新規作成する2つの実装方法
はじめに
Pythonを使用して、Googleドライブの特定のフォルダに新しいGoogleスプレッドシートを作成する方法をまとめます。
Googleスプレッドシートを新規作成する方法として、以下の2つの方法を実装していきます。
gspreadライブラリを使用する方法
PyDriveライブラリを使用する方法
動作環境
・windows11
・Jupyter Notebook 6.4.11(Python 3.9.12)
・Python 3.10.2
手順
1. 事前準備
事前準備として、Google Cloud Platformの設定が必要です。
設定方法を下記にまとめましたので、記事内の手順「1. Google Cloud Platformの設定」をご参照ください。
今回は、新規作成したファイルを配置するフォルダに対して、共有権限の設定を行います。
上記の手順で、Google Cloud Platformの設定で作成したJSON形式の秘密鍵ファイルを使用しますので、テキストエディタ等でファイルを開いておきます。
Googleドライブで、新規作成したファイルを配置するフォルダを開き、フォルダ名の隣の▼をクリックします(画像①)。その後、メニューから、「共有」をクリックします(画像②)
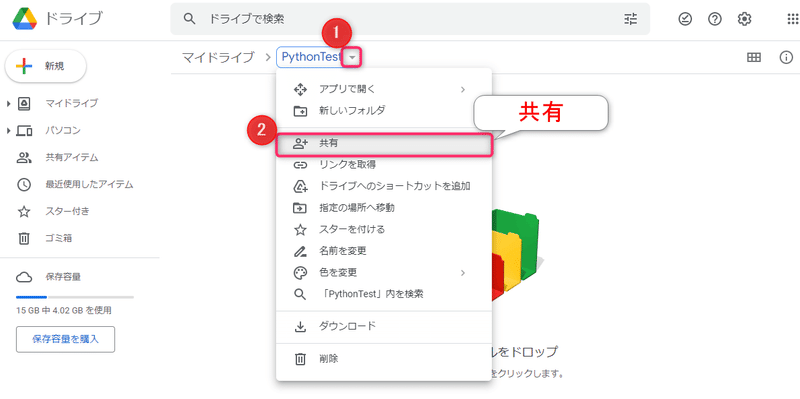
秘密鍵ファイルの中に記載されている「client_email:」の後のメールアドレスをコピーして、下記の赤枠内にペーストします。
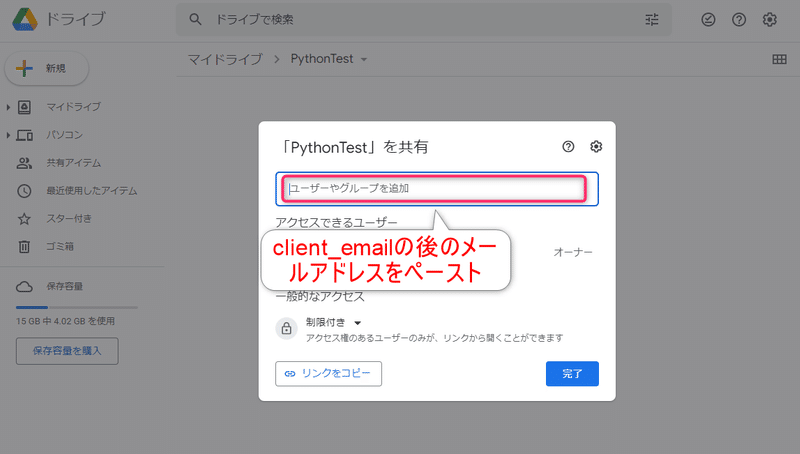
「送信」をクリックします。
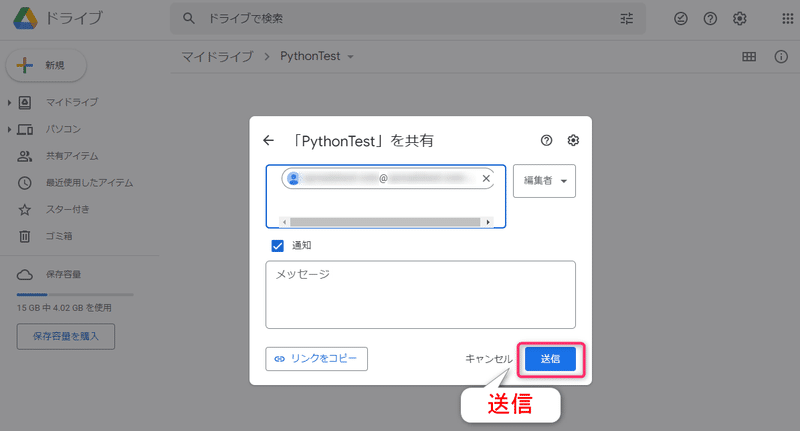
以上で設定完了です!
2. ライブラリをインストールする
今回は、下記のライブラリを使用します。インストールがまだの場合、インストールを行います。
共通して使用するライブラリ
oauth2client
google-api-python-client
google-auth-httplib2
google-auth-oauthlib
gspreadライブラリを使用する場合
gspread
PyDriveライブラリを使用する場合
pydrive
3. Pythonスクリプトを実装する
gspreadライブラリを使用する場合
コード全体は下記です。
from google.oauth2.service_account import Credentials
import gspread
folder_id = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
file_name = 'GC_test_by_gspread'
scopes = [
'<https://www.googleapis.com/auth/spreadsheets>',
'<https://www.googleapis.com/auth/drive>'
]
# Credentials 情報を取得
credentials = ServiceAccountCredentials.from_json_keyfile_name(
'./service_account.json',
scopes=scopes
)
# Auth2のクレデンシャルを使用してGoogleAPIにログイン
gc = gspread.authorize(credentials)
# スプレッドシートの新規作成
sh = gc.create(file_name, folder_id)
順に説明してきます。
①OAuthの認証
scopes = [
'https://www.googleapis.com/auth/spreadsheets',
'https://www.googleapis.com/auth/drive'
]
# Credentials 情報を取得
credentials = ServiceAccountCredentials.from_json_keyfile_name(
'./service_account.json',
scopes=scopes
)
APIで使用する権限を指定します。今回は、Googleスプレッドシートの権限と、Gogoleドライブ上のファイル操作の権限を指定しています。 ServiceAccountCredentials.from_json_keyfile_nameメソッドの第1引数には、Google Cloud Platformで作成した秘密鍵情報が格納されている、JSONファイルのファイルパスを指定します。
②gspreadライブラリを使ってOAuth認証
gc = gspread.authorize(credentials)
gspreadライブラリのauthorizeメソッドを実行すると、OAuth2のクレデンシャルを使用してGoogleAPIにログインし、認証を行います。
戻り値として、生成されたgspreadインスタンスが返されます。
③Googleスプレッドシートを新規作成
# スプレッドシートの新規作成
sh = gc.create('GC_test_by_gspread', folder_id)
Googleスプレッドシートを新規作成するには、②で生成されたgspreadインスタンスのcreateメソッドを実行します。 第1引数に、ファイル名、第2引数に、作成したファイルを配置するフォルダIDを指定します。
PyDriveライブラリを使用する場合
コード全体は下記です。
from google.oauth2.service_account import Credentials
from pydrive.auth import GoogleAuth
from pydrive.drive import GoogleDrive
folder_id = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
file_name = 'GC_test_by_PyDrive'
scopes = [
'<https://www.googleapis.com/auth/spreadsheets>',
'<https://www.googleapis.com/auth/drive>'
]
# Credentials 情報を取得
credentials = ServiceAccountCredentials.from_json_keyfile_name(
'./service_account.json',
scopes=scopes
)
# OAuth認証
gauth = GoogleAuth()
gauth.credentials = credentials
drive = GoogleDrive(gauth)
# スプレッドシートの新規作成
file = drive.CreateFile({
'title': file_name,
'mimeType': 'application/vnd.google-apps.spreadsheet',
"parents": [
{"id": folder_id}
]})
file.Upload()
順に説明してきます。
①OAuthの認証
gspreadライブラリを使用する場合と同様です。
②PyDriveライブラリを使ってOAuth認証
gauth = GoogleAuth()
gauth.credentials = credentials
drive = GoogleDrive(gauth)
OAuth認証を行い、認証情報をもとにGoogleDriveオブジェクトを生成します。
③Googleスプレッドシートを新規作成
# スプレッドシートの新規作成
file = drive.CreateFile({
'title': file_name,
'mimeType': 'application/vnd.google-apps.spreadsheet',
"parents": [
{"id": folder_id}
]})
file.Upload()
CreateFileメソッドで、ファイル名、ファイルの種類、親となるフォルダIDを指定し、Googleスプレッドシートを新規作成します。
作成したファイルを、Uploadメソッドを実行しアップロードします。アップロードを行うことで、Googleドライブ上で参照することが可能となります。
作成したGoogleスプレッドシートを、Googleドライブ上で見てみます。ファイルがそれぞれ作成できているのが確認できました!
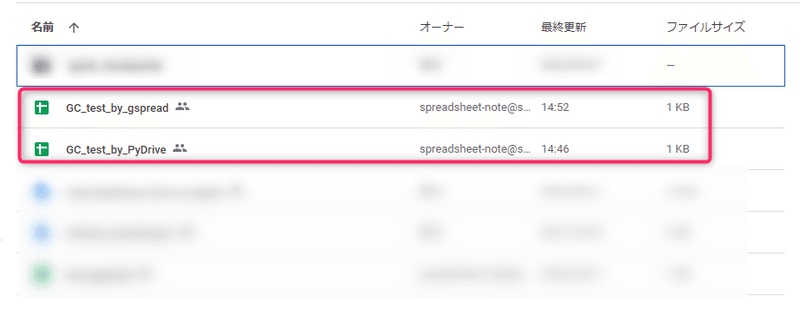
まとめ
今回は、Pythonを使用して、Googleドライブの特定のフォルダにGoogleスプレッドシートを新規作成する2つの実装方法についてまとめました。
Googleスプレッドシートを新規作成した後、書き込みを行う処理が必要な場合が多いと思われます。そのため、gspreadライブラリを使用する方法がスムーズかつ簡単に実装できるかと思います。
参考サイト
この記事が気に入ったらサポートをしてみませんか?