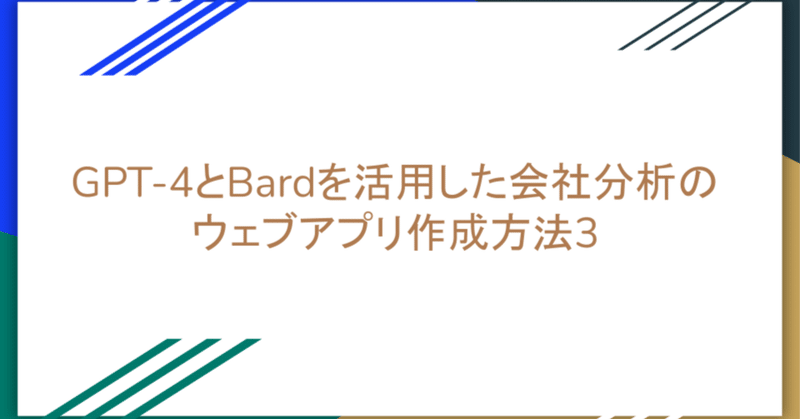
GPT-4とBardを活用した会社分析のウェブアプリ作成方法3
今回作成するウェブアプリの画面となります。
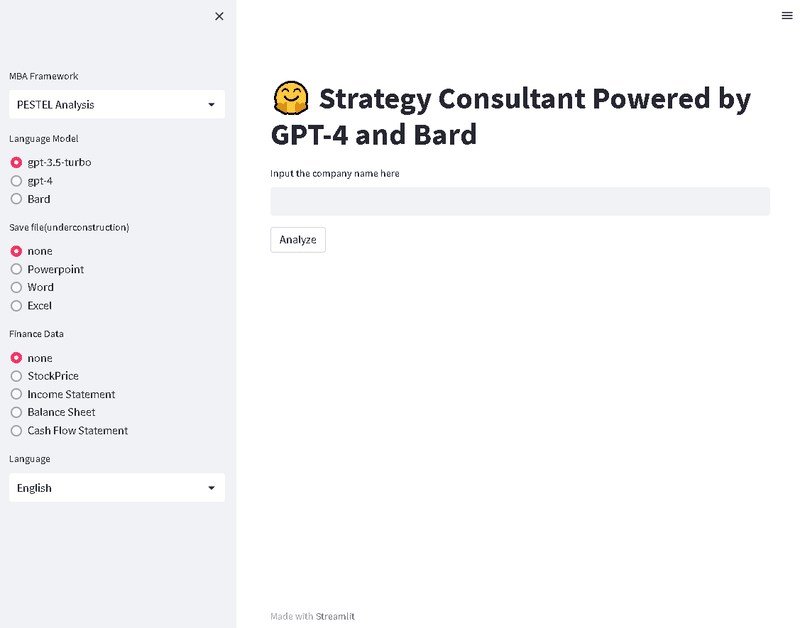
今回のウェブアプリでは、ユーザが会社名を入力し、分析で使いたいフレームワークを指定して、Analyzeをクリックします。
また、その時の分析結果をパワーポイント、ワード、エクセルに保存することができます。
さらに、その会社の財務情報として、株価のグラフ、Income Statement、Balance Sheet、Cash Flow Statementを表示することができるようになっております。
過去にも類似のウェブアプリしており、第3弾目となります。
今回は、事前準備として3つ実施します。
app.py、requirements.txtの準備
OpenAIのAPIキーとグーグルBardのAPIキーの取得
Alpha VantageのAPIキーの取得
1.app.py、requirements.txtの準備
app.pyとして、保存してください。OpenAI、Bard、ALPHA VANTAGEのAPIキーの取得方法については、後続を参考にしてください。
#Import the basic library
import os
import openai
from bardapi import Bard
import streamlit as st
#Import the required libraries for powerpoint
import collections
import collections.abc
from pptx import Presentation
#Import the required libraries for word
import docx
#Import the required libraries for Excel
import openpyxl
#Import the required libraries for StockPrice
import requests
import json
import pandas as pd
import matplotlib.pyplot as plt
#Set the API Key for OpenAI
openai.api_key="sk-xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
#Set the API Key for Bard
os.environ['_BARD_API_KEY']="xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx."
#Set the API Key for ALPHA VANTAGE
api_key = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
#Initialize the presenetaion
prs = Presentation()
title_slide_layout = prs.slide_layouts[1]
slide = prs.slides.add_slide(title_slide_layout)
title = slide.shapes.title
subtitle = slide.placeholders[1]
#prompt
company_info_prompt="""List the Company information including the following:
- Company name
- Industry
- Company size
- Company website
- Company headquarters
- Company founded date
- Company revenue
- Company profit
- Company market value
- Company operating cash flow
- Company balance sheet"""
#Title
st.title('🤗 Strategy Consultant Powered by GPT-4 and Bard')
prompt = st.text_input('Input the company name here')
#select the framework
pagelist_framework = ["PESTEL Analysis","Porter's Five Forces Analysis","SWOT Analysis","Scenario analysis","Ansoff's Growth Matrix",
"BCG Matrix","GE McKinsey Matrix","Resource-Based View analysis","Balanced Scorecard","Core conpetency analysis",
"VRIO Analysis","Blue Ocean Strategy","7S Framework","Lean Startup","Business Model Canvas",
"Customer Journey Map","5C Analysis","4P's (Product, Price, Place, Promotion)","STP (Segmentation, Targeting, Positioning)",
"RACI Matrix (Responsible, Accountable, Consulted, Informed)","OKR (Objectives and Key Results)",
"PDCA Cycle (Plan, Do, Check, Act)"]
sel_framework=st.sidebar.selectbox( "MBA Framework",pagelist_framework)
#select the LLM
sel_llm=st.sidebar.radio("Language Model",["gpt-3.5-turbo","gpt-4","Bard"])
#select whether you save the powepoint or not
sel_file=st.sidebar.radio("Save file(underconstruction)",["none","Powerpoint", "Word","Excel"])
#Select the finance data
sel_finance_data=st.sidebar.radio("Finance Data", ["none","StockPrice","Income Statement", "Balance Sheet", "Cash Flow Statement"])
#Select the language
pagelist_language = ["English","Japanese","Chinese","Spanish"]
sel_language=st.sidebar.selectbox("Language", pagelist_language)
def process_request(prompt, sel_llm, sel_framework, sel_language, operation, sel_file, additional_prompt=''):
st.write(f'{operation}...')
result_area = st.empty()
text = f'{prompt}:'
if sel_llm == "gpt-3.5-turbo" or sel_llm == "gpt-4":
# OpenAI
response = openai.ChatCompletion.create(
model=sel_llm,
messages=[{"role":"user", "content": f"""{operation} {prompt} using {sel_framework}.
{additional_prompt}. Your answer is {sel_language}."""}],
stream=True,
)
for chunk in response:
next = chunk['choices'][0]['delta'].get('content','')
text += next
result_area.write(text)
elif sel_llm=="Bard":
# Google Bard
response = Bard().get_answer(f"""{operation} {prompt} using {sel_framework} in {sel_language}.
{additional_prompt}. Your answer is {sel_language}.""")['content']
st.write( f"{text} {response}")
if sel_file=="Powerpoint":
create_powerpoint(prompt,response)
elif sel_file=="Word":
create_word(prompt,response)
elif sel_file=="Excel":
create_excel(prompt,response)
if sel_finance_data=="StockPrice":
display_stock_price(sel_llm,prompt,api_key)
elif sel_finance_data=="Income Statement":
get_finance_data(prompt, api_key, "INCOME_STATEMENT")
elif sel_finance_data=="Balance Sheet":
get_finance_data(prompt, api_key, "BALANCE_SHEET")
elif sel_finance_data=="Cash Flow Statement":
get_finance_data(prompt, api_key, "CASH_FLOW")
def create_powerpoint(prompt, response):
title.text = f"{prompt} Information"
subtitle.text = f"{response}"
prs.save(prompt + '.pptx')
st.write("Powerpoint is Completed!")
def create_word(prompt, response):
doc = docx.Document()
doc.add_paragraph(f"{prompt} Information")
doc.add_paragraph(f"{response}")
doc.save(prompt + '.docx')
st.write("Word is Completed!")
def create_excel(prompt, response):
wb = openpyxl.Workbook()
ws = wb.active
ws["A1"]=f"{prompt} Information"
ws["A2"]=f"{response}"
wb.save(prompt + '.xlsx')
st.write("Excel is Completed!")
def get_stock_price(symbol, api_key):
base_url = "https://www.alphavantage.co/query"
params = {
"function": "TIME_SERIES_DAILY_ADJUSTED",
"symbol": symbol,
"apikey": api_key
}
response = requests.get(base_url, params=params)
data = response.json()
return data
def display_stock_price(sel_llm, prompt, api_key):
#Get Ticker Symbol
res_ticker_symbol = openai.ChatCompletion.create(
model=sel_llm,
messages=[{"role":"user", "content": f"""Please display the ticker
symbol from {prompt}. Only display the ticker symbol,
nothing else."""}]
)
ticker_symbol=res_ticker_symbol['choices'][0]['message']['content']
st.write(f"{ticker_symbol}")
# Get stock price
data = get_stock_price(ticker_symbol, api_key)
# transform datafram
df = pd.DataFrame(data['Time Series (Daily)']).T
df['4. close'] = pd.to_numeric(df['4. close']) # 終値を数値に変換
# change datatime
df.index = pd.to_datetime(df.index)
# Draw graph
st.title(f'{ticker_symbol} Daily Close Price')
st.line_chart(df['4. close'])
def get_finance_data(ticker_symbol, api_key, statement):
base_url = "https://www.alphavantage.co/query"
params = {
"function": statement,
"symbol": ticker_symbol,
"apikey": api_key
}
response = requests.get(base_url, params=params)
data = response.json()
# Extract the annualReports data to a DataFrame
df = pd.DataFrame(data['annualReports'])
# Display the DataFrame in Streamlit
st.write(statement)
st.write(df)
# Push the button to run the model to analyze the company
if st.button('Analyze'):
process_request(prompt, sel_llm, sel_framework, sel_language, 'Analyzing the company',sel_file, company_info_prompt)
次のコードをrequirements.txtとして保存してください。
streamlit
openai
bardapi
python-pptx
python-docx
openpyxl
matplotlib
2.OpenAIのAPIキーとグーグルBardのAPIキーの取得
OpenAIのAPIキーの取得方法は、各々、下記リンクから取得してください。
BardのAPIキーの取得は、以前書いた記事が参考になります。
3.Alpha VantageのAPIキーの取得
Alpha Vantageとは、金融データを提供するAPIです。ユーザーはこのAPIを利用して、リアルタイムや過去の株価データ、仮想通貨のデータ、外国為替レート、企業の基本情報などを取得することができます。今回は、フリーのAPIキーを利用します。
次に、GET YOUR FREE API KEY TODAYをクリックします。
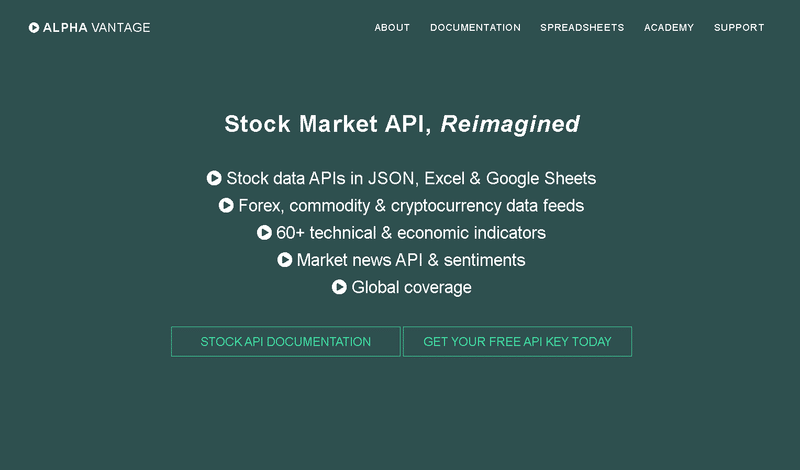
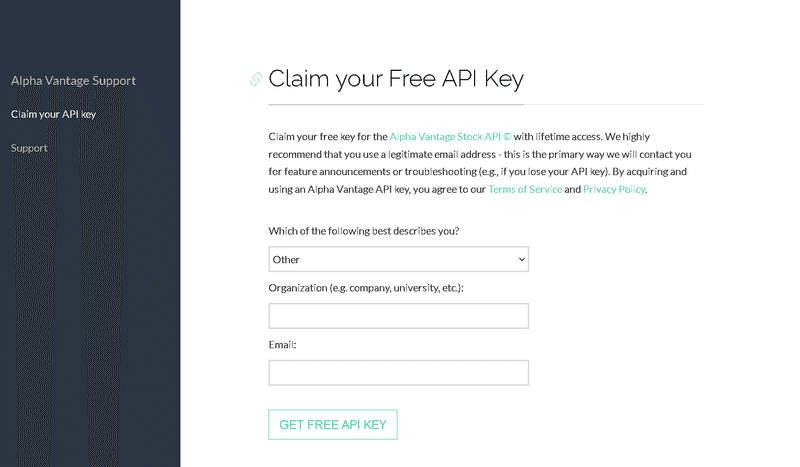
APIキーが表示されたらどこかに保存しておきましょう。
4.Strategic Consultantのウェブアプリの実行
自PCで次を実行します。
python -m venv venv
venv\Scripts\Activate
pip -r requirements.txt
streamlit run app.py
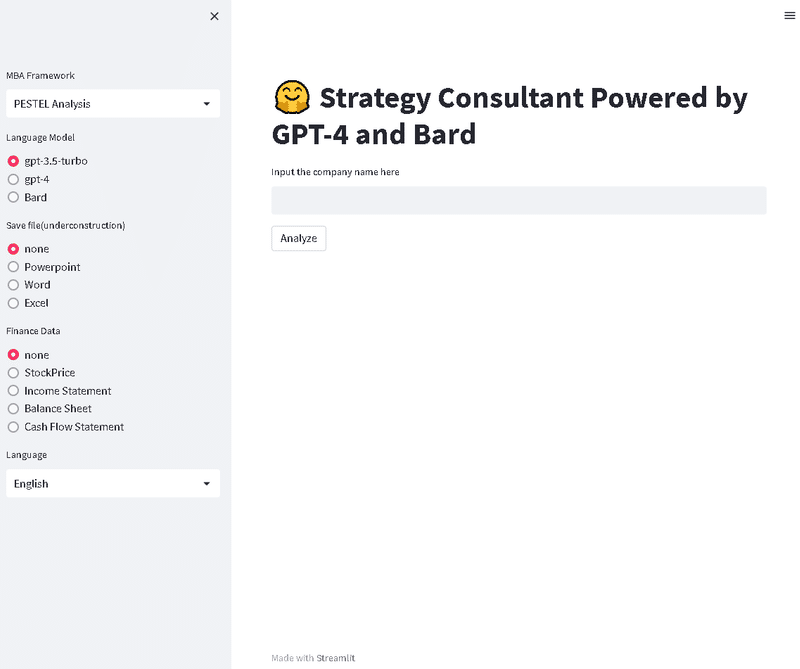
左側で色々と選択して、右側のフォームに会社名を入力してAnalyzeをクリックします。
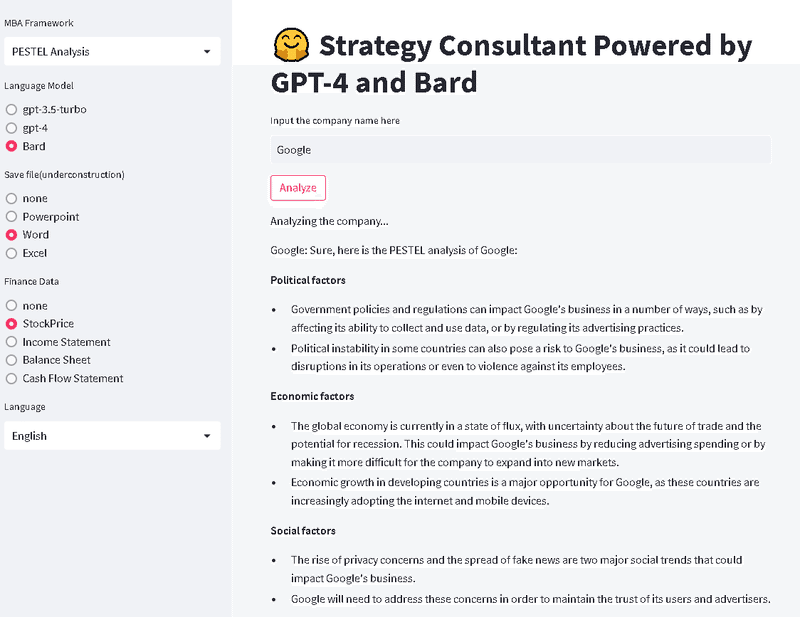
この記事が気に入ったらサポートをしてみませんか?