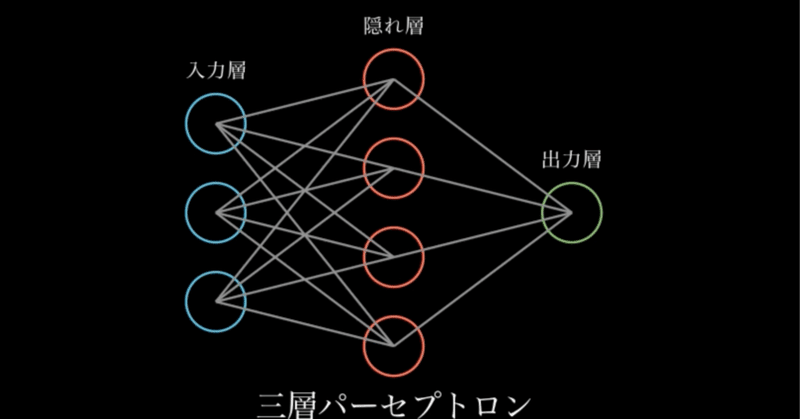
Claude-3でアニメーション書かせてみた!AIアニメ新時代
学校の先生の皆さんに朗報です。
もうアニメーションをパワポで頑張って作る必要はないかもしれません。
まあ別のことを頑張らなければなりませんが。
Claude-3は、Pythonでアニメーションを生成するプログラムを生成できます。
「こんな感じのアニメーションを作るPythonコードを書け」と言えば作ってくれます。
まず百聞は一見にしかず。こちらをご覧あれ
俺も作ってみた。MatPlotlabだけど。
— shi3z (@shi3z) March 10, 2024
ちゃんと細かく指示するとかなりやってくれる。… https://t.co/J0PRfDKx79 pic.twitter.com/HNJWk0k3Jh
これはニューラルネットの基本中の基本である「三層パーセプトロン」の動作をアニメーション化したものです。これは便利。今までKeynoteとかのアニメーション機能を駆使して作っていたのですが、できることとできないことがあり、どうしても妥協するしかありませんでした。
このアニメーションのように、パーセプトロンの上をどのように信号が流れていくかを可視化するのは専用のプログラムを書かなくてはならず、非常に面倒でした。仕事でなければやってらんねえ。
ところが、Claude-3はかなり高度な無茶振りもやってくれるので、コマンドラインのPython環境さえ手元にあれば簡単にアニメーションを作ってくれます。
僕は最初manimと言うツールを知らなかったのでいきなりこんなことを言ってみました。
RS
三層パーセプトロンをアニメーションで解説したい。pythoコードを書いて
すると、Claude-3からこんな返事が返ってきました。
三層パーセプトロンをアニメーションで解説するPythonコードを以下に示します。このコードでは、
`numpy`と`matplotlib`を使用しています。
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# パーセプトロンの設定
input_size = 2
hidden_size = 3
output_size = 1
# ウェイトとバイアスの初期化
hidden_weights = np.random.randn(input_size, hidden_size)
hidden_bias = np.zeros((1, hidden_size))
output_weights = np.random.randn(hidden_size, output_size)
output_bias = np.zeros((1, output_size))
# シグモイド関数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 順伝播
def forward(inputs):
hidden_layer = sigmoid(np.dot(inputs, hidden_weights) + hidden_bias)
output_layer = sigmoid(np.dot(hidden_layer, output_weights) + output_bias)
return hidden_layer, output_layer
# アニメーションの設定
fig, ax = plt.subplots()
ax.set_xlim(-0.1, 1.1)
ax.set_ylim(-0.1, 1.1)
ax.set_title("3-Layer Perceptron")
# ノードの位置
input_pos = np.array([[0, 0], [0, 1]])
hidden_pos = np.array([[0.5, 0], [0.5, 0.5], [0.5, 1]])
output_pos = np.array([[1, 0.5]])
# ノードの描画
input_nodes = ax.scatter(input_pos[:, 0], input_pos[:, 1], s=200, color='lightblue')
hidden_nodes = ax.scatter(hidden_pos[:, 0], hidden_pos[:, 1], s=200, color='lightgreen')
output_nodes = ax.scatter(output_pos[:, 0], output_pos[:, 1], s=200, color='lightyellow')
# 重みの描画
hidden_lines = []
output_lines = []
for i in range(input_size):
for j in range(hidden_size):
line, = ax.plot([input_pos[i, 0], hidden_pos[j, 0]], [input_pos[i, 1], hidden_pos[j, 1]], 'gray', alpha=0.5)
hidden_lines.append(line)
for i in range(hidden_size):
line, = ax.plot([hidden_pos[i, 0], output_pos[0, 0]], [hidden_pos[i, 1], output_pos[0, 1]], 'gray', alpha=0.5)
output_lines.append(line)
# アニメーションの更新関数
def update(frame):
inputs = np.random.rand(1, input_size)
hidden_layer, output_layer = forward(inputs)
input_nodes.set_color(['lightblue' if x < 0.5 else 'blue' for x in inputs[0]])
hidden_nodes.set_color(['lightgreen' if x < 0.5 else 'green' for x in hidden_layer[0]])
output_nodes.set_color(['lightyellow' if x < 0.5 else 'yellow' for x in output_layer[0]])
for i in range(input_size):
for j in range(hidden_size):
hidden_lines[i*hidden_size+j].set_alpha(hidden_weights[i, j] * 0.8 + 0.1)
for i in range(hidden_size):
output_lines[i].set_alpha(output_weights[i, 0] * 0.8 + 0.1)
return input_nodes, hidden_nodes, output_nodes, *hidden_lines, *output_lines
# アニメーションの作成
ani = FuncAnimation(fig, update, frames=100, interval=500, blit=True)
plt.show()
```
このコードでは、以下の手順でアニメーションを作成しています。
1. 3層パーセプトロンの設定(入力層、隠れ層、出力層のサイズ)を定義します。
2. ウェイトとバイアスをランダムに初期化します。
3. シグモイド関数と順伝播の関数を定義します。
4. アニメーションの設定(図の範囲、タイトル)を行います。
5. ノードの位置を定義し、散布図で描画します。
6. 重みを表す線を描画します。
7. アニメーションの更新関数を定義します。この関数では、ランダムな入力に対する順伝播を計算し、ノードの色と重みの透明度を更新します。
8. `FuncAnimation`を使用してアニメーションを作成します。
アニメーションが表示され、ランダムな入力に対するパーセプトロンの動作を可視化できます。ノードの色は入力値に応じて変化し、重みの太さは重みの値に応じて変化します。
このコードを実行すると、3層パーセプトロンの動作を理解するための視覚的な補助となるアニメーションが表示されます。
このコードの結果表示された画面はアニメーションしませんでした。
そこでこんな指示を加えました。
アニメーションしていない。左側から入力された二つの信号が、重みの影響を受けて線上を拡大縮小しながら移動し、最終的に右の出力へ向かうアニメーションを作れ
するとこんな答えが返ってきました
了解です。入力信号が重みの影響を受けて線上を移動し、
最終的に出力に到達するアニメーションを作成してみましょう。
以下のコードでは、信号を表す円をアニメーション化し、重みに応じて線の太さを変化させています。
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# パーセプトロンの設定
input_size = 2
hidden_size = 3
output_size = 1
# ウェイトとバイアスの初期化
hidden_weights = np.random.randn(input_size, hidden_size)
hidden_bias = np.zeros((1, hidden_size))
output_weights = np.random.randn(hidden_size, output_size)
output_bias = np.zeros((1, output_size))
# シグモイド関数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 順伝播
def forward(inputs):
hidden_layer = sigmoid(np.dot(inputs, hidden_weights) + hidden_bias)
output_layer = sigmoid(np.dot(hidden_layer, output_weights) + output_bias)
return hidden_layer, output_layer
# アニメーションの設定
fig, ax = plt.subplots()
ax.set_xlim(-0.1, 1.1)
ax.set_ylim(-0.1, 1.1)
ax.set_title("3-Layer Perceptron")
# ノードの位置
input_pos = np.array([[0, 0], [0, 1]])
hidden_pos = np.array([[0.5, 0], [0.5, 0.5], [0.5, 1]])
output_pos = np.array([[1, 0.5]])
# ノードの描画
input_nodes = ax.scatter(input_pos[:, 0], input_pos[:, 1], s=200, color='lightblue')
hidden_nodes = ax.scatter(hidden_pos[:, 0], hidden_pos[:, 1], s=200, color='lightgreen')
output_nodes = ax.scatter(output_pos[:, 0], output_pos[:, 1], s=200, color='lightyellow')
# 重みの描画
hidden_lines = []
output_lines = []
for i in range(input_size):
for j in range(hidden_size):
line, = ax.plot([input_pos[i, 0], hidden_pos[j, 0]], [input_pos[i, 1], hidden_pos[j, 1]], 'gray', alpha=0.5)
hidden_lines.append(line)
for i in range(hidden_size):
line, = ax.plot([hidden_pos[i, 0], output_pos[0, 0]], [hidden_pos[i, 1], output_pos[0, 1]], 'gray', alpha=0.5)
output_lines.append(line)
# 信号の描画
signal_pos = np.zeros((input_size, 2))
signal_scatter = ax.scatter(signal_pos[:, 0], signal_pos[:, 1], s=100, color='red')
# アニメーションの更新関数
def update(frame):
t = frame / 100
# 信号の位置を更新
signal_pos[:, 0] = t
signal_pos[:, 1] = input_pos[:, 1]
signal_scatter.set_offsets(signal_pos)
# 重みの更新
for i in range(input_size):
for j in range(hidden_size):
alpha = (hidden_weights[i, j] - hidden_weights.min()) / (hidden_weights.max() - hidden_weights.min())
hidden_lines[i*hidden_size+j].set_alpha(alpha * 0.8 + 0.1)
hidden_lines[i*hidden_size+j].set_linewidth(alpha * 3 + 1)
for i in range(hidden_size):
alpha = (output_weights[i, 0] - output_weights.min()) / (output_weights.max() - output_weights.min())
output_lines[i].set_alpha(alpha * 0.8 + 0.1)
output_lines[i].set_linewidth(alpha * 3 + 1)
return signal_scatter, *hidden_lines, *output_lines
# アニメーションの作成
ani = FuncAnimation(fig, update, frames=100, interval=50, blit=True)
plt.show()
```
このコードでは、以下の変更を加えました。
1. 信号の位置を表す`signal_pos`配列を作成し、`signal_scatter`で描画しています。
2. `update`関数内で、`frame`引数を使用して信号の位置を更新しています。
`t = frame / 100`は、0から1までの値を取るパラメータです。信号の位置は、
`signal_pos[:, 0] = t`で更新されます。
3. 重みの更新部分で、`set_linewidth`メソッドを使用して線の太さを変更しています。
重みが大きいほど、線が太くなります。
4. `FuncAnimation`を使用して、`update`関数を各フレームで呼び出しています。
`frames=100`は、100フレームのアニメーションを作成することを意味します。
このコードを実行すると、信号が左から右へ移動し、
重みの影響を受けて線の太さが変化するアニメーションが表示されます。
信号は入力ノードから始まり、隠れ層を経由して出力ノードに到達します。
アニメーションがうまく表示されない場合や、他に質問があれば、お知らせください。
するとこんな画面が出てきました。
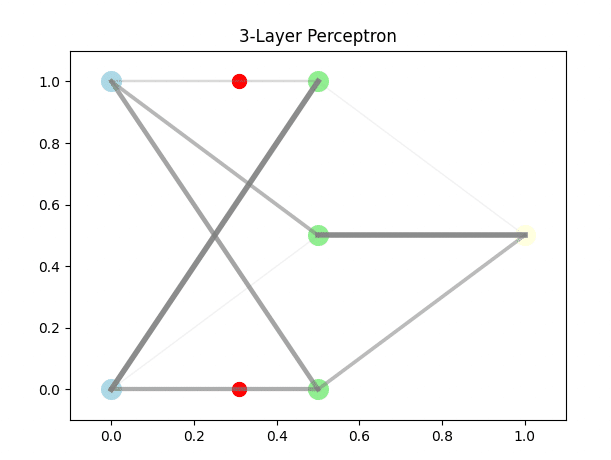
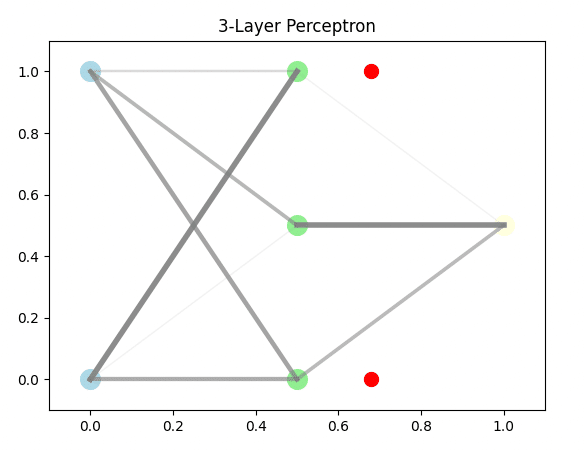
そこでさらにこういうメッセージを送りました。結果を毎回表示すると長くなりすぎるのでプロンプトだけ貼り付けます
惜しい。二層目から出力に向かう信号が平行に移動してしまってる。出力に直接向かうようにすべき
ちょっとマシになったんだけどイマイチでした
惜しい。一層目から二層目まで行く信号もそれぞれ中間層に向けて3つに分岐すべきである
分岐しませんでした
一層目から二層目の各ニューロンへ信号が分配されていない。やり直し
分岐するようになりました。だいぶ完成系に近づいています
信号の大きさがノードの重みによって徐々に拡大縮小するようになってない。やり直し
まだだめでした
File "/Users/shi3z/miniconda3/lib/python3.10/site-packages/matplotlib/path.py", line 642, in get_extents
xys = np.concatenate(xys)
File "<__array_function__ internals>", line 180, in concatenate
ValueError: need at least one array to concatenate
このエラーが出た。第二層から第三層への信号は合体されるため、
信号の数は同一ではなくなることに注意されたし。
各ノードでは入力層から隠れ層のノードに届いた信号の合計値が隠れ層から出力層への信号の大きさとなる
これでもだめです。
細かく指示を出します。
エラーを自分で訂正できないインターンへ指示出すのとすごく似てます
ons.py", line 391, in draw
extents = paths[0].get_extents(combined_transform)
File "/Users/shi3z/miniconda3/lib/python3.10/site-packages/matplotlib/path.py", line 642, in get_extents
xys = np.concatenate(xys)
File "<__array_function__ internals>", line 180, in concatenate
ValueError: need at least one array to concatenate
またエラーが出たし、第二層から第三層に余計な信号が行くようになっている。
第一層から第二層への信号は第二層到達時点で表示をやめ、
新たに第二層から第三層に向かう信号を描画するようにすること
これでさっきPostを貼り付けたようなアニメーションができました。
元ツイートのようにmanimでも試してみたいと思い、こんなプロンプトを描きました
これをmanimにしろ
何回か苦戦して、エラーコードをもらったらひたすらClaude-3に戻すと言うことを繰り返して何回目かにやっと静止画っぽいものが出てきました。
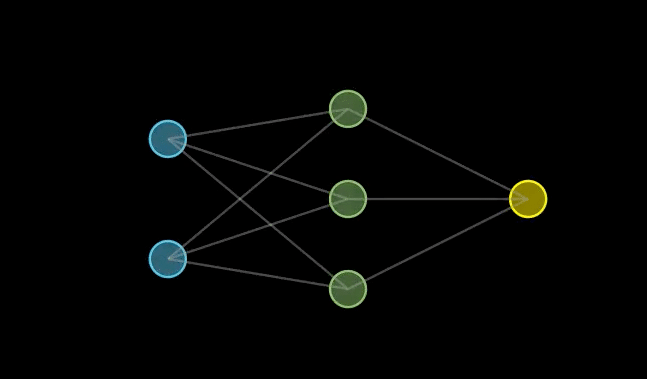
しかしなかなか動画が出てきません。この辺り、manimはちょっとマニアックでclaude-2があまり知識を持ってないのかもしれません。
苦戦しすぎたので仕切り直しで新しいチャットを立てます。
三層パーセプトロンをアニメーションで解説したい。pythoコードを書いて。manimを使う
すると一発でこれが出てきました。
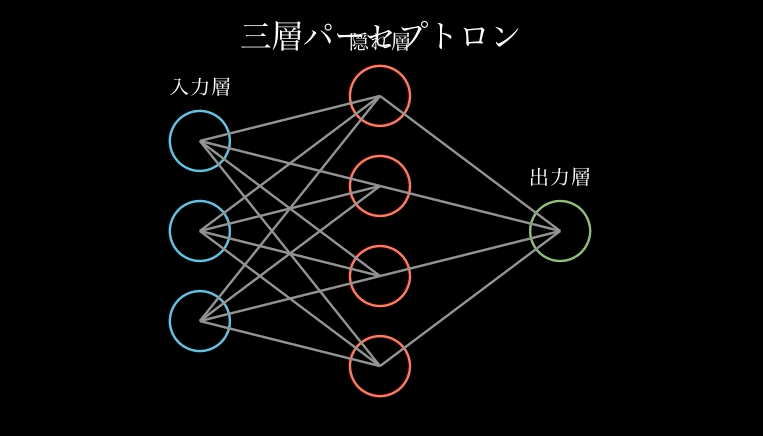
すげえ!
Matplotlibのやりとりがかえって邪魔をしていたようです。
アニメーションが地味なのと三層パーセプトロンというタイトルが隠れそうと被っているのでそれを修正してもらいます。
三層パーセプトロンという文字が隠れ層とかぶっているので離してほしい。
また、入力層の各ニューロンがランダムに光った後、それぞれのノードを伝って信号が隠れ層に行き、
隠れ層のノードが再びランダムに光り、出力層に行くようなアニメーションを作れ
するといきなりすげーアニメが表示されました。
僕が想像もしてなかった動きです。
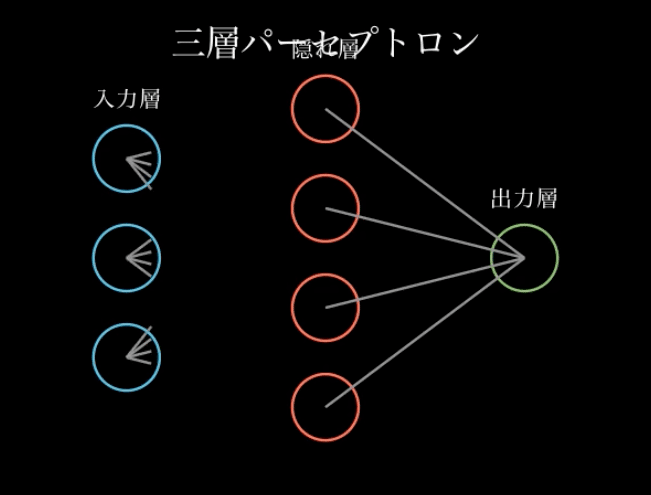
文字が被ってるのは理解できなかったみたいなのでここまできたら手を加えようかと思います。manim知らないけど
こんな感じの仕上がりになりました。所要時間は、Claude-3のチャット立ち上げ直してから5分
https://twitter.com/shi3z/status/1766765085488472306
Claude-3によるアニメ自動生成
— shi3z (@shi3z) March 10, 2024
manim使うようにしたら想像を絶する結果になって絶句してる。これ嬉しいけど仕事減る人確実に出る(でもこれ本来やりたくない仕事の部類だからもういいのかな)
これ昔MAXHEADROOMでブライスリンチがやってたデモとほぼ同じじゃんよ#AIアニメ… pic.twitter.com/gCs3N21xy9
ソースはこちら
from manim import *
import random
class ThreeLayerPerceptron(Scene):
def construct(self):
# ニューロンを表す円を作成
input_neurons = [Circle(radius=0.5, color=BLUE) for _ in range(3)]
hidden_neurons = [Circle(radius=0.5, color=RED) for _ in range(4)]
output_neuron = Circle(radius=0.5, color=GREEN)
# ニューロンを配置
input_layer = VGroup(*input_neurons).arrange(DOWN, buff=0.5)
hidden_layer = VGroup(*hidden_neurons).arrange(DOWN, buff=0.5)
output_layer = VGroup(output_neuron)
layers = VGroup(input_layer, hidden_layer, output_layer).arrange(RIGHT, buff=2)
# 層間の接続を表す線を作成
connections = []
for input_neuron in input_neurons:
for hidden_neuron in hidden_neurons:
connections.append(Line(input_neuron.get_center(), hidden_neuron.get_center(), color=GRAY))
for hidden_neuron in hidden_neurons:
connections.append(Line(hidden_neuron.get_center(), output_neuron.get_center(), color=GRAY))
# アニメーションを構築
self.play(FadeIn(layers))
self.wait(1)
for connection in connections:
self.play(Create(connection), run_time=0.1)
self.wait(1)
# 解説テキストを表示
title = Text("三層パーセプトロン").scale(0.8).to_edge(DOWN)
input_text = Text("入力層", font_size=24).next_to(input_layer, UP)
hidden_text = Text("隠れ層", font_size=24).next_to(hidden_layer, UP)
output_text = Text("出力層", font_size=24).next_to(output_layer, UP)
self.play(Write(title))
self.wait(1)
self.play(FadeIn(input_text), FadeIn(hidden_text), FadeIn(output_text))
self.wait(1)
# 信号の伝達をアニメーション
for _ in range(10):
for _ in range(2):
# 入力層のニューロンをランダムに光らせる
input_animations = []
for neuron in input_neurons:
input_animations.append(Flash(neuron, color=YELLOW, line_length=0.3, num_lines=8, flash_radius=0.5, time_width=0.3, run_time=0.5))
self.play(*input_animations)
# 信号を隠れ層に伝達
hidden_animations = []
for connection in connections[:len(input_neurons) * len(hidden_neurons)]:
hidden_animations.append(ShowPassingFlash(connection, time_width=0.5, run_time=0.5))
self.play(*hidden_animations)
# 隠れ層のニューロンをランダムに光らせる
hidden_flash_animations = []
for neuron in hidden_neurons:
hidden_flash_animations.append(Flash(neuron, color=YELLOW, line_length=0.3, num_lines=8, flash_radius=0.5, time_width=0.3, run_time=0.5))
self.play(*hidden_flash_animations)
# 信号を出力層に伝達
output_animations = []
for connection in connections[len(input_neurons) * len(hidden_neurons):]:
output_animations.append(ShowPassingFlash(connection, time_width=0.5, run_time=0.5))
self.play(*output_animations)
# 出力層のニューロンを光らせる
self.play(Flash(output_neuron, color=YELLOW, line_length=0.3, num_lines=8, flash_radius=0.5, time_width=0.3, run_time=0.5))