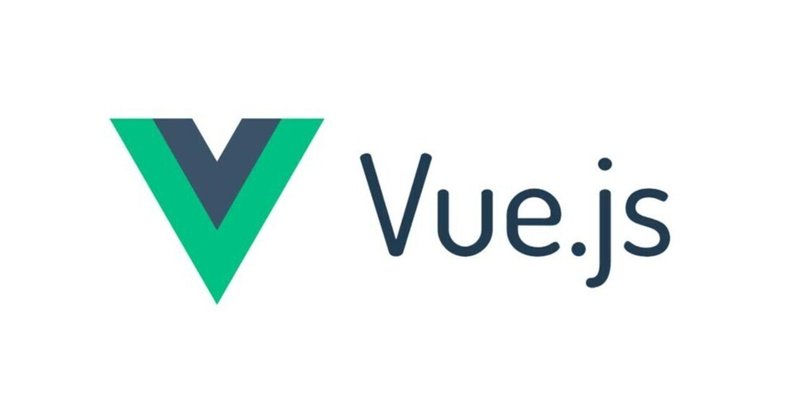
Vue.jsでCSSアニメーションのtransitionを使用する場合
Vue.jsでアニメーションを使用する際、以下3つの方法がある事を学んだので記録していく。
transitionで囲まれた要素に対し、以下デフォルトClass名を適用させる
.v-enter-from
.v-enter-to
.v-enter-active
.v-leave-from
.v-leave-to
.v-leave-active
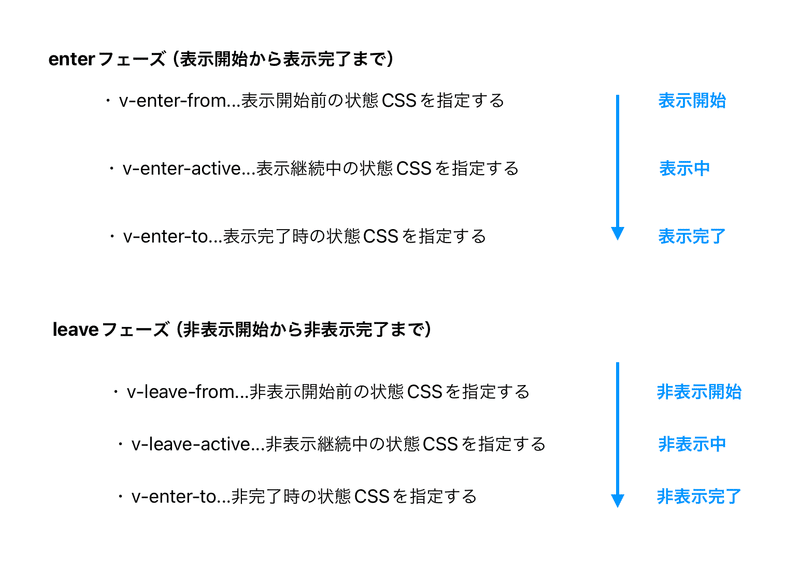
<!doctype html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>RadioButton2</title>
</head>
<body>
<div id="app">
<button @click="show = !show">表示の切り替え</button>
<transition>
<p v-if="show">
こんにちは<br />
私はVue.jsの勉強中です。<br />
</p>
</transition>
</div>
</body>
</html>
<script src="https://unpkg.com/vue@3.2.31/dist/vue.global.js"></script>
<script>
const app = Vue.createApp({
data() {
return {
show: true
}
}
})
const vm = app.mount('#app')
</script>
<style>
.v-enter-from,
.v-leave-to {
opacity: 0;
}
.v-enter-to,
.v-leave-from {
opacity: 1;
}
.v-enter-active,
.v-leave-active {
transition: opacity 0.5s;
}
</style>
上記コードはボタンがクリックされる度、0.5秒をかけてフェードイン/フェードアウトが発生。
transitionにname属性を指定し、デフォルトCSS名の先頭を任意の名称にする場合
<!doctype html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>RadioButton2</title>
</head>
<body>
<div id="app">
<button @click="show = !show">表示の切り替え</button>
<transition name="fade">
<p v-if="show">
こんばんは<br />
fadeに名称をかえました。<br />
</p>
</transition>
</div>
</body>
</html>
<script src="https://unpkg.com/vue@3.2.31/dist/vue.global.js"></script>
<script>
const app = Vue.createApp({
data() {
return {
show: true
}
}
})
const vm = app.mount('#app')
</script>
<style>
.fade-enter-from,
.fade-leave-to {
opacity: 0;
}
.fade-enter-to,
.fade-leave-from {
opacity: 1;
}
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s;
}
</style>
外部CSSライブラリに適用させる場合
.enter-from-class
.enter-active-class
.enter-to-class
.leave-from-class
.leave-active-class
.leave-to-class
<!doctype html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Transition3</title>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css"
/>
</head>
<body>
<div id="app">
<button @click="show = !show">表示の切り替え</button>
<transition
name="zoom"
enter-active-class="animate__heartBeat"
leave-active-class="animate__heartBeat"
>
<p class="text" v-if="show">
Hello<br />
animasitionがつきました。<br />
</p>
</transition>
</div>
</body>
</html>
<script src="https://unpkg.com/vue@3.2.31/dist/vue.global.js"></script>
<script>
const app = Vue.createApp({
data() {
return {
show: true
}
}
})
const vm = app.mount('#app')
</script>
<style>
.text {
text-align: center;
}
</style>
この記事が気に入ったらサポートをしてみませんか?