p5.FFTのリファレンスを見てみた
p5.sound.jsのFFT解析が少しパワーアップしていたっぽいので、
今日はリファレンスを上から読んでいこうかなと思います。
途中自分の感想やメモ書き的なものが含まれていますが、
自分用のメモののつもりではあるので、ご承知ください。
FFT (Fast Fourier Transform) is an analysis algorithm that isolates individual audio frequencies within a waveform.
FFT(高速フーリエ変換)波形から個別の音声周波数を解析できる手法です。
ここはいいですね。FFTがそもそもわからんって方は、こちらの方の解説がわかりやすいです。有識者に怒られるかもですが、つまりは、音に含まれる成分を解析できる手法で、低い音が多いとか、高い音が多いとか、波形の成分を解析できる手法だと思っていれば問題ないかなと思います。
computes amplitude values along the time domain. The array indices correspond to samples across a brief moment in time. Each value represents amplitude of the waveform at that sample of time.
この辺はFFTの話でした。ので飛ばします。
Once instantiated, a p5.FFT object can return an array based on two types of analyses:
• FFT.waveform() computes amplitude values along the time domain. The array indices correspond to samples across a brief moment in time. Each value represents amplitude of the waveform at that sample of time.
• FFT.analyze() computes amplitude values along the frequency domain. The array indices correspond to frequencies (i.e. pitches), from the lowest to the highest that humans can hear. Each value represents amplitude at that slice of the frequency spectrum. Use with getEnergy() to measure amplitude at specific frequencies, or within a range of frequencies.
p5.FFTオブジェクトには、waveform() とanalyze()の2通りあるよんとのこと。
FFT.waveform()はサンプル時間あたりの振幅を返してくれる。
FFT.analyze()は、サンプル時間あたりの周波数を返してくれる。人間の可聴域の周波数を返すとあるので、低い音で20Hz、高い音で20kHzくらいまでの範囲だと思います。
さらにgetEnergy()を使うと、特定の周波数帯を取り出せるようになっているそうです。
FFT analyzes a very short snapshot of sound called a sample buffer. It returns an array of amplitude measurements, referred to as bins. The array is 1024 bins long by default. You can change the bin array length, but it must be a power of 2 between 16 and 1024 in order for the FFT algorithm to function correctly. The actual size of the FFT buffer is twice the number of bins, so given a standard sample rate, the buffer is 2048/44100 seconds long.
周波数分解能(FFTbins)の値は、1024がデフォルトとなっているそうです。
FFTバッファーサイズは、その二倍のbinsの値なので、サンプリングレートが44100Hzだったら、2048/44100秒間ってことになるとのこと。
この辺がわかりやすかった。参考資料。
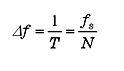
つまりは、FFTbinsの値とバッファーサイズの関係性がわかりました。
This function requires you include the p5.sound library. Add the following into the head of your index.html file:
<script src="path/to/p5.sound.js"></script>
これらの関数を使うには、p5.soundライブラリーが必要なので、index.htmlファイルの中に<script src="path/to/p5.sound.js"></script>みたいな感じで読み込んで使おうとのこと。
パラメータの使い方
new p5.FFT([smoothing], [bins])
Parameterssmoothing
Number:
Smooth results of Freq Spectrum. 0.0 < smoothing < 1.0. Defaults to 0.8.
(Optional)
bins
Number:
Length of resulting array. Must be a power of two between 16 and 1024. Defaults to 1024.
(Optional)
new p5.FFT([smoothing],[bins])とできる。
例えば、こんな感じで使用可能。
fft = new p5.FFT(0.9, 1024);
Smoothingの値は、デフォルトが0.8で、0から1の間で設定可能。
binsは1024がデフォルト。
setInput()
Set the input source for the FFT analysis. If no source is provided, FFT will analyze all sound in the sketch.
音声入力を設定できる。引数にsetInput(song); のような形で設定可能。
waveform()
Returns an array of amplitude values (between -1.0 and +1.0) that represent a snapshot of amplitude readings in a single buffer. Length will be equal to bins (defaults to 1024). Can be used to draw the waveform of a sound.
-1 から1の範囲で1つのバッファーあたりの振幅の配列を返す。配列の長さはbinsの値と同じ1024がデフォルト。
analyze()
Returns an array of amplitude values (between 0 and 255) across the frequency spectrum. Length is equal to FFT bins (1024 by default). The array indices correspond to frequencies (i.e. pitches), from the lowest to the highest that humans can hear. Each value represents amplitude at that slice of the frequency spectrum. Must be called prior to using getEnergy().
0-255の範囲で周波数あたりの振幅の配列を返す。配列のインデックスは周波数(ピッチ音高)ごとの音量になっている。
getEnergy()
Returns the amount of energy (volume) at a specific frequency, or the average amount of energy between two frequencies. Accepts Number(s) corresponding to frequency (in Hz), or a "string" corresponding to predefined frequency ranges ("bass", "lowMid", "mid", "highMid", "treble"). Returns a range between 0 (no energy/volume at that frequency) and 255 (maximum energy). NOTE: analyze() must be called prior to getEnergy(). analyze() tells the FFT to analyze frequency data, and getEnergy() uses the results to determine the value at a specific frequency or range of frequencies.
特定の周波数のエネルギー(音量)を返すか、2つの周波数範囲の平均値を返す。
また、("bass", "lowMid", "mid", "highMid", "treble")っとストリング型で指定することができ、0から255の値を返す。
getCentroid()
Returns the spectral centroid of the input signal. NOTE: analyze() must be called prior to getCentroid(). Analyze() tells the FFT to analyze frequency data, and getCentroid() uses the results determine the spectral centroid.
周波数のセントロイドを返す。セントロイドは全体の周波数成分に含まれる音量の重心という意味。
smooth()
Smooth FFT analysis by averaging with the last analysis frame.
最後の解析するフレームの平均をとって、スムーズにFFT解析する関数らしい??ようはスムージングですね。
linAverages()
Returns an array of average amplitude values for a given number of frequency bands split equally. N defaults to 16. NOTE: analyze() must be called prior to linAverages(). Analyze() tells the FFT to analyze frequency data, and linAverages() uses the results to group them into a smaller set of averages.
周波数バンドの振幅の平均値の配列を返す。
logAverages()
Returns an array of average amplitude values of the spectrum, for a given set of Octave Bands NOTE: analyze() must be called prior to logAverages(). Analyze() tells the FFT to analyze frequency data, and logAverages() uses the results to group them into a smaller set of averages.
スペクトラムの振幅の平均値の配列を返す。オクターブバンドを設定できる。オクターブバンドは、ある周波数を中心にして上限と下限の周波数比が 1 オクターブとなる周波数の帯域(バンド)のこと。
使用例としては、
オクターブバンドってなんだっけ?は、この辺の記事がわかりやすかったです。
https://www.onosokki.co.jp/HP-WK/c_support/newreport/noise/souon_11.htm
getOctaveBands()
Calculates and Returns the 1/N Octave Bands N defaults to 3 and minimum central frequency to 15.625Hz. (1/3 Octave Bands ~= 31 Frequency Bands) Setting fCtr0 to a central value of a higher octave will ignore the lower bands and produce less frequency groups.
オクターブバンドの1/Nの値を返す。デフォルトのNの値は3です。最小中心周波数は 15.625Hz です。(1/3 Octave Bands ~= 31 Frequency Bands) fCtr0をより高いオクターブの中心値に設定すると、より低いバンドは無視され、より少ない周波数グループが生成される。
Notice any errors or typos? Please let us know. Please feel free to edit lib/addons/p5.sound.js and issue a pull request!
この記事が気に入ったらサポートをしてみませんか?