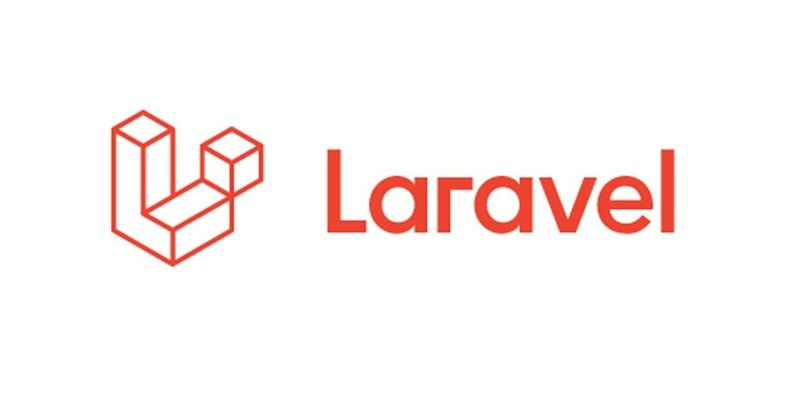
Laravel でCron設定
スタッフ向けにLaravelでの開発環境の構築手順&メモ書き
cronっていうのは、定期的に処理したい時のコマンドって感じ
毎週ランキングを集計したいとか、デイリーで何か処理したいとか、予め決められたスケジュールで動作する処理を設定するコマンド的なやつ
とりあえずは、サーバにすでにcronがインストールされているか確認
/etc/rc.d/init.d/crond status
cronのインストール
yum install -y crontabs vixie-cron
cronの起動
/etc/rc.d/init.d/crond start
サービスの起動設定
chkconfig --add crond
これで、cronは動いている状態になってるはず!
ここからがプログラムの作業になる
①コマンドを作って処理を書く
②スケジュールを作る
③cronにエントリーを追加
ざっくり上記の3工程
①コマンドを作って処理を書く
php artisan make:command CronCommand
*CronCommandのところは任意
上記コマンドを打つとCronCommand.phpが生成されているはず
生成される場所は以下
app/Console/Commands/CronCommand.php
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class CronCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
//修正箇所1:コマンド名を適宜変更
protected $signature = 'command:rankingcommand';
/**
* The console command description.
*
* @var string
*/
//修正箇所2:コマンドの説明文
protected $description = 'rankingcommand 週刊ランキング';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
//修正箇所3:処理したいプログラム
logger('rankingcommandのテスト中');
//ここが本当のメイン
}
}
3箇所を修正する
②スケジュールを作る
app/Console/Kernel.php
<?php
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
/**
* The Artisan commands provided by your application.
*
* @var array
*/
protected $commands = [
//修正箇所1:コマンドの登録
\App\Console\Commands\CronCommand::class,
];
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
// $schedule->command('inspire')
// ->hourly();
//修正箇所2:スケジュールの登録
$schedule->command('command:rankingcommand')->everyMinute();
}
/**
* Register the commands for the application.
*
* @return void
*/
protected function commands()
{
$this->load(__DIR__.'/Commands');
require base_path('routes/console.php');
}
}
2箇所を修正する
*修正箇所2については、CronCommand.phpの修正箇所1で決めたコマンド名を書く
*->everyMinute(); の部分でスケジュール間隔を決めている
->everyMinute(); 毎分
->everyFiveMinutes(); 5分ごと
->everyTenMinutes(); 10分ごと
->everyFifteenMinutes(); 15分ごと
->everyThirtyMinutes(); 30分ごと
->hourly(); 毎時
->daily(); 毎日
->weekly(); 毎週
->monthly(); 毎月
③cronにエントリーを追加
$ crontab -e
* * * * * php /{path_to_project}/artisan schedule:run >> /dev/null 2>&1
終わり
この記事が気に入ったらサポートをしてみませんか?