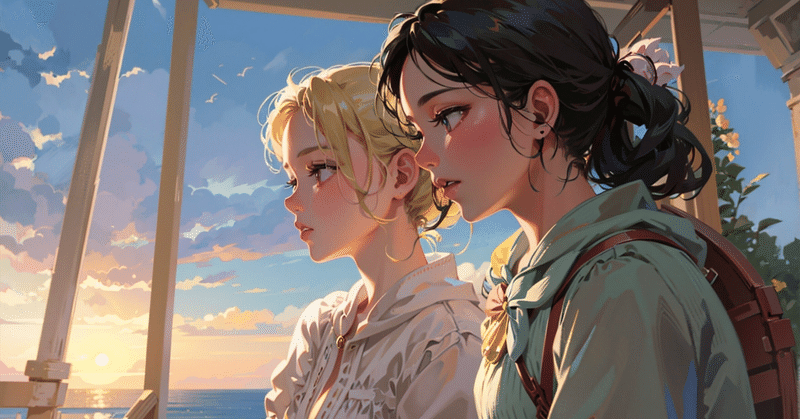
Photo by
dongmu
随時追記 Open Interpreter遊んでみたメモ
公式サイト
環境構築
pip install open-interpreter
環境汚したくない人はpyenvなりanacondaなりで仮想環境作って↑を実行する。
起動方法
interpreter
GPT-3.5使う場合↓
interpreter --fast
Llama使う場合↓
interpreter --local
ケース1. 京都でやってる映画をリストアップ
> Please write web scraping script in Python
Sure! I can help you with that. Here's a plan to write a web scraping script in Python:
1 Import the necessary libraries: We'll need the requests library to send HTTP requests and the BeautifulSoup library to parse the HTML content.
2 Send a GET request to the website: Use the requests.get() function to send a GET request to the website you want to scrape. Make sure to store the response in a
variable.
3 Parse the HTML content: Use BeautifulSoup to parse the HTML content of the website. This will allow us to extract specific elements from the page.
4 Find the elements you want to scrape: Use the find() or find_all() methods of BeautifulSoup to locate the specific elements you want to scrape. You can use CSS
selectors or other methods to find the elements.
5 Extract the data: Once you have located the elements, use the appropriate methods to extract the data you need. This could be the text content, attributes, or other
information.
6 Store the data: You can store the scraped data in a file, database, or any other desired format.
7 Handle pagination or multiple pages: If the data you want to scrape is spread across multiple pages, you'll need to handle pagination. This may involve iterating
through multiple pages and extracting the data from each page.
8 Handle errors and exceptions: It's important to handle any errors or exceptions that may occur during the scraping process. This could include handling HTTP errors,
handling missing elements, or handling other issues that may arise.
9 Test and debug: Finally, test your script on different websites and make sure it's working as expected. Debug any issues that arise and make necessary adjustments to
your code.
出してくれたサンプルコードがこれ。あくまでサンプルなのでこのままでは映画のリストアップができない
> Please run these
import requests
from bs4 import BeautifulSoup
# Send a GET request to the website
response = requests.get('https://example.com')
# Parse the HTML content
soup = BeautifulSoup(response.content, 'html.parser')
# Find the elements you want to scrape
title = soup.find('h1').text
paragraph = soup.find('p').text
# Print the extracted data
print('Title:', title)
print('Paragraph:', paragraph)
Would you like to run this code? (y/n)
> y
File "<stdin>", line 6, in <module>
ModuleNotFoundError: No module named 'bs4'
It seems that the BeautifulSoup library is not installed on your system. To proceed with the web scraping script, we need to install the beautifulsoup4 package.
Please run the following command to install the required package:
!pip install beautifulsoup4
Once the installation is complete, we can proceed with running the web scraping script. Let me know if you have any questions or if you encounter any issues during the
installation process.
> yes run it
!pip install beautifulsoup4
Would you like to run this code? (y/n)
> y
こっから、package installに失敗したり、質問がループしたりで進まなくなってきたけどなんだかんだで必要packageのインストールには成功
Collecting beautifulsoup4
Using cached beautifulsoup4-4.12.2-py3-none-any.whl (142 kB)
Collecting soupsieve>1.2 (from beautifulsoup4)
Obtaining dependency information for soupsieve>1.2 from
https://files.pythonhosted.org/packages/4c/f3/038b302fdfbe3be7da016777069f26ceefe11a681055ea1f7817546508e3/soupsieve-2.5-py3-none-any.whl.metadata
Downloading soupsieve-2.5-py3-none-any.whl.metadata (4.7 kB)
Downloading soupsieve-2.5-py3-none-any.whl (36 kB)
Installing collected packages: soupsieve, beautifulsoup4
Successfully installed beautifulsoup4-4.12.2 soupsieve-2.5
このあと、サンプルコードの向き先を映画館のサイトに変えたり、そのサイトの映画タイトルのタグ要素を教えてあげて一応リストアップはできた
> Please list elements using js-title-film class in https://tjoy.jp/t-joy_kyoto
import requests
from bs4 import BeautifulSoup
# Send a GET request to the website
response = requests.get('https://tjoy.jp/t-joy_kyoto')
# Parse the HTML content
soup = BeautifulSoup(response.content, 'html.parser')
# Find the elements with the js-title-film class
elements = soup.find_all(class_='js-title-film')
# Extract and print the text of the elements
for element in elements:
print(element.text.strip())
Here are the movie titles currently showing at T-Joy Kyoto:
• 禁じられた遊び(PG12)
• 【公開記念舞台挨拶中継】禁じられた遊び(PG12)
• 劇場版シティーハンター 天使の涙(エンジェルダスト)
• 「劇場版シティーハンター 天使の涙(エンジェルダスト)」聖地新宿舞台挨拶 ライブビューイング
• 【字幕】ヒンターラント(PG12)
• 【字幕】ドラキュラ/デメテル号最期の航海(PG12)
• Stray Kids 5-STAR Dome Tour 2023 Live Viewing
• 【字幕】ホーンテッドマンション
• 【吹替】ホーンテッドマンション
• こんにちは、母さん
• スイート・マイホーム
• 【字幕】アステロイド・シティ
• 爆竜戦隊アバレンジャー20th 許されざるアバレ
• 【日本語字幕付】Gメン
• 【2D字幕】MEG ザ・モンスターズ2
• 【2D吹替】MEG ザ・モンスターズ2
• 春に散る
• BE:the ONE
• 【声だしOK!ライブ上映会】BE:the ONE
• 映画『SAND LAND』
• リボルバー・リリー
• 【字幕】バービー
• ミンナのウタ
• しん次元!クレヨンしんちゃんTHE MOVIE 超能力大決戦 ~とべとべ手巻き寿司~
• 【吹替】マイ・エレメント
• 【2D吹替】トランスフォーマー/ビースト覚醒
• キングダム 運命の炎
• 映画『仮面ライダーギーツ 4人のエースと黒狐』/映画『王様戦隊キングオージャー アドベンチャー・ヘブン』
• 【吹替】RRR
• 【字幕】ミッション:インポッシブル/デッドレコニング PART
でもさ、ここでハタと気がついたワケ。取得先のサイトと要素まで指定するんだったらその時点でワシはサイト見てるわけで、もう自分でやった方が速いし、余計な手間かかってるわとwww
まあ、この辺もユースケースごとにこうするんだよっていうのをライブラリ内でうまく隠蔽すればいいので、1年もしたらこういうことも無くなるだろうけど
ちょっと残念だったけど あの頃夢見てたSFにまた近づいたな という感想でした
ケース2. 普通の動画に字幕をつけて怖くしてもらう
そのうちやる
この記事が気に入ったらサポートをしてみませんか?