一週間で身につくJSP/Servlet やってみた
これを最初からやった。
JSP, Servletについて
ページ上部の「基本編」はJSP, Servletなどのについての説明。
静的: 動きのないページ
動的: 動きのあるページ。具体的には表示される日時が変わる、フォームがあるページのこと
JSP(JavaServer Pages): HTMLの中にJavaの処理の埋め込みが可能なる仕組み
Servlet: Web上で実行されるJavaプログラムのこと
実行にはアプリケーションサーバが必要になり、たいていTomcatを使う
サンプル
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Sample01</title>
</head>
<body>
<%
for(int i = 0; i < 5; i++){
out.println("<p>JSP Sample</p>");
}
%>
</body>
</html>
<%= 式 %>
<%= 変数 %> <%= i %>
<%= 演算 %> <%= i + 1 %>
<%= メソッド呼び出し %>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Sample02</title>
</head>
<body>
<!-- テーブルのサンプル -->
<b>テーブルのサンプル</b><br/>
<table border="1">
<tr>
<th width="50">No.</th><th width="100">名前</th>
</tr>
<%String[] name = {"山田太朗","太田京子","佐藤隆俊","鈴木喜一"}; %>
<%for(int i = 0; i < name.length ; i++){ %>
<tr>
<th><%=i %></th><td><%= name[i] %></td>
</tr>
<%} %>
</table>
<!-- プルダウンのサンプル -->
<b>プルダウンのサンプル</b><br/>
<%String[] subjects = {"国語","数学","理科","社会","英語"}; %>
<select>
<%for(int i = 0; i < subjects.length ; i++){ %>
<option value="<%= i %>"><%= subjects [i] %></option>
<%} %>
</select>
</body>
</html>
get送信の例
<form id="frm1" name="frm1" action="sample05.html" method="get">
sample1_from.jsp
sample1_to.jspに遷移する
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample1_from</title>
</head>
<body>
<br/>
<% String links[] = {"link1","リンク2" }; %>
<% for(int i = 0; i < links.length ; i++){%>
<a href="sample1_to.jsp?text=<%= links[i]%>">リンク処理<%=i %></a>
<br/>
<% } %>
</body>
</html>
sample1_to.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample2_to</title>
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
String text = request.getParameter("text");
%>
<b>受信結果</b><br/>
<p><%=text %></p>
<a href="sample1_from.jsp">戻る</a>
</body>
</html>
post送信の例
<form action="sample1_to.jsp" method="post">
sample1_from.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample1_from</title>
</head>
<body>
<form action="sample1_to.jsp" method="post">
<table>
<tr>
<th colspan="2">名前</th>
</tr>
<tr>
<th>姓</th><td><input type="text" id="family" name="family"/></td>
</tr>
<tr>
<th>名</th><td><input type="text" id="name" name="name"/></td>
</tr>
</table>
<br/>
<p><b>性別</b>
<input type="radio" id="sex" name="sex" value="男" checked>男
<input type="radio" id="sex" name="sex" value="女" >女
</p>
<br/>
<b>年代</b><br/>
<% String age[] = {"10歳未満","10代","20代","30代","40代以上"}; %>
<select id="age_id" name="age_id">
<%for(int i = 0; i < age.length ; i++){ %>
<option value="<%= i %>"><%= age[i] %></option>
<%} %>
</select>
<br/>
<br/>
<input type="submit" id="送信" name="送信" value="送信" />
</form>
</body>
</html>
sample1_to.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample1_to</title>
</head>
<body>
<%
String age[] = {"10歳未満","10代","20代","30代","40代以上"};
request.setCharacterEncoding("utf-8");
String family = request.getParameter("family");
String name = request.getParameter("name");
String sex = request.getParameter("sex");
String age_area = age[Integer.parseInt(request.getParameter("age_id"))];
%>
<b>受信結果</b>
<table>
<tr>
<th>姓</th><td><%=family %></td>
</tr>
<tr>
<th>名</th><td><%=name %></td>
</tr>
<tr>
<th>性別</th><td><%=sex %></td>
</tr>
<tr>
<th>年代</th><td><%=age_area %></td>
</tr>
</table>
<br/>
<a href="sample2_from.jsp">戻る</a>
</body>
</html>
これは早い話がページを跨いでもデータを保持できるということ。
sample1_from.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample1_from.jsp</title>
</head>
<body>
<h2>sample1_from.jsp</h2>
<!-- セッションIDを取得 -->
<p>session ID:<%= session.getId() %></p>
<!-- セッションで値を設定 -->
<%
session.setAttribute("foo","bar");
session.setAttribute("hoge","fuga");
%>
<p><a href = "sample1_to.jsp">sample1_to.jsp</a></p>
</body>
</html>
sample1_to.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>sample1_to.jsp</title>
</head>
<body>
<h2>sample1_to.js</h2>
<p>session ID:<%= session.getId() %></p>
<p>foo:<%= session.getAttribute("foo") %></p>
<p>hoge:<%= session.getAttribute("hoge") %></p>
<h2>request Object</h2>
<p>Referer:<%= request.getHeader("referer") %></p>
<p><a href = "sample1_from.jsp">sample1_from.jsp</a></p>
</body>
</html>
セッションIDの取得
session.getId()
セッションでの値の設定
session.setAttribute("名前","値")
セッションの値の取得
session.getAttribute("名前");
サーブレット: サーバ上でウェブページなどを動的に生成したりデータ処理を行うために、Javaで作成されたプログラム
JSP -> HTML内にプログラムを埋め込む
サーブレット -> HTMLを生成できるJavaプログラム
基本的に両者は同じもので、JSPも最終的には内部的にサーブレットに変換されて出力される
しかし、主な使用目的が違う。
JSP -> Webアプリの表示部分に特化している
サーブレット -> 処理の部分に特化している
表示部分はJSP、サーバーサイド処理はサーブレット
package day6;
import java.io.*;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class Sample02
*/
@WebServlet("/day6/Sample02")
public class Sample02 extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Sample02() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("utf-8");
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.println(output("Get"));
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("utf-8");
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.println(output("Post"));
}
public StringBuffer output(String type){
StringBuffer sb = new StringBuffer();
sb.append("<html>");
sb.append("<head>");
sb.append("<title>Sample02</title>");
sb.append("</head>");
sb.append("<body>");
sb.append("<p>呼び出し方法:" + type + "送信</p>");
sb.append("<a href='Sample02'>リンク</a>");
sb.append("<form action='Sample02' method='get'>");
sb.append("<input type='submit' value='GET送信' />");
sb.append("</form>");
sb.append("<form action='Sample02' method='post'>");
sb.append("<input type='submit' value='POST送信' />");
sb.append("</form>");
sb.append("</body>");
sb.append("</html>");
return (sb);
}
}
表示側
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.util.*" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>カレンダー</title>
<style type="text/css">
<!--
*{padding:5px; margin:0px;}
body{text-align:center;}
table{width:800px; background:white; border:2px black solid; border-collapse:collapse;}
th{border:1px black solid; background:#CCFFFF;}
td{border:1px black solid; text-align:right; padding:5px 20px 5px 20px;}
br{line-height:1em;}
-->
</style>
</head>
<body>
<b><%= request.getAttribute("year") %>年<%=request.getAttribute("month") %>月のカレンダー</b>
<br/>
<br/>
<%= request.getAttribute("calender") %>
<br/>
<b>カレンダーの変更</b>
<br/>
<div style="text-align:center;">
<form action="CalenderAccess" method="get">
<select id="year" name="year">
<%
int year = Integer.parseInt(request.getAttribute("year").toString());
int month = Integer.parseInt(request.getAttribute("month").toString());
for(int i = year-10; i <= year+10; i++){
%>
<option value="<%=i %>"
<%
if(i == year){
%>
selected
<%
}
%>
><%=i %>年</option>
<%
}
%>
</select>
<select id="moneth" name="month">
<%
for(int i = 1; i <= 12; i++){
%>
<option value="<%=i %>"
<%
if(i == month){
%>
selected
<%
}
%>
><%=i %>月</option>
<%
}
%>
</select>
<br/>
<br/>
<input type="submit" id="ok" name="ok" value="送信"/>
</form>
</div>
</body>
</html>
サーバーサイド処理側
package day7;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.*;
/**
* Servlet implementation class CalenderAccess
*/
@WebServlet("/day7/CalenderAccess")
public class CalenderAccess extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public CalenderAccess() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
prepData(request);
RequestDispatcher dispatcher = request.getRequestDispatcher("Calender.jsp");
// フォワードによるページ遷移
dispatcher.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
}
// 送信用のデータの作成
private void prepData(HttpServletRequest request){
// 変数初期化
int startday;
int lastday;
// カレンダーの取得
Calendar cal = Calendar.getInstance();
// 年が設定されていれば、その値を取得。そうでなければ、今年の年号を入れる
if(request.getParameter("year")==null){
request.setAttribute("year", cal.get(Calendar.YEAR)); // 現在の年
}else{
request.setAttribute("year", request.getParameter("year")); // 現在の年
}
if(request.getParameter("month")==null){
request.setAttribute("month", cal.get(Calendar.MONTH)+1); // 現在の月
}else{
request.setAttribute("month", request.getParameter("month")); // 与えらられた月
}
int year = Integer.parseInt(request.getAttribute("year").toString());
int month = Integer.parseInt(request.getAttribute("month").toString());
// 月初めの曜日(日-> 1)
cal.set(year, month - 1, 1);
startday = cal.get(Calendar.DAY_OF_WEEK);
// 月末の日付
cal.add(Calendar.MONTH, 1);
cal.add(Calendar.DATE, -1);
lastday = cal.get(Calendar.DATE);
// カレンダーのデータを作成する
int date = 1;
int maxday = 6 * 7;
StringBuilder sb = new StringBuilder();
sb.append("<table>");
sb.append("<tr>");
sb.append("<th style=¥"color:red;¥">日</th>");
sb.append("<th>月</th><th>火</th><th>水</th><th>木</th><th>金</th>");
sb.append("<th style=¥"color:blue;¥">土</th>");
sb.append("</tr>");
sb.append("<tr>");
for (int num = 1; num <= maxday; num++) {
if(num < startday || num > lastday + startday - 1){
sb.append("<td></td>");
}else{
sb.append("<td>"+date+"</td>");
date++;
}
if(num % 7 == 0){
sb.append("</tr>");
if(num > startday + lastday - 1){
break;
}
if(date < lastday){
sb.append("<tr>");
}else{
// 最後だったら、ループから抜ける
break;
}
}
}
sb.append("</table>");
// パラメータを設定
request.setAttribute("calender", sb);
return;
}
}
Eclipseでの開発
実際にEclipseを用いての開発は「統合開発環境」の項目で行う。
tomcatの設定とプロジェクトの作成は「Apache/Tomcatサーバーの起動」のページを読むこと
sample.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>Sample サンプル</h1>
</body>
</html>
package sample;
import java.io.*;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class Sample01
*/
@WebServlet("/sample/Sample")
public class Sample extends HttpServlet{
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Sample() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>サーブレットのサンプル</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>サーブレットのサンプル</h1>");
out.println("</body>");
out.println("</html>");
}
}
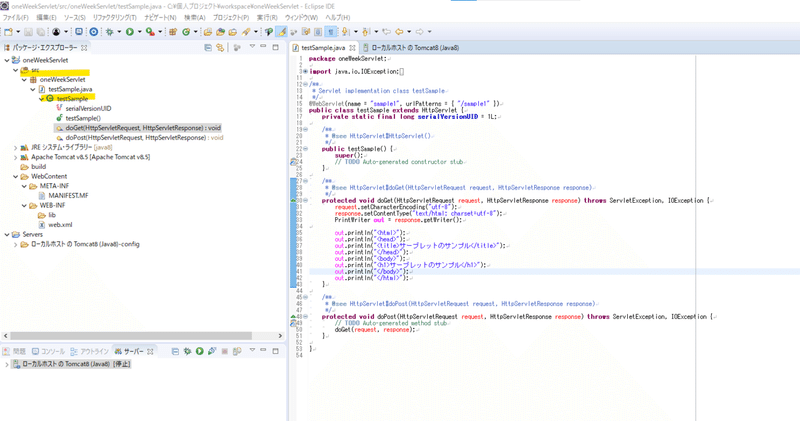
この記事が気に入ったらサポートをしてみませんか?