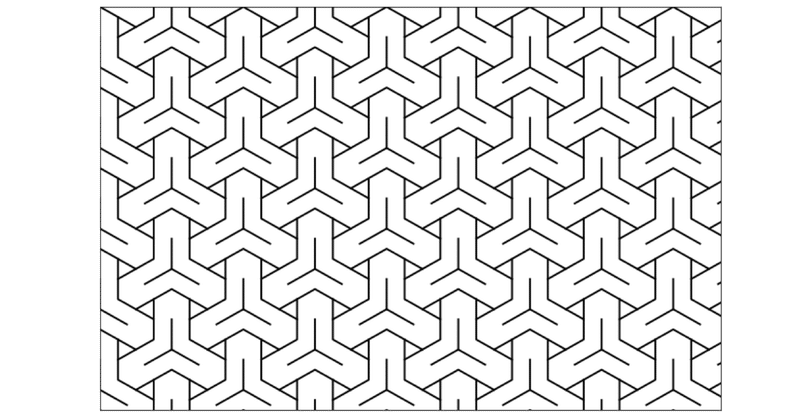
刺し子模様をPythonで描く:亀甲・毘沙門亀甲
亀甲
亀甲は亀の甲羅で,六角形が基本。それをつないだいろいろなバリエーションがある。たとえば,同心円状に3つの正六角形を描いたものを並べたもの。
一つ描くだけなら六角形を3つ描けばよいが,つないで描くときに,刺し子では同じところを通らないようにするので,同じように考えて,外側の六角形は半分だけ描くことにする。(実際の刺し子の手順とは異なる)
この模様を手で描くなら,斜眼用紙を使うが,麻の葉や斜紗綾形で使ったものとは向きが異なる。斜紗綾形で使ったものは,縦軸が斜めになっているもの。
ここで使うのは,横軸が斜めになっているものだ。
前者を stype = 1, 後者を stype = 2 として,syagan(stype, plist) で変換する。斜眼のページに書いたもの。
次のプログラムで,basicpattern() が亀甲の定義で,それより前は今まで使っている共通の関数だ。
import numpy as np
import matplotlib.pyplot as plt
plt.figure(figsize=(8, 6))
plt.axis([10, 38, 0, 21])
plt.xticks([])
plt.yticks([])
# 点を線分で結ぶ
def draw(org, plist):
x = []
y = []
for pt in plist:
x.append(org[0]+pt[0])
y.append(org[1]+pt[1])
plt.plot(x, y, lw=1, color=Drawcolor)
# 横方向にm,縦方向にn個のパターンを描く
def drawpattern(m, n):
for x in range(m):
for y in range(n):
org = x*Shiftx+ y*Shifty
basicpattern(org)
# 斜眼に変換
def syagan(stype,plist):
if stype == 1:
ex = np.array([1, 0])
ey = np.array([0.5, np.sqrt(3)/2])
else:
ex = np.array([np.sqrt(3)/2, 0.5])
ey = np.array([0, 1])
ret = []
for i in range(len(plist)):
p = plist[i]
ret.append(list(p[0]*ex + p[1]*ey))
return ret
# 基本パターンをorgを原点として描く
def basicpattern(org):
p1 = [1, 0]
p2 = [0, 1]
p3 = [-1, 1]
p4 = [-1, 0]
p5 = [0, -1]
p6 = [1, -1]
p7 = [2, 0]
p8 = [0, 2]
p9 = [-2, 2]
p10 = [-2, 0]
p11 = [0, -2]
p12 = [2, -2]
p13 = [0, 3]
p14 = [-3, 3]
p15 = [-3, 0]
p16 = [0, -3]
draw(org, syagan(2, [p1, p2, p3, p4, p5, p6, p1]))
draw(org, syagan(2, [p7, p8, p9, p10, p11, p12, p7]))
draw(org, syagan(2, [p13, p14, p15, p16]))
# 横方向のシフト量,縦方向のシフト量,描画色を定義して描く
Shiftx = np.array([3*np.sqrt(3), 0])
p = syagan(2, [[3, 3]])
Shifty = np.array(p[0])
Drawcolor = 'k'
drawpattern(10, 10)
#plt.savefig("kikkou.png")
plt.show()
毘沙門亀甲
亀甲のバリエーションの一つで,丸毘沙門に対して,まっすぐな線にしたのが毘沙門亀甲。亀甲の模様のバリエーションのひとつだ。(見出し画像)
基本パターンは次のもの。中の線を入れないものもある。
これで座標を拾っていく。
def basicpattern(org):
p1 = [-1, 3]
p2 = [-1, 1]
p3 = [-3, 1]
p4 = [-2, -1]
p5 = [0, -1]
p6 = [2, -3]
p7 = [3, -2]
p8 = [1, 0]
p9 = [1, 2]
p10 = [0, 1.5]
p11 = [0, 0]
p12 = [-1.5, 0]
p13 = [1.5, -1.5]
draw(org, syagan(2, [p1, p2, p3]))
draw(org, syagan(2, [p4, p5, p6]))
draw(org, syagan(2, [p7, p8, p9]))
draw(org, syagan(2, [p10, p11, p12]))
draw(org, syagan(2, [p11, p13]))
描画設定の平行移動量は次の通り。
Shiftx = np.array([2*np.sqrt(3),2])
Shifty = np.array([0, 4])
できたのが見出し画像だ。