赤外線センサー ボタンによって好きな音(mp3)を流しました。DFPlayer
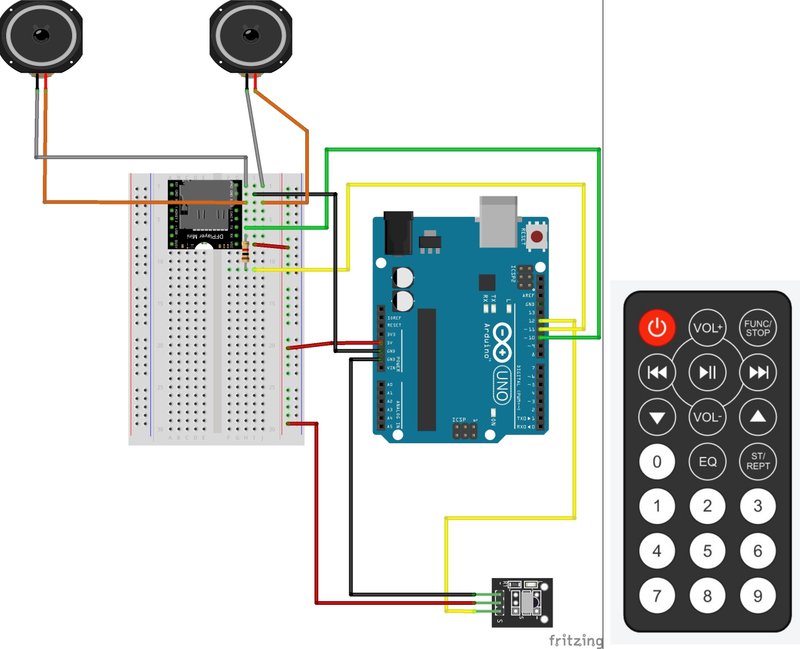
抵抗は1kΩ
最初自分の声を録音していてノイズだらけではまったけど、自分の声をきれいに録音すればよかっただけ。
あと音が小さい問題も audacity で音を大きくすればいい ことに気づいた。
曲の取り込み↓
効果音頂きました。
参考になりました。ありがとうございます。
赤外線の信号の値をとってくるプログラム
/*
* IRremote: IRrecvDump - dump details of IR codes with IRrecv
* An IR detector/demodulator must be connected to the input RECV_PIN.
* Version 0.1 July, 2009
* Copyright 2009 Ken Shirriff
* http://arcfn.com
* JVC and Panasonic protocol added by Kristian Lauszus (Thanks to zenwheel and other people at the original blog post)
* LG added by Darryl Smith (based on the JVC protocol)
*/
#include <IRremote.h>
int RECV_PIN = 12;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
Serial.begin(9600);
irrecv.enableIRIn(); // Start the receiver
}
// Dumps out the decode_results structure.
// Call this after IRrecv::decode()
// void * to work around compiler issue
//void dump(void *v) {
// decode_results *results = (decode_results *)v
void dump(decode_results *results) {
int count = results->rawlen;
if (results->decode_type == UNKNOWN) {
Serial.print("Unknown encoding: ");
}
else if (results->decode_type == NEC) {
Serial.print("Decoded NEC: ");
}
else if (results->decode_type == SONY) {
Serial.print("Decoded SONY: ");
}
else if (results->decode_type == RC5) {
Serial.print("Decoded RC5: ");
}
else if (results->decode_type == RC6) {
Serial.print("Decoded RC6: ");
}
else if (results->decode_type == PANASONIC) {
Serial.print("Decoded PANASONIC - Address: ");
Serial.print(results->panasonicAddress,HEX);
Serial.print(" Value: ");
}
else if (results->decode_type == LG) {
Serial.print("Decoded LG: ");
}
else if (results->decode_type == JVC) {
Serial.print("Decoded JVC: ");
}
Serial.print(results->value, HEX);
Serial.print(" (");
Serial.print(results->bits, DEC);
Serial.println(" bits)");
Serial.print("Raw (");
Serial.print(count, DEC);
Serial.print("): ");
for (int i = 0; i < count; i++) {
if ((i % 2) == 1) {
Serial.print(results->rawbuf[i]*USECPERTICK, DEC);
}
else {
Serial.print(-(int)results->rawbuf[i]*USECPERTICK, DEC);
}
Serial.print(" ");
}
Serial.println("");
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
dump(&results);
irrecv.resume(); // Receive the next value
}
}
上のプログラムを書き込んでシリアルモニタを開く。
ボタンを押したら
「FFC23D
Decoded NEC: FFC23D (32 bits)
Raw (68): -29926 9250 -4400 650 -450 650 -550 650 -450 700 -550 650 -500 650 -450 750 -450 700 -500 650 -1600 600 -1650 650 -1600 600 -1650 600 -1600 600 -1650 650 -1600 600 -1650 600 -1600 650 -1600 650 -500 650 -500 650 -500 650 -550 600 -1650 600 -550 650 -500 650 -500 650 -1600 650 -1600 600 -1650 600 -1650 600 -500 650 -1650 600
FF22DD
Decoded NEC: FF22DD (32 bits)
Raw (68): -10834 9200 -4450 600 -500 700 -450 700 -500 650 -500 650 -550 600 -550 650 -450 700 -500 650 -1600 650 -1600 650 -1550 650 -1600 650 -1600 600 -1600 650 -1600 650 -1600 600 -500 700 -450 700 -1600 600 -500 700 -500 650 -450 700 -1600 650 -500 650 -1600 650 -1600 650 -450 700 -1600 600 -1600 650 -1600 650 -500 650 -1600 650
FF30CF
Decoded NEC: FF30CF (32 bits)
Raw (68): 3962 9200 -4450 650 -550 600 -550 600 -600 600 -550 600 -550 600 -550 600 -600 600 -550 600 -1650 600 -1650 600 -1600 600 -1650 600 -1650 600 -1650 550 -1650 600 -1650 600 -550 600 -600 550 -1650 600 -1650 600 -550 600 -600 600 -550 600 -550 600 -1650 600 -1650 600 -550 600 -600 550 -1650 600 -1650 600 -1650 600 -1650 550 」
値がとれるのでこれを下のプログラムにははりつけ。
とりま完成プログラム↓
/*
**************************************************
DFPlayer - A Mini MP3 Player For Arduino
<https://www.dfrobot.com/index.php?route=product/product&product_id=1121>
***************************************************
This example shows the basic function of library for DFPlayer.
Created 2016-12-07
By [Angelo qiao](Angelo.qiao@dfrobot.com)
GNU Lesser General Public License.
See <http://www.gnu.org/licenses/> for details.
All above must be included in any redistribution
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<https://www.dfrobot.com/wiki/index.php/DFPlayer_Mini_SKU:DFR0299#Connection_Diagram>
2.This code is tested on Arduino Uno, Leonardo, Mega boards.
****************************************************/
#include "Arduino.h" #include "SoftwareSerial.h" #include "DFRobotDFPlayerMini.h"
#include <IRremote.h> #define T 15
int RECV_PIN = 12;
IRrecv irrecv(RECV_PIN);
decode_results results;
//boolean flechas = false;
boolean rotacion = false;
SoftwareSerial mySoftwareSerial(10, 11); // RX, TX
DFRobotDFPlayerMini myDFPlayer;
void printDetail(uint8_t type, int value);
void IRreceived(long code) {
//here i have mapped some buttons of my blueray remote.
if (code == 0xFF30CF) {
myDFPlayer.play(1);
}
else if (code == 0xFF18E7) {
myDFPlayer.play(2);
}
else if (code == 0xFF7A85) {
myDFPlayer.play(3);
}
else if (code == 0xFF10EF) {
myDFPlayer.play(4);
}
else if (code == 0xFF38C7) {
myDFPlayer.play(5);
}
else if (code == 0xFF5AA5) {
myDFPlayer.play(6);
}
else if (code == 0xFF42BD) {
myDFPlayer.play(7);
}
else if (code == 0xFF4AB5) {
myDFPlayer.play(8);
}
else if (code == 0xFF52AD) {
myDFPlayer.play(9);
}
else if (code == 0xFF10EF) {
myDFPlayer.play(10);
}
else if (code == 0xFF6897) {
myDFPlayer.play(11);
}
else if (code == 0xFF629D) {
myDFPlayer.volumeUp();
}
else if (code == 0xFFA857) {
myDFPlayer.volumeDown();
}
else {
Serial.print(results.value, HEX);
Serial.println("-----DESCONOCIDO!");
}
}
void setup()
{
pinMode(0, OUTPUT);
pinMode(1, OUTPUT);
irrecv.enableIRIn(); // Start the receiver
mySoftwareSerial.begin(9600);
Serial.begin(115200);
Serial.println();
Serial.println(F("DFRobot DFPlayer Mini Demo"));
Serial.println(F("Initializing DFPlayer ... (May take 3~5 seconds)"));
if (!myDFPlayer.begin(mySoftwareSerial)) { //Use softwareSerial to communicate with mp3.
Serial.println(F("Unable to begin:"));
Serial.println(F("1.Please recheck the connection!"));
Serial.println(F("2.Please insert the SD card!"));
while(true);
}
Serial.println(F("DFPlayer Mini online."));
myDFPlayer.volume(30); //Set volume value. From 0 to 30
//// myDFPlayer.play(1); //Play the first mp3
// myDFPlayer.play(0002); //Play the first mp3
// myDFPlayer.play(0003); //Play the first mp3
// myDFPlayer.play(0004); //Play the first mp3
// myDFPlayer.play(0005); //Play the first mp3
// myDFPlayer.play(0006); //Play the first mp3
// myDFPlayer.play(0007); //Play the first mp3
// myDFPlayer.play(0008); //Play the first mp3
}
void loop()
{
static unsigned long timer = millis();
if (millis() - timer > 3000) {
/*
timer = millis();
myDFPlayer.next(); //Play next mp3 every 3 second.
}
*/
if (irrecv.decode(&results)) {
//DellRemote(results.value);
IRreceived(results.value);
//mapping(results.value);
delay(300);//estaba en 100//500 es muy largo
irrecv.resume(); // Receive the next value
} } }
// if (myDFPlayer.available()) {
// printDetail(myDFPlayer.readType(), myDFPlayer.read()); //Print the detail message from DFPlayer to handle different errors and states.
// }
//}
//
//void printDetail(uint8_t type, int value){
// switch (type) {
// case TimeOut:
// Serial.println(F("Time Out!"));
// break;
// case WrongStack:
// Serial.println(F("Stack Wrong!"));
// break;
// case DFPlayerCardInserted:
// Serial.println(F("Card Inserted!"));
// break;
// case DFPlayerCardRemoved:
// Serial.println(F("Card Removed!"));
// break;
// case DFPlayerCardOnline:
// Serial.println(F("Card Online!"));
// break;
// case DFPlayerPlayFinished:
// Serial.print(F("Number:"));
// Serial.print(value);
// Serial.println(F(" Play Finished!"));
// break;
// case DFPlayerError:
// Serial.print(F("DFPlayerError:"));
// switch (value) {
// case Busy:
// Serial.println(F("Card not found"));
// break;
// case Sleeping:
// Serial.println(F("Sleeping"));
// break;
// case SerialWrongStack:
// Serial.println(F("Get Wrong Stack"));
// break;
// case CheckSumNotMatch:
// Serial.println(F("Check Sum Not Match"));
// break;
// case FileIndexOut:
// Serial.println(F("File Index Out of Bound"));
// break;
// case FileMismatch:
// Serial.println(F("Cannot Find File"));
// break;
// case Advertise:
// Serial.println(F("In Advertise"));
// break;
// default:
// break;
// }
// break;
// default:
// break;
// }
//}
//
この記事が気に入ったらサポートをしてみませんか?