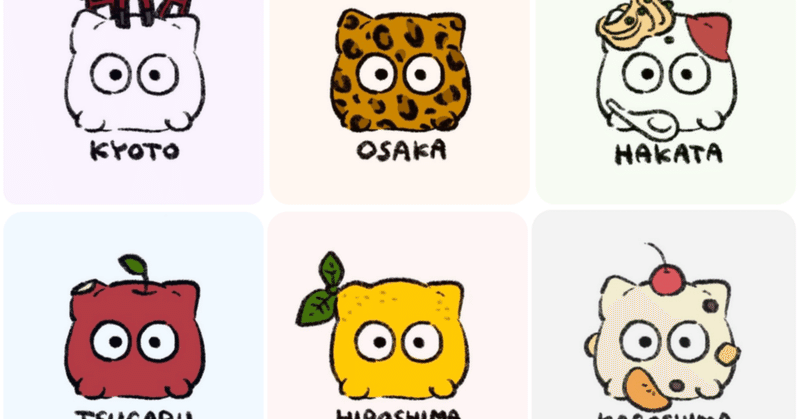
【ミーア】LINEリッチメニューで複数方言に対応できるようにする:方言切り替え記憶を保存②
現状だと、方言切り替えを保存できていない問題
前回、下記記事で、LINEリッチメニューで複数方言に対応できるようにしたのだが、方言切り替えが保存されずに、標準語での応答に戻ってしまう問題を解決していなかった。
現在のコードでは、ユーザーが方言を選択すると**handle_postback関数がトリガーされ、選択された方言に基づいて応答が生成される。しかし、その後のテキストメッセージが送信されるとhandle_message**関数がトリガーされ、ここでは方言の設定が考慮されていないため、応答が標準語に戻ってしまう。
import os
from datetime import datetime
from flask import Request, abort
from google.cloud import firestore
from linebot import LineBotApi, WebhookHandler
from linebot.exceptions import InvalidSignatureError
from linebot.models import MessageEvent, TextMessage, TextSendMessage, PostbackEvent
from openai import OpenAI
line_bot_api = LineBotApi(os.environ["LINE_CHANNEL_ACCESS_TOKEN"])
handler = WebhookHandler(os.environ["LINE_CHANNEL_SECRET"])
db = firestore.Client()
client = OpenAI()
USE_HISTORY = True
def get_previous_messages(user_id: str) -> list:
query = (
db.collection("users")
.document(user_id)
.collection("messages")
.order_by("timestamp", direction=firestore.Query.DESCENDING)
.limit(3)
)
return [
{
"chatgpt_response": doc.to_dict()["chatgpt_response"],
"user_message": doc.to_dict()["user_message"],
}
for doc in query.stream()
]
def format_history(previous_messages: list) -> str:
return "".join(
f"ユーザー: {d['user_message']}\\nアシスタント: {d['chatgpt_response']}\\n"
for d in previous_messages[::-1]
)
def load_prompt(dialect: str) -> str:
# 方言に対応するファイルパスを構築
filename = f"dialect_prompt/{dialect}_prompt.txt"
# ファイルからプロンプト文を読み込む
with open(filename, "r", encoding="utf-8") as file:
prompt = file.read()
return prompt
def generate_response(user_message: str, history: str, dialect: str = None) -> str:
if dialect:
# 方言に基づいたプロンプトを読み込む
prompt = load_prompt(dialect)
else:
# 通常のプロンプトを使用
prompt = "ここに通常のプロンプトを設定"
if USE_HISTORY and history:
history_section = f"これまでの会話履歴:\\n{history}\\n"
else:
history_section = ""
full_prompt = f"{prompt}\\n{history_section}\\nユーザーからのメッセージ: {user_message}"
# OpenAI APIを使用して応答を生成
chatgpt_response = client.chat.completions.create(
model="gpt-3.5-turbo-1106",
messages=[{"role": "system", "content": full_prompt},
{"role": "user", "content": user_message}],
temperature=0.2,
)
print(chatgpt_response)
print(type(chatgpt_response))
# 応答のテキスト部分を取得
return chatgpt_response.choices[0].message.content
def reply_to_user(reply_token: str, chatgpt_response: str) -> None:
line_bot_api.reply_message(
reply_token, TextSendMessage(text=chatgpt_response))
def save_message_to_db(user_id: str, user_message: str, chatgpt_response: str) -> None:
doc_ref = db.collection("users").document(
user_id).collection("messages").document()
doc_ref.set(
{
"user_message": user_message,
"chatgpt_response": chatgpt_response,
"timestamp": datetime.now(),
}
)
def main(request: Request) -> str:
signature = request.headers["X-Line-Signature"]
body = request.get_data(as_text=True)
try:
handler.handle(body, signature)
except InvalidSignatureError:
abort(400)
return "OK"
@handler.add(MessageEvent, message=TextMessage)
def handle_message(event: MessageEvent) -> None:
user_id = event.source.user_id
user_message = event.message.text
if USE_HISTORY:
previous_messages = get_previous_messages(user_id)
history = format_history(previous_messages)
else:
history = None
chatgpt_response = generate_response(user_message, history)
reply_to_user(event.reply_token, chatgpt_response)
if USE_HISTORY:
save_message_to_db(user_id, user_message, chatgpt_response)
@handler.add(PostbackEvent)
def handle_postback(event: PostbackEvent):
user_id = event.source.user_id
data = event.postback.data
dialect = data.split('=')[1] if '=' in data else None
if USE_HISTORY:
previous_messages = get_previous_messages(user_id)
history = format_history(previous_messages)
else:
history = None
chatgpt_response = generate_response("", history, dialect)
reply_to_user(event.reply_token, chatgpt_response)
if USE_HISTORY:
save_message_to_db(user_id, "POSTBACK: " + data, chatgpt_response)
というわけで、ユーザーがLINEリッチメニューで選択した方言情報を保持しておかないといけない。
方言選択をFirestoreに保存
続きは、こちらに記載しています。
よろしければサポートお願いします!いただいたサポートはクリエイターとしての活動費に使わせていただきます!