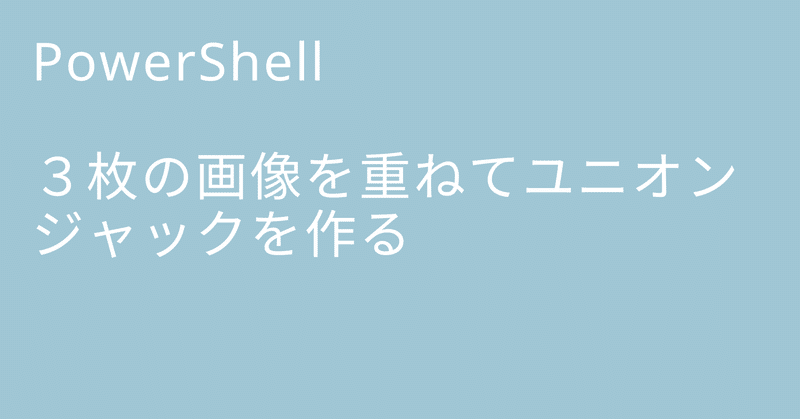
PowerShell 3枚の画像を重ねてユニオンジャックを作る
PowerShell で画像加工をしてみます。
まず左右変転画像を作るサンプル
Add-Type -AssemblyName System.Drawing
function FlipY($src_image, $dst_image){
$image_width = $src_image.Width
$image_height = $src_image.Height
0..($image_width-1) | %{
$x = $_
0..($image_height-1) | %{
$y = $_
#ここでx座標を入れ替えて左右反転させている
$dst_image.SetPixel( ($image_width-1) - $x, $y,$src_image.GetPixel($x, $y))
}
}
return $dst_image
}
# 画像読み込み
$in_image = [System.Drawing.Image]::FromFile("c:\temp\test.png")
# 出力用オブジェクト生成
$out_image = New-Object System.Drawing.Bitmap($in_image.Width,$in_image.Height)
# 画像処理の関数を実行
$out_image = (FlipY $in_image $out_image)
# 画像の保存
$out_image.Save("c:\temp\test_out.png", [System.Drawing.Imaging.ImageFormat]::png)
# オブジェクトを破棄
$in_image.Dispose()
$out_image.Dispose()
c:\temp\test.pngを開いて関数FlipYで左右反転しています。
$dst_image.SetPixel…あたりで1ビットずつx座標位置を入れ替えています。
これを応用して
次は2枚の画像を重ねてミックスするサンプル
#画像をミックスする
Add-Type -AssemblyName System.Drawing
function picmix() {
Param(
$src_image_file, #入力画像ファイル1 フルパス
$src_image2_file, #入力画像ファイル2 フルパス
$dst_image_file #出力画像ファイル フルパス
)
# 画像読み込み
$src_image = [System.Drawing.Image]::FromFile($src_image_file)
$src_image2 = [System.Drawing.Image]::FromFile($src_image2_file)
# Bitmapオブジェクトを出力用として生成
$dst_image_width = $src_image.Width
$dst_image_height = $src_image.Height
if($dst_image_width -gt $src_image2.Width){$dst_image_width=$src_image2.Width}
if($dst_image_height -gt $src_image2.Height){$dst_image_height=$src_image2.Height}
$dst_image = New-Object System.Drawing.Bitmap($dst_image_width,$dst_image_height)
0..($dst_image_width-1) | %{
$x = $_
0..($dst_image_height-1) | %{
$y = $_
$src_a = $src_image.GetPixel($x, $y).A
$src_r = $src_image.GetPixel($x, $y).R
$src_g = $src_image.GetPixel($x, $y).G
$src_b = $src_image.GetPixel($x, $y).B
$src_a2 = $src_image2.GetPixel($x, $y).A
$src_r2 = $src_image2.GetPixel($x, $y).R
$src_g2 = $src_image2.GetPixel($x, $y).G
$src_b2 = $src_image2.GetPixel($x, $y).B
# ピクセルがほぼ白か,ほぼ黒の場合は色を無視する処理
$color_avg = [int](($src_image.GetPixel($x, $y).R + $src_image.GetPixel($x, $y).G + $src_image.GetPixel($x, $y).B) /3)
$color_avg2 = [int](($src_image2.GetPixel($x, $y).R + $src_image2.GetPixel($x, $y).G + $src_image2.GetPixel($x, $y).B) /3)
if( ($color_avg -lt 10) -or ($color_avg -gt 245) ){
$src_r=$src_r2
$src_g=$src_g2
$src_b=$src_b2
} elseif( ($color_avg2 -lt 10) -or ($color_avg2 -gt 245) ){
$src_r2=$src_r
$src_g2=$src_g
$src_b2=$src_b
}
$a = [int]( ($src_a + $src_a2)/2 )
$r = [int]( ($src_r + $src_r2)/2 )
$g = [int]( ($src_g + $src_g2)/2 )
$b = [int]( ($src_b + $src_b2)/2 )
$color = [System.Drawing.Color]::FromArgb($a, $r, $g, $b)
$dst_image.SetPixel($x, $y, $color)
}
}
# 画像の保存
$dst_image.Save($dst_image_file, [System.Drawing.Imaging.ImageFormat]::png)
# オブジェクトを破棄
$src_image.Dispose()
$src_image2.Dispose()
$dst_image.Dispose()
}
「picmix 入力ファイル1 入力ファイル2 出力ファイル」
で重ね合わせできます(ファイルはフルパス)
こういう部分「$r = [int]( ($src_r + $src_r2)/2 )」でRGBのカラー情報の平均値を出力してミックスしています。
そしてこの関数picmixを使って3枚の画像を重ねてユニオンジャックを作ってみます。
3枚画像を用意して
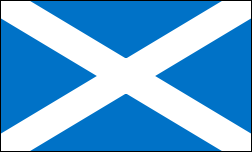
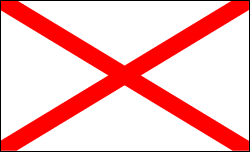
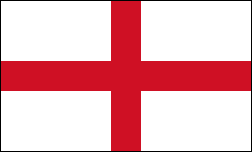
これらをc:\tempフォルダに入れて重ねてみます
PS C:\temp> picmix C:\temp\0.png C:\temp\1.png c:\temp\0-1.png
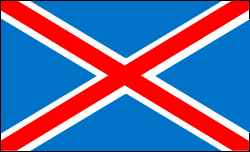
なんかそれっぽい
さらに重ねます
PS C:\temp> picmix C:\temp\0-1.png C:\temp\2.png c:\temp\out.png
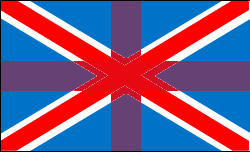
できた!ユニオンジャック
思ってるのと違うけど、できた
#PowerShell #国旗 #ユニオンジャック #プログラミング初心者 #プログラミング学習 #Windows #毎日更新 #毎日Note
この記事が気に入ったらサポートをしてみませんか?