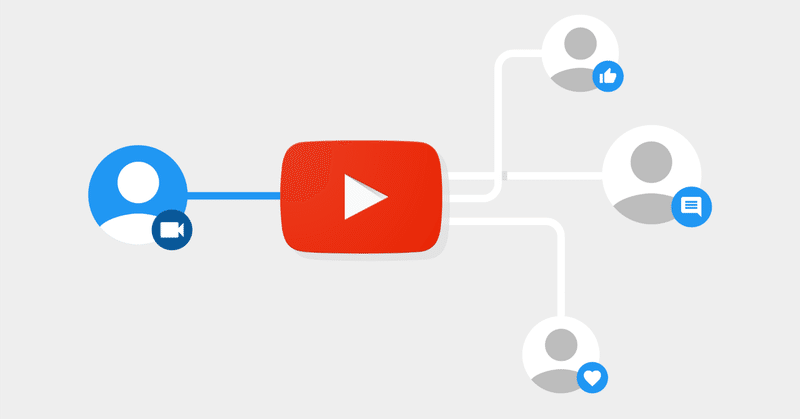
YouTube Data API を使ってみたメモ
Google 公式のYoutubeフィッチライブラリであるYoutube Data APIを用いて、Youtubeデータをフィッチしたので、メモを残しておく。メモなので詳細な説明や懇切丁寧な説明はない。
1.ワード検索を行う(Search: list)
1A. ワード検索で動画を見つける
通常のYoutube検索に近い動画検索を行う場合、
qにて検索名を設定し、typeにてViodeを選択する。
今回はmaxResultsを5とし、5件の検索結果を出力させた。なおmaxResultsの最大値は50。
コード:
# -*- coding: utf-8 -*-
# Sample Python code for youtube.search.list
# See instructions for running these code samples locally:
# https://developers.google.com/explorer-help/code-samples#python
import os
import google_auth_oauthlib.flow
import googleapiclient.discovery
import googleapiclient.errors
scopes = ["https://www.googleapis.com/auth/youtube.force-ssl"]
def main():
# Disable OAuthlib's HTTPS verification when running locally.
# *DO NOT* leave this option enabled in production.
os.environ["OAUTHLIB_INSECURE_TRANSPORT"] = "1"
api_service_name = "youtube"
api_version = "v3"
client_secrets_file = "YOUR_CLIENT_SECRET_FILE.json"
# Get credentials and create an API client
flow = google_auth_oauthlib.flow.InstalledAppFlow.from_client_secrets_file(
client_secrets_file, scopes)
credentials = flow.run_console()
youtube = googleapiclient.discovery.build(
api_service_name, api_version, credentials=credentials)
request = youtube.search().list(
part="snippet",
maxResults=3,
q="Vtuber",
type="video"
)
response = request.execute()
print(response)
if __name__ == "__main__":
main()
レスポンス:
{
"kind": "youtube#searchListResponse",
"etag": "o800RHv2owLTVL5gnMK6u8L9xzY",
"nextPageToken": "CAMQAA",
"regionCode": "JP",
"pageInfo": {
"totalResults": 1000000,
"resultsPerPage": 3
},
"items": [
{
"kind": "youtube#searchResult",
"etag": "_VV6yPUNxDS7XRwW_xB9eero9YM",
"id": {
"kind": "youtube#video",
"videoId": "NwVDn9KkaV4"
},
"snippet": {
"publishedAt": "2024-01-07T23:30:03Z",
"channelId": "UCICvQAnA8u-_nWZa_6i81WA",
"title": "Don't You Like It...",
"description": "Buff just really likes you! If you'd like to support me you can donate here - https://streamlabs.com/obivtuber1 Buff's Social's: Twitch ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "Obi Vtuber Clips",
"liveBroadcastContent": "none",
"publishTime": "2024-01-07T23:30:03Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "H-kgMmxWmg6W1rBBCoaacJkF_q0",
"id": {
"kind": "youtube#video",
"videoId": "ZUZwrbxk3Jk"
},
"snippet": {
"publishedAt": "2021-04-08T12:30:12Z",
"channelId": "UCt_oFAUph4_8P3N_Xs-FGHg",
"title": "The Insane Rise of Vtubers and Why I Hate Them",
"description": "For a limited time, grab the 1-year NordPass Premium plan with 50% off at https://nordpass.com/scambolireviews or use code ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/ZUZwrbxk3Jk/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/ZUZwrbxk3Jk/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/ZUZwrbxk3Jk/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "Scamboli Reviews",
"liveBroadcastContent": "none",
"publishTime": "2021-04-08T12:30:12Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "45qWMezOMN7mIXkh1BNUlWG1kXw",
"id": {
"kind": "youtube#video",
"videoId": "6QECKU17Gp4"
},
"snippet": {
"publishedAt": "2023-06-11T01:00:05Z",
"channelId": "UCMj4YareOFuEfxUBbncWF9w",
"title": "Japanese VTuber, But She Forgets To Turn On Her Voice Changer",
"description": "This is a story of a Japanese person who forgot to turn on her voice changer on stream I guess The VTuber in this video: ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/6QECKU17Gp4/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/6QECKU17Gp4/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/6QECKU17Gp4/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "Sora The Troll",
"liveBroadcastContent": "none",
"publishTime": "2023-06-11T01:00:05Z"
}
}
]
}
1B.レスポンス結果を解析してみる
以下に配列の内容を項目名ごとに説明を追記した。
{
"kind": "このレスポンスの種類(YouTube Data APIのレスポンスタイプ)",
"etag": "このレスポンスのetag(キャッシュを効率的に管理するための識別子)",
"nextPageToken": "次のページのデータを取得するためのトークン",
"regionCode": "検索が実行された地域のコード",
"pageInfo": {
"totalResults": "検索結果の総数",
"resultsPerPage": "ページあたりの結果数"
},
"items": [
{
"kind": "このアイテムの種類(ここではYouTube動画)",
"etag": "このアイテムのetag",
"id": {
"kind": "このIDの種類(ここではYouTube動画のID)",
"videoId": "動画のID"
},
"snippet": {
"publishedAt": "動画の公開日時",
"channelId": "動画を公開しているチャンネルのID",
"title": "動画のタイトル",
"description": "動画の説明",
"thumbnails": {
"default": {
"url": "標準画質のサムネイルのURL",
"width": "標準画質のサムネイルの幅",
"height": "標準画質のサムネイルの高さ"
},
"medium": {
"url": "中画質のサムネイルのURL",
"width": "中画質のサムネイルの幅",
"height": "中画質のサムネイルの高さ"
},
"high": {
"url": "高画質のサムネイルのURL",
"width": "高画質のサムネイルの幅",
"height": "高画質のサムネイルの高さ"
}
},
"channelTitle": "チャンネルのタイトル",
"liveBroadcastContent": "動画がライブ放送のコンテンツかどうか('none'、'live'、'upcoming')",
"publishTime": "動画が公開された日時"
}
}
// 他のアイテムも同様の構造
]
}
つまり、以下のレスポンス結果より、
{
"kind": "youtube#searchResult",
"etag": "_VV6yPUNxDS7XRwW_xB9eero9YM",
"id": {
"kind": "youtube#video",
"videoId": "NwVDn9KkaV4"
},
"snippet": {
"publishedAt": "2024-01-07T23:30:03Z",
"channelId": "UCICvQAnA8u-_nWZa_6i81WA",
"title": "Don't You Like It...",
"description": "Buff just really likes you! If you'd like to support me you can donate here - https://streamlabs.com/obivtuber1 Buff's Social's: Twitch ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/NwVDn9KkaV4/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "Obi Vtuber Clips",
"liveBroadcastContent": "none",
"publishTime": "2024-01-07T23:30:03Z"
}
},
以下の情報が含まれている
動画タイトル: Don't You Like It...
動画ID: NwVDn9KkaV4
公開日時: 2024-01-07T23:30:03Z
チャンネルID: UCICvQAnA8u-_nWZa_6i81WA
チャンネル名: Obi Vtuber Clips
説明: Buff just really likes you! If you'd like to support me you can donate here - https://streamlabs.com/obivtuber1 Buff's Social's: Twitch ...
ライブ配信コンテンツ: なし
サムネイル:
2.チャンネル内検索を行う(Search: list)
2A.チャンネル内検索をチャンネルIDで行う
特定のチャンネルのコンテンツを検索したい場合、「channelId」を設定することで検索が可能。
コード
# -*- coding: utf-8 -*-
# Sample Python code for youtube.search.list
# See instructions for running these code samples locally:
# https://developers.google.com/explorer-help/code-samples#python
import os
import googleapiclient.discovery
def main():
# Disable OAuthlib's HTTPS verification when running locally.
# *DO NOT* leave this option enabled in production.
os.environ["OAUTHLIB_INSECURE_TRANSPORT"] = "1"
api_service_name = "youtube"
api_version = "v3"
DEVELOPER_KEY = "YOUR_API_KEY"
youtube = googleapiclient.discovery.build(
api_service_name, api_version, developerKey = DEVELOPER_KEY)
request = youtube.search().list(
part="snippet",
channelId="UCxyEJ2PblLJDPxOkN_6ALLw",
maxResults=3,
type="video"
)
response = request.execute()
print(response)
if __name__ == "__main__":
main()
レスポンス:
{
"kind": "youtube#searchListResponse",
"etag": "WFkI_eejZP9_j0l3jZxhjS1wUQ8",
"nextPageToken": "CAMQAA",
"regionCode": "JP",
"pageInfo": {
"totalResults": 73,
"resultsPerPage": 3
},
"items": [
{
"kind": "youtube#searchResult",
"etag": "BD9HDR9PuZQuBekucqn10A4JjRM",
"id": {
"kind": "youtube#video",
"videoId": "OVpYBxK0sCg"
},
"snippet": {
"publishedAt": "2022-10-15T14:25:11Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "流行りのAIにTell Your World食べさせてみた【比較】",
"description": "素晴らしい元動画様! https://www.youtube.com/watch?v=UUaVUWCPv_Y AI動画のみVer ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/OVpYBxK0sCg/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/OVpYBxK0sCg/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/OVpYBxK0sCg/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2022-10-15T14:25:11Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "v8Rj7aklm3y-C-9ffnmdLzf2V08",
"id": {
"kind": "youtube#video",
"videoId": "c-rTb9e13eE"
},
"snippet": {
"publishedAt": "2022-05-14T14:36:37Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "自宅で巨大ドローンモータ出力試験してみた① #Shorts",
"description": "回転数計回ってなかった。。 バッテリーは12S Lipo 自宅でのフルパワー試験は、危険。。 チャンネル <登録よろしく!",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/c-rTb9e13eE/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/c-rTb9e13eE/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/c-rTb9e13eE/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2022-05-14T14:36:37Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "59_SKVCBGAGi6lYcAxdsc6KoseI",
"id": {
"kind": "youtube#video",
"videoId": "tqRip7ifALk"
},
"snippet": {
"publishedAt": "2022-10-15T14:46:41Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "流行りのAIにミクさんのTell Your World食べさせてみた",
"description": "素晴らしい元動画様! https://www.youtube.com/watch?v=UUaVUWCPv_Y&t=0s 流行りの #novelai に動画を食わせるとどうなる ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/tqRip7ifALk/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/tqRip7ifALk/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/tqRip7ifALk/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2022-10-15T14:46:41Z"
}
}
]
}
2B.検索を日付順でソートする
レスポンス関数については、ワード検索時と同一であるが、本検索においては、順番が「検索クエリとの関連性順」となるため、作成日順にする場合は、orderを設定する必要がある。
order = "date"
にて、レスポンス結果は、
{
"kind": "youtube#searchListResponse",
"etag": "sQhGQ9AAbO6jaHWyIrJ4QqGttHc",
"nextPageToken": "CAMQAA",
"regionCode": "JP",
"pageInfo": {
"totalResults": 73,
"resultsPerPage": 3
},
"items": [
{
"kind": "youtube#searchResult",
"etag": "WOcgFiojArc-fxSUeeqGbTsGwLY",
"id": {
"kind": "youtube#video",
"videoId": "9mvsLb70C1Q"
},
"snippet": {
"publishedAt": "2024-01-09T06:11:23Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "AIのみ配信の後日談",
"description": "チャンネル 登録よろしく! メガゴリラ Twitter https://twitter.com/Mega_Gorilla_.",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-09T06:11:23Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "hV27a_brPZ7sXQohp67RmzkmPFo",
"id": {
"kind": "youtube#video",
"videoId": "gmAZ9yZM-kI"
},
"snippet": {
"publishedAt": "2024-01-09T04:46:39Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "AIが入部する?!【ドキドキ文芸部!! #3】 #AITuber #新人Vtuber #Vtuber #chatgpt #ai",
"description": "AI Tuber 未来アイリとは?  ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ 猩々博士によって、開発され ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-09T04:46:39Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "XRNd3TX0ZDorikM3A8tzUzeSx3Q",
"id": {
"kind": "youtube#video",
"videoId": "oPPNoCi77F8"
},
"snippet": {
"publishedAt": "2024-01-05T05:00:24Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "新年なので、コーヒーマシーンを大掃除してみた【作業音ASMR】#shorts",
"description": "チャンネル 登録よろしく! メガゴリラ Twitter https://twitter.com/Mega_Gorilla_.",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/oPPNoCi77F8/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/oPPNoCi77F8/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/oPPNoCi77F8/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-05T05:00:24Z"
}
}
]
}
2C.orderソート方法一覧
orderソートには、以下の関数がある
rating - リソースは、評価の高い順に並べ替えられています。
relevance – 検索クエリとの関連性に基づいてリソースが並べ替えられます。このパラメータのデフォルト値です。
title – リソースはタイトルのアルファベット順に並べ替えられます。
videoCount - チャンネルは、アップロードされた動画の数の多い順に並べ替えられています。
viewCount – リソースは、閲覧数の多い順に並べ替えられています。ライブ配信中は、配信中に同時同時視聴者数で並べ替えられます。
date - リソースは、作成日が新しい時系列で並べ替えられます。
2D.配信アーカイブのみ取得したい
配信アーカイブのみ取得したい場合、以下のように設定することで取得可能
type="video"
eventType="completed"
eventType を Completedにすることで、配信アーカイブのみに絞ることが可能となる。
レスポンス:
{
"kind": "youtube#searchListResponse",
"etag": "mzPaJ8xqn3YAk6emHoLOiyMtTIQ",
"nextPageToken": "CAMQAA",
"regionCode": "JP",
"pageInfo": {
"totalResults": 28,
"resultsPerPage": 3
},
"items": [
{
"kind": "youtube#searchResult",
"etag": "WOcgFiojArc-fxSUeeqGbTsGwLY",
"id": {
"kind": "youtube#video",
"videoId": "9mvsLb70C1Q"
},
"snippet": {
"publishedAt": "2024-01-09T06:11:23Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "AIのみ配信の後日談",
"description": "チャンネル 登録よろしく! メガゴリラ Twitter https://twitter.com/Mega_Gorilla_.",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/9mvsLb70C1Q/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-09T06:11:23Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "hV27a_brPZ7sXQohp67RmzkmPFo",
"id": {
"kind": "youtube#video",
"videoId": "gmAZ9yZM-kI"
},
"snippet": {
"publishedAt": "2024-01-09T04:46:39Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "AIが入部する?!【ドキドキ文芸部!! #3】 #AITuber #新人Vtuber #Vtuber #chatgpt #ai",
"description": "AI Tuber 未来アイリとは?  ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ 猩々博士によって、開発され ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/gmAZ9yZM-kI/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-09T04:46:39Z"
}
},
{
"kind": "youtube#searchResult",
"etag": "ovRJKB1_Bcd4u0EnFUhF7v4lY0U",
"id": {
"kind": "youtube#video",
"videoId": "scQ3edVTEsc"
},
"snippet": {
"publishedAt": "2024-01-02T17:28:43Z",
"channelId": "UCxyEJ2PblLJDPxOkN_6ALLw",
"title": "AIと入部する?!【ドキドキ文芸部!! #2】#AITuber #新人Vtuber #Vtuber #chatgpt #ai",
"description": "AI Tuber 未来アイリとは?  ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ 猩々博士によって、開発され ...",
"thumbnails": {
"default": {
"url": "https://i.ytimg.com/vi/scQ3edVTEsc/default.jpg",
"width": 120,
"height": 90
},
"medium": {
"url": "https://i.ytimg.com/vi/scQ3edVTEsc/mqdefault.jpg",
"width": 320,
"height": 180
},
"high": {
"url": "https://i.ytimg.com/vi/scQ3edVTEsc/hqdefault.jpg",
"width": 480,
"height": 360
}
},
"channelTitle": "未来 アイリ + ハカセ",
"liveBroadcastContent": "none",
"publishTime": "2024-01-02T17:28:43Z"
}
}
]
}
3.検索結果を日本のみに絞りたい(Search: list)
Youtube Data APIには、検索にて国名でフィルタリングする方法について、関数が、
regionCode
relevanceLanguage
の2つが存在するが、
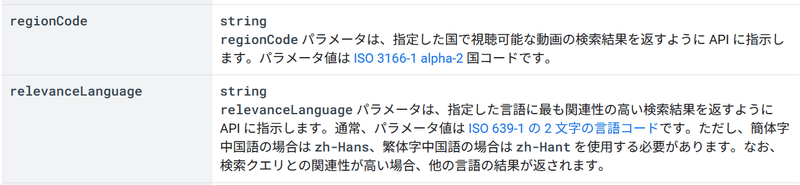
画像の説明の通り、regionCodeは、その国で見れるか、見れないかをフィルタするパラメータであるため、JPを指定しても、日本から視聴可能なコンテンツすべてが表示されてしまう。そのため、日本語コンテンツを表示したい場合は、relevanceLanguageを用いる必要がある。
また関数に入れるStringについても、regionCodeは、ISO 3166-1 alpha-2を用いるのに対して、relevanceLanguageについてはISO 639-1 の 2 文字の言語コードを用いる必要がある。
そのためregionCodeの場合は"JP"を、relevanceLanguageの場合は'ja'を使う必要があり注意が必要だ。
4.チャンネル情報を取得する(Channels: list)
チャンネルIDよりチャンネル情報を取得します。
コード:
# -*- coding: utf-8 -*-
# Sample Python code for youtube.channels.list
# See instructions for running these code samples locally:
# https://developers.google.com/explorer-help/code-samples#python
import os
import google_auth_oauthlib.flow
import googleapiclient.discovery
import googleapiclient.errors
scopes = ["https://www.googleapis.com/auth/youtube.readonly"]
def main():
# Disable OAuthlib's HTTPS verification when running locally.
# *DO NOT* leave this option enabled in production.
os.environ["OAUTHLIB_INSECURE_TRANSPORT"] = "1"
api_service_name = "youtube"
api_version = "v3"
client_secrets_file = "YOUR_CLIENT_SECRET_FILE.json"
# Get credentials and create an API client
flow = google_auth_oauthlib.flow.InstalledAppFlow.from_client_secrets_file(
client_secrets_file, scopes)
credentials = flow.run_console()
youtube = googleapiclient.discovery.build(
api_service_name, api_version, credentials=credentials)
request = youtube.channels().list(
part="statistics",
id="UCxyEJ2PblLJDPxOkN_6ALLw"
)
response = request.execute()
print(response)
if __name__ == "__main__":
main()
レスポンス(statistics):
{
"kind": "youtube#channelListResponse",
"etag": "0F8_X6UFFlRL6jR-xV1LvpSiQ5k",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "b_2Z4H609nkwIKyzUSug99cgC4g",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"statistics": {
"viewCount": "2542233",
"subscriberCount": "4480",
"hiddenSubscriberCount": false,
"videoCount": "72"
}
}
]
}
上記コードでは、partを「statistics」にて設定しております。
Statisticsは「チャンネルの統計情報、例えば登録者数、ビデオの総視聴回数、ビデオの数など」を提供し、Channels.listはstatisticsのほかに、以下のパラメータがあります。
auditDetails: チャンネルがコミュニティガイドラインや著作権に違反していないかの監査情報を提供します。
brandingSettings: チャンネルのブランディングに関する設定、例えばチャンネルのタイトル、説明、アイコン、バナー画像などの情報を提供します。
contentDetails: チャンネルのコンテンツに関する詳細情報、例えばアップロードされた動画のリストやプレイリスト情報などを提供します。
contentOwnerDetails: チャンネルのコンテンツオーナーに関する情報を提供します。主にYouTubeコンテンツパートナーに関連します。
id: チャンネルのID情報を提供します。
localizations: チャンネルに設定された異なる言語のローカライズされた情報を提供します。
snippet: チャンネルに関する基本情報、例えばチャンネル名、説明、作成日、サムネイルなどを提供します。
statistics: チャンネルの統計情報、例えば登録者数、ビデオの総視聴回数、ビデオの数などを提供します。
status: チャンネルのステータスに関する情報、例えばチャンネルが非公開かどうか、コンテンツIDの状態などを提供します。
topicDetails: チャンネルに関連するトピック情報、例えばFreebaseのトピックIDなどを提供します。これにより、チャンネルがどのようなトピックに関連しているかがわかります。
他のパラメータについてもいくつか、レスポンスを示します。
レスポンス(brandingSettings)
{
"kind": "youtube#channelListResponse",
"etag": "dk5v8Kr472nbvFx9Qr8-Swq2gvU",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "BmWyJSGh2ao8XCI2aaXswYF9nVM",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"brandingSettings": {
"channel": {
"title": "未来 アイリ + ハカセ",
"description": "AITuber みらいちゃんと、その開発者であるゴリラの日常を描くチャンネルです。",
"defaultLanguage": "ja",
"country": "JP"
}
}
}
]
}
レスポンス(contentDetails)
{
"kind": "youtube#channelListResponse",
"etag": "Qg7RrgDaN4WW0cDbNsoMpjgLw1M",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "_ejHE0oxw8KlI0hQ-VYiXFVTOyM",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"contentDetails": {
"relatedPlaylists": {
"likes": "",
"uploads": "UUxyEJ2PblLJDPxOkN_6ALLw"
}
}
}
]
}
レスポンス(id)
{
"kind": "youtube#channelListResponse",
"etag": "Z0SykH_Ai7TfZ1LqVpHT_nHJJKQ",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "PE5d1_kORkulh3uOLHSZczvzGnA",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw"
}
]
}
レスポンス(localizations)
{
"kind": "youtube#channelListResponse",
"etag": "3heSwE13f2KzEth-oj8upzDWJ8c",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "jUCU0R0NuoQnWtjAoTNgxeae_nA",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"localizations": {
"ja_JP": {
"title": "未来 アイリ + ハカセ",
"description": "AITuber みらいちゃんと、その開発者であるゴリラの日常を描くチャンネルです。"
},
"en_US": {
"title": "AI[Mirai]&Gorilla",
"description": "This is a channel about the daily life of AITuber Mirai and her developer, Gorilla."
}
}
}
]
}
レスポンス(snippet)
{
"kind": "youtube#channelListResponse",
"etag": "E7W39UokCJVWwqY8jhgnjCgy--I",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "v-ccH3pgM_FqnZdyGheIz9OUaJE",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"snippet": {
"title": "未来 アイリ + ハカセ",
"description": "AITuber みらいちゃんと、その開発者であるゴリラの日常を描くチャンネルです。",
"customUrl": "@hakase_ai_channel",
"publishedAt": "2019-04-12T05:28:05Z",
"thumbnails": {
"default": {
"url": "https://yt3.ggpht.com/Pvxw19ieFse_9fFXbVcoq8oHgRt3PuVuPvlS5A6sHNzQw9F9uSorzMpnPNXDdsZNiWIJFuGDXw=s88-c-k-c0x00ffffff-no-rj",
"width": 88,
"height": 88
},
"medium": {
"url": "https://yt3.ggpht.com/Pvxw19ieFse_9fFXbVcoq8oHgRt3PuVuPvlS5A6sHNzQw9F9uSorzMpnPNXDdsZNiWIJFuGDXw=s240-c-k-c0x00ffffff-no-rj",
"width": 240,
"height": 240
},
"high": {
"url": "https://yt3.ggpht.com/Pvxw19ieFse_9fFXbVcoq8oHgRt3PuVuPvlS5A6sHNzQw9F9uSorzMpnPNXDdsZNiWIJFuGDXw=s800-c-k-c0x00ffffff-no-rj",
"width": 800,
"height": 800
}
},
"defaultLanguage": "ja",
"localized": {
"title": "未来 アイリ + ハカセ",
"description": "AITuber みらいちゃんと、その開発者であるゴリラの日常を描くチャンネルです。"
},
"country": "JP"
}
}
]
}
レスポンス(status)
{
"kind": "youtube#channelListResponse",
"etag": "cXvXnZGtvJ3AnMWsT8bzgOMldz8",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "6V4tqoWNqOs1YjZGBHEX16wI6bA",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"status": {
"privacyStatus": "public",
"isLinked": true,
"longUploadsStatus": "longUploadsUnspecified",
"madeForKids": false
}
}
]
}
レスポンス(topicDetails)
{
"kind": "youtube#channelListResponse",
"etag": "oAySDBM_XKk1P0HnZbOSQhVzltk",
"pageInfo": {
"totalResults": 1,
"resultsPerPage": 5
},
"items": [
{
"kind": "youtube#channel",
"etag": "4SQUE18JOuF_QoeSG1nlgqDjjPY",
"id": "UCxyEJ2PblLJDPxOkN_6ALLw",
"topicDetails": {
"topicIds": [
"/m/02vxn",
"/m/0bzvm2",
"/m/02jjt"
],
"topicCategories": [
"https://en.wikipedia.org/wiki/Film",
"https://en.wikipedia.org/wiki/Video_game_culture",
"https://en.wikipedia.org/wiki/Entertainment"
]
}
}
]
}
詳細については以下を参考にしてください。
5.YouTube Data APIのQuotaコストについて
Youtube Data APIは、Quotaという割り当て費用が設定されており、クオーター制限は1日あたり、10,000クオーターとなっている。一見10,000リクエスト送れるなんて十分じゃん!と思うかもしれないが、これには罠があり、1リクエスト1クオーターではない。
クオーターの計算方法は以下のリンクを参考
簡単に言うと、今回のサンプルで用いた「snippet」を用いると1リクエストあたり100クオーターとなります。つまり、1つの動画あたり、2クオーターのコストが発生します。
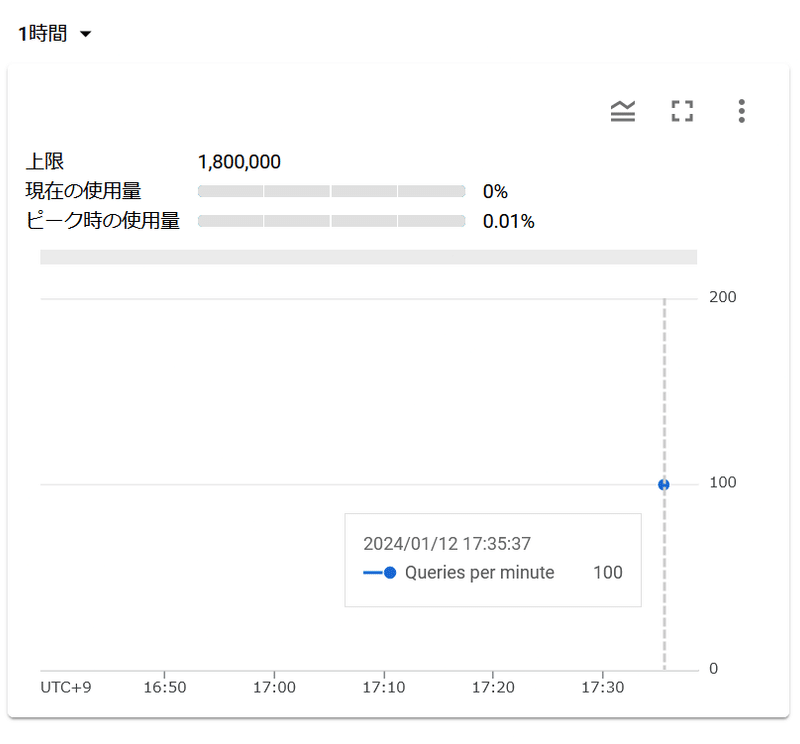
そのため、snippetで問い合わせを行うと、最大で1日あたり、5000件までしか取得できません。しかし、Channels: listにてチャンネル情報を参照した場合、クオーターコストは「1」と低コストです。そのため、snippetは必要な時のみ使うことをお勧めします。
参考:
この記事が気に入ったらサポートをしてみませんか?