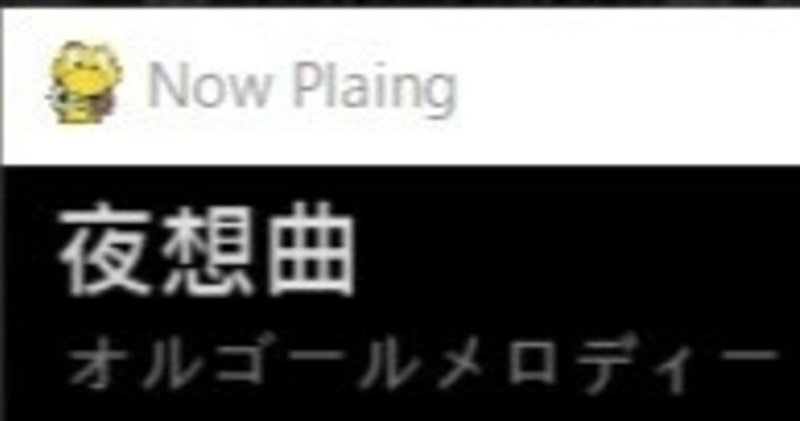
Volumio で再生中の曲を表示する Windows 10 のアプリ
ラズパイで動作している Volumio 3 で再生中の曲名とアーティスト名をシンプルに表示する Windows 10 のアプリです。 Python 3 + pygame で動作します。
Volumio は同じネットワーク内にあり、volumio.local でアクセス可能であることが前提です。(ホスト名を変更または名前解決できない場合は base_url を適宜変更することで対応可能)
Code
import sys
import requests
import time
import pygame
from pygame.locals import *
# 設定
FONT = "C:\Windows\Fonts\msjhbd.ttc"
# pygame 初期設定
pygame.init()
screen = pygame.display.set_mode((400, 50))
pygame.display.set_caption("Now Plaing")
font1 = pygame.font.Font(FONT, 20)
font2 = pygame.font.Font(FONT, 14)
# Volumioで再生中の情報を取得
def volumio_nowplaying():
base_url = 'http://volumio.local/api/v1/getState'
url = f'{base_url}'
r = requests.get(url)
print(url, r)
if r.status_code != 200:
return None
return r.json()
def main():
# 曲名とアーティストを描画
def draw_song_data():
text = font1.render(song_title, True, (220, 220, 220))
screen.blit(text, [10, 2])
text = font2.render(song_artist, True, (120, 120, 120))
screen.blit(text, [12, 27])
last_song_title = None # 1つ前の再生曲名
get_count = 0 # 曲を取得する時間のカウンタ
while True:
# イベントを処理
for event in pygame.event.get():
if event.type == QUIT: # 閉じるボタンが押されたら終了
pygame.quit()
sys.exit()
# 再生中の曲を取得 (Volumioの負荷を考慮して5秒に1回)
if get_count == 0:
r = volumio_nowplaying()
song_title = r["title"]
song_artist = r["artist"]
get_count = 50 # 50 x 0.1 sec = 5秒
else:
get_count = get_count - 1
# 曲が変わった時だけ画面更新
if last_song_title != song_title:
screen.fill((0, 0 ,0))
draw_song_data()
pygame.display.update()
last_song_title = song_title
time.sleep(0.1)
if __name__ == '__main__':
main()
コンソール画面を非表示にする
通常の Python ファイル名の拡張子は .py ですが .pyw にすることでコンソール画面 (cmd.exe) を非表示にすることができます。
この記事が気に入ったらサポートをしてみませんか?