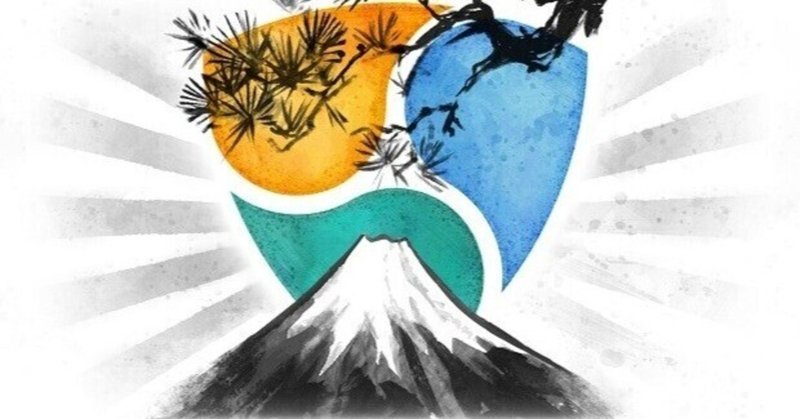
【Symbol Blog】NEM PYTHON SDK: トランザクション初心者ガイド
この記事は2023年1月24日にSymbol Blogにて投稿された記事「18 JAN SYMBOL NEM SDK: A BEGINNERS GUIDE TO TRANSACTIONS」をChatGPTを用いて翻訳されたものです。
Following on from the last post about creating Symbol transactions using the Python SDK, here's a very short post showing how NEM transfers, namespaces and mosaics can be written.https://t.co/RDzBvn54Y3#NEM #Symbol #Python
— Symbol Blog @symbolblog.symbol 🏴☠️ (@blog_symbol) January 24, 2024
前回のポストでPythonでSymbolトランザクションを書くことについて書きましたが、その続きとして、Python SDKを使用してNEMトランザクションを送信する方法を示す簡単なポストがあります。すべてのトランザクションタイプを網羅していませんが、これは始めるためのアイデアを提供するはずです。いつものように、これらの例はすべてテストネットを使用しており、そのためにAliceの秘密鍵も含まれています(自分の秘密鍵は絶対に共有しないでください!)。
注意: これは少し前に書かれたものです。問題がある場合はお知らせください。
TRANSFER TRANSACTION
Python SDKを使用して、NEMネットワーク上でシンプルな送金、メッセージあり・なしの送金も可能です。この例では、Aliceがプレーンなメッセージを添えてBobに1 XEMを送金しています。これは `transfer_transaction_v1` を使用しており、デフォルトで送信されるモザイクは nem.xem に設定されています。資金を送金するには amount フィールドのみを指定する必要があります。
TRANSACTION
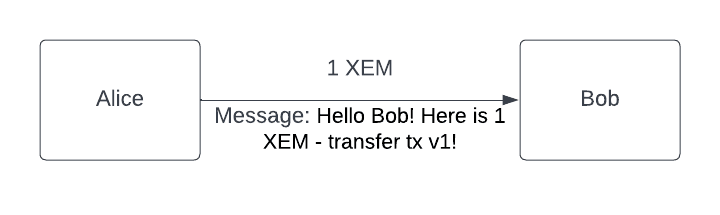
CODE
from symbolchain.nem.KeyPair import KeyPair
from symbolchain.facade.NemFacade import NemFacade
from symbolchain.CryptoTypes import PrivateKey
from symbolchain.nem.Network import NetworkTimestamp
from binascii import unhexlify, hexlify
import requests
import datetime
import json
facade = NemFacade('testnet')
# Replace with your private key
alicePrivateKey = PrivateKey(unhexlify('9A243BD88D1F3EA4B46264EF0505EFE102A46E58E4EC100407DA5F5AAD08BC0A'))
# Replace with the recipient address
bobAddress = 'TBNEN3NVDMKRDVMLIVDVAS4SLZMJRIBWFWOCERZV'
# Replace with node URL and connection port
node_url = 'http://95.216.73.245:7890'
# Set URLs for obtaining network time and to post transactions to
post_string = node_url + '/transaction/announce'
time_string = node_url + '/time-sync/network-time'
# Obtain network time
networkTimeRequest = requests.get(time_string)
# Convert from milliseconds to seconds
networkTime = NetworkTimestamp(int(networkTimeRequest.json()['sendTimeStamp'] / 1000))
# Set the deadline to 1 hour in the future
deadline = networkTime.add_hours(1).timestamp
aliceKeypair = NemFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Create a transfer transaction
transfer = facade.transaction_factory.create({
'type': 'transfer_transaction_v1',
'signer_public_key': alicePubkey,
'recipient_address': bobAddress, # Send to Bob
'deadline': deadline,
'message': {
'message_type': 'plain', # Plain message type
'message': 'Hello Bob! Here is 1 XEM - transfer tx v1!' #message
},
'amount': 1000000, # Send 1 XEM
'fee': 200000, # Add a 0.2 XEM fee
'timestamp': networkTime.timestamp # Add network time stamp
})
# Sign the transaction and attach the signature
signature = facade.sign_transaction(aliceKeypair, transfer)
tx = facade.transaction_factory.attach_signature(transfer, signature)
# Announce the transaction to the network
r = requests.post(post_string, json=json.loads(tx))
print(f"Status Code: {r.status_code}, Response: {r.json()}")
TRANSFER TRANSACTION (V2)
`transfer_transaction_v2` を使用すると、トランザクションで送信するカスタムモザイクを設定できます。この例では、Aliceは関連するメッセージを添えて、1 nem.xem と 1 test1.testmosaic をBobに送金しています。
TRANSACTION
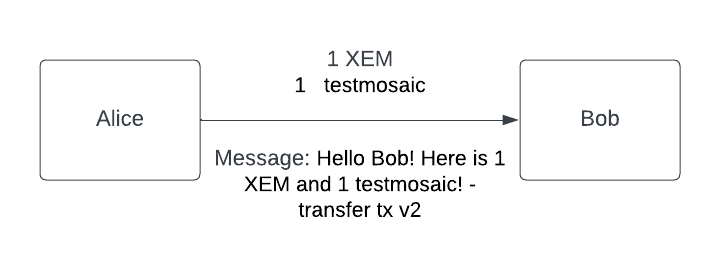
CODE
from symbolchain.nem.KeyPair import KeyPair
from symbolchain.facade.NemFacade import NemFacade
from symbolchain.CryptoTypes import PrivateKey
from symbolchain.nem.Network import NetworkTimestamp
from binascii import unhexlify, hexlify
import requests
import datetime
import json
facade = NemFacade('testnet')
# Replace with your private key
alicePrivateKey = PrivateKey(unhexlify('9A243BD88D1F3EA4B46264EF0505EFE102A46E58E4EC100407DA5F5AAD08BC0A'))
# Replace with the recipient address
bobAddress = 'TBNEN3NVDMKRDVMLIVDVAS4SLZMJRIBWFWOCERZV'
# Replace with node URL and connection port
node_url = 'http://95.216.73.245:7890'
# Set URLs for obtaining network time and to post transactions to
post_string = node_url + '/transaction/announce'
time_string = node_url + '/time-sync/network-time'
# Obtain network time
networkTimeRequest = requests.get(time_string)
# Convert from milliseconds to seconds
networkTime = NetworkTimestamp(int(networkTimeRequest.json()['sendTimeStamp'] / 1000))
# Set the deadline to 1 hour in the future
deadline = networkTime.add_hours(1).timestamp
aliceKeypair = NemFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Create a transfer transaction. version 2
transfer = facade.transaction_factory.create({
'type': 'transfer_transaction_v2',
'signer_public_key': alicePubkey,
'recipient_address': bobAddress, # Bob's address
'deadline': deadline,
'message': {
'message_type': 'plain', # Plain message
'message': 'Hello Bob! Here is 1 XEM and 1 testmosaic! - transfer tx v2'
},
# Set mosaics to send
'mosaics': [{'mosaic':
# Send 1 XEM
{'mosaic_id': {'namespace_id': {'name': b'nem'}, 'name': b'xem'},
'amount': 1000000}
},
# Send 10 "testmosaic" mosaics linked to the namespace "test1"
{'mosaic':
{'mosaic_id': {'namespace_id': {'name': b'test1'}, 'name': b'testmosaic'},
'amount': 1}
}
],
'fee': 200000, # Add a 0.2 XEM fee
'timestamp': networkTime.timestamp # Add network timestamp
})
# Sign the transaction and attach signature
signature = facade.sign_transaction(aliceKeypair, transfer)
tx = facade.transaction_factory.attach_signature(transfer, signature)
# Announce the transaction to the network
r = requests.post(post_string, json=json.loads(tx))
print(f"Status Code: {r.status_code}, Response: {r.json()}")
ネームスペース定義
NEMのネームスペースは、1年間の固定期間の賃料が100 XEMで借りることができ、期限切れ前に更新することができます。以下のトランザクションでは、Aliceが新しいネームスペース "newnamespace" を登録し、テストネットの TAMESPACEWH4MKFMBCVFERDPOOP4FK7MTDJEYP35 に対してネームスペースの賃料を送金しています。
CODE
from symbolchain.nem.KeyPair import KeyPair
from symbolchain.facade.NemFacade import NemFacade
from symbolchain.CryptoTypes import PrivateKey
from symbolchain.nem.Network import NetworkTimestamp
from binascii import unhexlify, hexlify
import requests
import datetime
import json
facade = NemFacade('testnet')
# Replace with your private key
alicePrivateKey = PrivateKey(unhexlify('9A243BD88D1F3EA4B46264EF0505EFE102A46E58E4EC100407DA5F5AAD08BC0A'))
# Replace with the recipient address
bobAddress = 'TBNEN3NVDMKRDVMLIVDVAS4SLZMJRIBWFWOCERZV'
# Replace with node URL and connection port
node_url = 'http://95.216.73.245:7890'
# Set URLs for obtaining network time and to post transactions to
post_string = node_url + '/transaction/announce'
time_string = node_url + '/time-sync/network-time'
# Obtain network time
networkTimeRequest = requests.get(time_string)
# Convert from milliseconds to seconds
networkTime = NetworkTimestamp(int(networkTimeRequest.json()['sendTimeStamp'] / 1000))
# Set the deadline to 1 hour in the future
deadline = networkTime.add_hours(1).timestamp
aliceKeypair = NemFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Create a namespace transaction
namespace_def = facade.transaction_factory.create({
'type': 'namespace_registration_transaction_v1',
'signer_public_key': alicePubkey,
'deadline': deadline,
'rental_fee': 100000000, # Set 100 XEM rental fee
'fee': 200000, # 0.2 XEM fee for the transaction
'name': 'newnamespace', # Name the namespace "newnamespace"
# Set the namespace rental fee sink address (this is the address for testnet)
'rental_fee_sink': 'TAMESPACEWH4MKFMBCVFERDPOOP4FK7MTDJEYP35',
'timestamp': networkTime.timestamp # Add timestamp
})
# Sign the transaction and attach signature
signature = facade.sign_transaction(aliceKeypair, namespace_def)
tx = facade.transaction_factory.attach_signature(namespace_def, signature)
# Announce the transaction to the network
r = requests.post(post_string, json=json.loads(tx))
print(f"Status Code: {r.status_code}, Response: {r.json()}")
モザイク定義
Symbolと同様に、NEMモザイクも作成できます。ただし、NEMモザイクは登録時にネームスペースと関連付ける必要があり、そのため親のネームスペースが更新されない限り期限が切れます。以下の例では、Aliceがテストネットモザイクのシンクアドレス TBMOSAICOD4F54EE5CDMR23CCBGOAM2XSJBR5OLC に10 XEMの固定料金を支払います。彼女は新しいモザイク "awesome_mosaic" を作成し、それを上記で作成した "newnamespace" と関連付けます。これにより、モザイクは newnamespace.awesome_mosaic となります。
CODE
from symbolchain.nem.KeyPair import KeyPair
from symbolchain.facade.NemFacade import NemFacade
from symbolchain.CryptoTypes import PrivateKey
from symbolchain.nem.Network import NetworkTimestamp
from binascii import unhexlify, hexlify
import requests
import datetime
import json
facade = NemFacade('testnet')
# Replace with your private key
alicePrivateKey = PrivateKey(unhexlify('9A243BD88D1F3EA4B46264EF0505EFE102A46E58E4EC100407DA5F5AAD08BC0A'))
# Replace with the recipient address
bobAddress = 'TBNEN3NVDMKRDVMLIVDVAS4SLZMJRIBWFWOCERZV'
# Replace with node URL and connection port
node_url = 'http://95.216.73.245:7890'
# Set URLs for obtaining network time and to post transactions to
post_string = node_url + '/transaction/announce'
time_string = node_url + '/time-sync/network-time'
# Obtain network time
networkTimeRequest = requests.get(time_string)
# Convert from milliseconds to seconds
networkTime = NetworkTimestamp(int(networkTimeRequest.json()['sendTimeStamp'] / 1000))
# Set the deadline to 1 hour in the future
deadline = networkTime.add_hours(1).timestamp
aliceKeypair = NemFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Create a NEM mosaic transaction
nem_mosaic = facade.transaction_factory.create({
'type': 'mosaic_definition_transaction_v1',
'signer_public_key': alicePubkey,
'deadline': deadline,
'rental_fee': 10000000, # Add 10 XEM rental fee
'fee': 200000, # Add 0.2 XEM transaction fee
# Set the mosaic sink address for testnet
'rental_fee_sink': 'TBMOSAICOD4F54EE5CDMR23CCBGOAM2XSJBR5OLC',
'timestamp': networkTime.timestamp, # Add timestamp
'mosaic_definition': {
'owner_public_key': alicePubkey,
# Create the mosaic "awesome mosaic" and assign this to "newnamespace"
'id': {'namespace_id': {'name': b'newnamespace'}, 'name': b'awesome_mosaic'},
# Add a mosaic description
'description': b'Just testing',
# Set mosaic properties:
# Divisibility = 0
# Initial supply = 1000
# Supply mutable = True
# Transferable = True
'properties': [
{'property_': {'name': b'divisibility', 'value': b'0'}},
{'property_': {'name': b'initialSupply', 'value': b'1000'}},
{'property_': {'name': b'supplyMutable', 'value': b'true'}},
{'property_': {'name': b'transferable', 'value': b'true'}}
]
}
})
# Sign the transaction and attach signature
signature = facade.sign_transaction(aliceKeypair, nem_mosaic)
tx = facade.transaction_factory.attach_signature(nem_mosaic, signature)
# Announce the transaction to the network
r = requests.post(post_string, json=json.loads(tx))
print(f"Status Code: {r.status_code}, Response: {r.json()}")
MOSAIC SUPPLY CHANGE
モザイクが可変として作成された場合、所有者はモザイクの供給を変更できます。以下の例では、Aliceが "newnamespace.awesome_mosaic" の供給を100ユニット増やしています。先に述べたように、デルタ値を設定する前に、モザイクの分割可能性を確認することを忘れないでください。この場合、モザイクの分割可能性は0なので、100の新しいトークンが作成されました。供給は、アクション値を減少に設定することで減少させることができます。
CODE
from symbolchain.nem.KeyPair import KeyPair
from symbolchain.facade.NemFacade import NemFacade
from symbolchain.CryptoTypes import PrivateKey
from symbolchain.nem.Network import NetworkTimestamp
from binascii import unhexlify, hexlify
import requests
import datetime
import json
facade = NemFacade('testnet')
# Replace with your private key
alicePrivateKey = PrivateKey(unhexlify('9A243BD88D1F3EA4B46264EF0505EFE102A46E58E4EC100407DA5F5AAD08BC0A'))
# Replace with the recipient address
bobAddress = 'TBNEN3NVDMKRDVMLIVDVAS4SLZMJRIBWFWOCERZV'
# Replace with node URL and connection port
node_url = 'http://95.216.73.245:7890'
# Set URLs for obtaining network time and to post transactions to
post_string = node_url + '/transaction/announce'
time_string = node_url + '/time-sync/network-time'
# Obtain network time
networkTimeRequest = requests.get(time_string)
# Convert from milliseconds to seconds
networkTime = NetworkTimestamp(int(networkTimeRequest.json()['sendTimeStamp'] / 1000))
# Set the deadline to 1 hour in the future
deadline = networkTime.add_hours(1).timestamp
aliceKeypair = NemFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Create a NEM mosaic modification transaction
nem_mosaic_mod = facade.transaction_factory.create({
'type': 'mosaic_supply_change_transaction_v1',
'signer_public_key': alicePubkey,
'deadline': deadline,
'fee': 200000, # Transaction fee
'timestamp': networkTime.timestamp, # Network timestamp
'mosaic_id': {
# Specify the mosaic name to be modified and the namespace that it is
# associated with
'namespace_id': {'name': b'newnamespace'},
'name': b'super_awesome_mosaic'
},
'action': 'increase', # Increase the number of units (can also be decreased)
'delta': 100 # Number of units to add/remove
})
# Sign the transaction and attach signature
signature = facade.sign_transaction(aliceKeypair, nem_mosaic_mod)
tx = facade.transaction_factory.attach_signature(nem_mosaic_mod, signature)
# Announce the transaction to the network
r = requests.post(post_string, json=json.loads(tx))
print(f"Status Code: {r.status_code}, Response: {r.json()}")
この記事が気に入ったらサポートをしてみませんか?