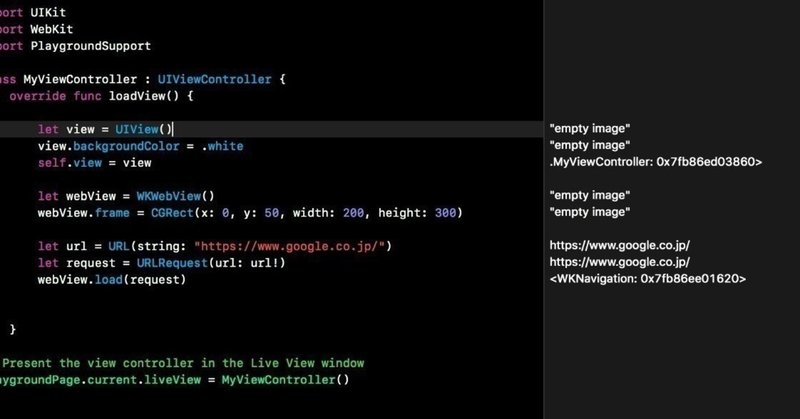
Swiftで行こう!--ちょっと書き。
アルゴニズム、便利なスニペットがまとめてありますので、ちょっと書きながら考えてみます。
まずバブルソートです。
func bubbleSort(_ inputArr:[Int]) -> [Int] {
guard inputArr.count > 1 else {
return inputArr
}
var res = inputArr
let count = res.count
var isSwapped = false
repeat {
isSwapped = false
for index in stride(from: 1, to: count, by: 1) {
if res[index] < res[index - 1] {
res.swapAt((index - 1), index)
isSwapped = true
}
}
} while isSwapped
return res
}
( _ inputArr:[Int])
引数ですが、"_"をつけることで、実際使う時に
bubbleSort(引数)
のように使えるようになります。ちなみに通常は( )ないは
bubbleSort(ラベル:引数)
という感じになります。
( _ inputArr:[Int])
次には引数の型ですが":[Int])"ということで[ ]は配列を意味し、Intということで整数が入ることを示しています。
次に
guard inputArr.count > 1 else {
return inputArr
}
guardの文ですね。 inputArr.count > 1であれば、次に進む具体的には、var res 以下を実行します。そうでなければreturn inputArrということで、終了します。
次にrepeat whileの文ですね。
repeat {
isSwapped = false
for index in stride(from: 1, to: count, by: 1) {
if res[index] < res[index - 1] {
res.swapAt((index - 1), index)
isSwapped = true
}
}
} while isSwapped
構造ですが、repeat whileに入り、 for in文で数字を取り出しif文で条件分岐指定き条件に合うものはswapAtで入れ替えるものです。あとfor in文で取り出す数字をstride(from,to,by)で指定指定ます。
具体的には、for in 文で、stride(from: 1, to: count, by: 1)を使い1から変数countまで1づつ数値を変える、indexに順番に1から変数countに入る数字まで入れていきます。
そしてif 文ですね。
if res[index] < res[index - 1] {
res.swapAt((index - 1), index)
isSwapped = true
}
最初はres[index]ということでres[1]が入り、res[index - 1]はres[0]が入ります。そこで、res[index - 1]の方が大きければ数字を入れ替えます。入れ替えは、
res.swapAt((index - 1), index)
ですね、swapAt関数を使っていますね。この操作を
isSwapped = false になるまで繰り返します。
var a = [4,1,9,7,3,2]
としてソートしてみます。
bubbleSort(a)とすると、
[1, 2, 3, 4, 7, 9]と表示されます。
この記事が気に入ったらサポートをしてみませんか?