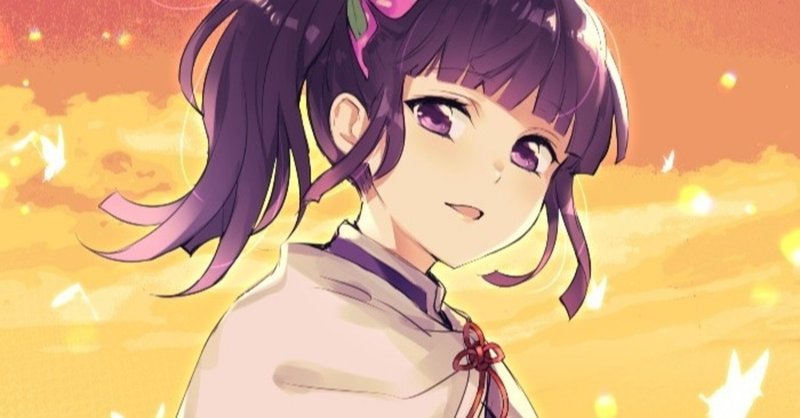
Binance先物 API 入門編
モチベーション
今までずっとBitcoinのリサーチをしてきましたが、ドローダウンはあるもののそこそこ落ち着いてきたので、他のシンボルの予測にも挑戦しようと思います。
そこで、界隈でBinanceが何やら騒がしいので、Binanceを調査してみようと思いました。
メモ用にご活用くださいませ。
準備
もし口座を持っていらっしゃらない方は、こちらから口座開設出来ます。
・Binanceの口座開設(自動的に現物口座になります。)
・Binanceの先物口座開設
・BinanceのAPI Listで新規作成
・Enable Futuresにチェック
あとは、Bitflyerと同じように証拠金を移したりする感じで、Binanceも先物の取引が開始出来ます。
API編
今回もお馴染みのccxtを使います。
公式APIドキュメントはこちら。
バージョン
ccxt.__version__
"""
'1.32.39'
"""
インスタンスを呼ぶ
import ccxt
binance = ccxt.binance(
{
"apiKey": "xxx",
"secret": "xxx",
"options": {"defaultType": "future"},
"enableRateLimit": True,
}
)
残高取得
binance.fapiPrivate_get_balance()
'''
[{'accountAlias': 'xxx',
'asset': 'USDT',
'balance': '26.88180000',
'withdrawAvailable': '26.77975919',
'updateTime': 0},
{'accountAlias': 'xxx',
'asset': 'BNB',
'balance': '3',
'withdrawAvailable': '3.00000000',
'updateTime': 1600571074178}]
'''
私は、現物口座から3BNB(手数料割引のため)と26.8818USDT移行していたため、ちゃんと取得出来ていますね。
全てのオーダーを取得
binance.fapiPrivate_get_allorders()
'''
[{'orderId': 46582703,
'symbol': 'UNIUSDT',
'status': 'NEW',
'clientOrderId': 'web_UZylosWSQIwy0pKiPwIc',
'price': '5',
'avgPrice': '0.0000',
'origQty': '1',
'executedQty': '0',
'cumQuote': '0',
'timeInForce': 'GTC',
'type': 'LIMIT',
'reduceOnly': False,
'closePosition': False,
'side': 'BUY',
'positionSide': 'BOTH',
'stopPrice': '0',
'workingType': 'CONTRACT_PRICE',
'priceProtect': 'false',
'origType': 'LIMIT',
'time': 1600571078042,
'updateTime': 1600571078042},
{'orderId': 258717782,
'symbol': 'IOSTUSDT',
'status': 'NEW',
'clientOrderId': 'web_HijtefuApAFH1EzN5Tp3',
'price': '0.005344',
'avgPrice': '0.000000',
'origQty': '1',
'executedQty': '0',
'cumQuote': '0',
'timeInForce': 'GTC',
'type': 'LIMIT',
'reduceOnly': False,
'closePosition': False,
'side': 'BUY',
'positionSide': 'BOTH',
'stopPrice': '0',
'workingType': 'CONTRACT_PRICE',
'priceProtect': 'false',
'origType': 'LIMIT',
'time': 1600572775679,
'updateTime': 1600572775679}]
'''
こちらは、複数シンボルによる異なるオーダーも同時に取得出来ます。
注文中のみのオーダーを取得
binance.fapiPrivate_get_openorders()
"""
[{'orderId': 258740258,
'symbol': 'IOSTUSDT',
'status': 'NEW',
'clientOrderId': '3davh37p3W3rR0xprp7qjy',
'price': '0.005000',
'avgPrice': '0.000000',
'origQty': '1',
'executedQty': '0',
'cumQuote': '0',
'timeInForce': 'GTC',
'type': 'LIMIT',
'reduceOnly': False,
'closePosition': False,
'side': 'BUY',
'positionSide': 'BOTH',
'stopPrice': '0',
'workingType': 'CONTRACT_PRICE',
'priceProtect': 'false',
'origType': 'LIMIT',
'time': 1600573830398,
'updateTime': 1600573886853}]
"""
キャンセル注文
orders = binance.fapiPrivate_get_openorders()
for order in orders:
if order["status"] == "NEW":
print(
binance.fapiPrivate_delete_order(
{"symbol": order["symbol"], "orderId": order["orderId"]}
)
)
"""
{'orderId': 258740258, 'symbol': 'IOSTUSDT', 'status': 'CANCELED', 'clientOrderId': '3davh37p3W3rR0xprp7qjy', 'price': '0.005000', 'avgPrice': '0.000000', 'origQty': '1', 'executedQty': '0', 'cumQty': '0', 'cumQuote': '0', 'timeInForce': 'GTC', 'type': 'LIMIT', 'reduceOnly': False, 'closePosition': False, 'side': 'BUY', 'positionSide': 'BOTH', 'stopPrice': '0', 'workingType': 'CONTRACT_PRICE', 'priceProtect': 'false', 'origType': 'LIMIT', 'updateTime': 1600573963241}
"""
最初のordersは注文中のオーダーを取得しています。今回は注文中のオーダーのみを取得しているので、statusは常にNEWですが、念のため条件づけております。
次に、delete_orderでシンボルと注文IDを指定してあげればキャンセル出来ます。
新規注文
binance.fapiPrivate_post_order(
{
"symbol": "IOSTUSDT",
"side": "BUY",
"quantity": 1,
"type": "LIMIT", # MARKET
"price": 0.005,
"timeInForce": "GTC",
"reduceOnly": False,
}
)
'''
{'orderId': 258730891,
'symbol': 'IOSTUSDT',
'status': 'NEW',
'clientOrderId': 'eDB5H9G3oxGn466Uplw9kF',
'price': '0.005000',
'avgPrice': '0.000000',
'origQty': '1',
'executedQty': '0',
'cumQty': '0',
'cumQuote': '0',
'timeInForce': 'GTC',
'type': 'LIMIT',
'reduceOnly': False,
'closePosition': False,
'side': 'BUY',
'positionSide': 'BOTH',
'stopPrice': '0',
'workingType': 'CONTRACT_PRICE',
'priceProtect': 'false',
'origType': 'LIMIT',
'updateTime': 1600573422540}
'''
ここでは簡単に指値注文だけを紹介します。その他に、トレイリングストップ注文等の特殊注文もあるので、気になる方はこちら[APIドキュメント]をご参考くださいませ。
取引履歴取得
binance.fapiPrivate_get_usertrades()
'''
[{'symbol': 'IOSTUSDT',
'id': 13325056,
'orderId': 258747971,
'side': 'BUY',
'price': '0.006373',
'qty': '1',
'realizedPnl': '0',
'quoteQty': '0.006373',
'commission': '0.00000008',
'commissionAsset': 'BNB',
'time': 1600574190487,
'positionSide': 'BOTH',
'maker': False,
'buyer': True}]
'''
この記事が気に入ったらサポートをしてみませんか?