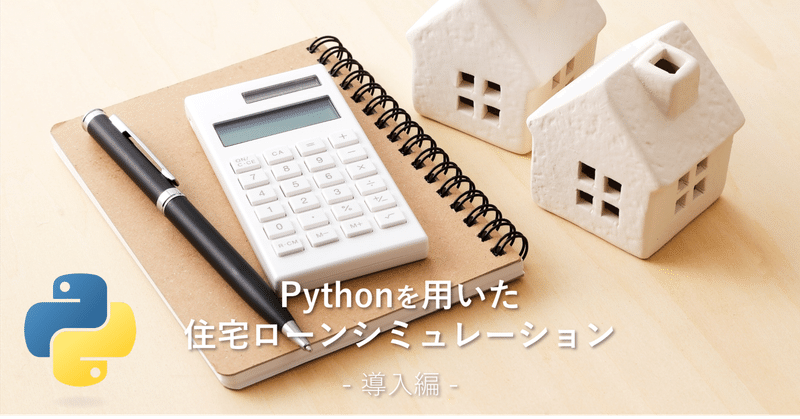
Pythonを用いた住宅ローンシミュレーション(導入編)
初めまして、分析屋のFと申します。
私は5年前、住宅購入を経験致しました。
その際、住宅ローンのシミュレーションについて様々な情報を集め、自分の予算に合ったプランを設計したのですが、本経験談に基づいて、皆さんに役立つ情報をお伝えしたいと思い、本連載を始めることにしました。
住宅を購入する際、ローンの返済額や期間、金利などは重要な要素となります。
しかし、初めての方にとってはどのようにすれば良いのか分からないこともあるかと思います。私自身もそうでしたし、その時に困った経験もありました。
そこで、本記事ではPythonを用いた住宅ローンのシミュレーションについて詳しくご紹介していきます。
具体的な手順や重要なポイントを分かりやすく解説することで、皆さんが自分に合ったローンプランを見つけるお手伝いができればと思っています。
本記事の構成は以下の通りとなります。
それでは、さっそく住宅ローンのシミュレーションについて詳しく見ていきましょう!
1.Pythonの実行環境
Pythonの実行環境は以下の通りとなります。
Pythonista v.3.4
Package:Numpy-financial(https://pypi.org/project/numpy-financial/)
2.償還表の概要
住宅ローンの償還表は毎月の返済額とその内訳(当該月の元金、利息、返済済元金、残債等)を返済初月から最終月まで全て記載したものとなります。
また、住宅ローンの返済方法は「元利均等返済」と「元金均等返済」の2つがございます。
元利均等返済
毎月の返済額が一定となる返済方法元金均等返済
毎月の返済額の内の元金の額が一定となる返済方法
詳細はシミュレーションで取り扱うことでイメージが膨らむかと思います。
3.償還表(元利均等返済)の作成仕様
金利(%)、返済期間(月)、借入額(万円)をユーザーにて入力頂く必要がございます。返済期間の月数だけレコードを生成致します。
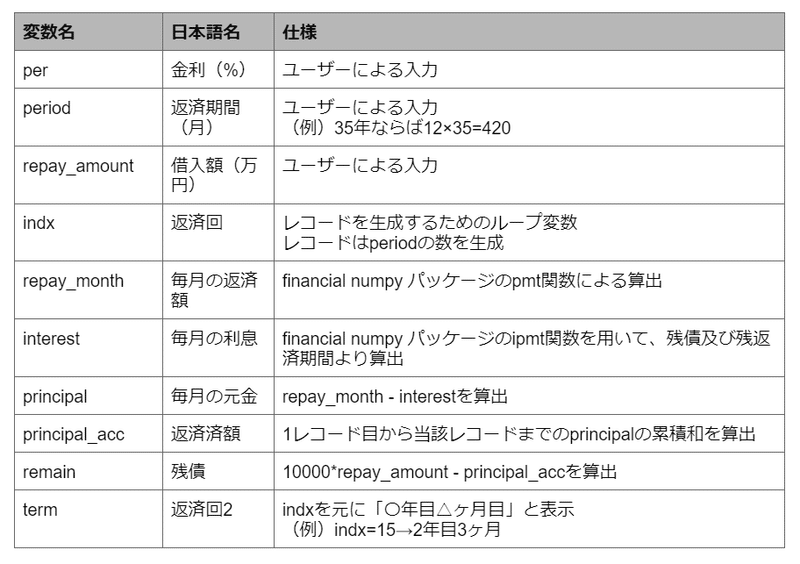
4.償還表(元利均等返済)の作成プログラム及び実行結果
import numpy as np
import numpy_financial as npf
import pandas as pd
import math
##############################
#### 基本情報(元利均等返済) ####
##############################
# 金利
per = 0.8
# 返済期間(単位:月)
period = int(12*35)
# 借入額(単位:万)
repay_amount = 4000
##### 入力ここまで #####
# 毎月の返済額
repay_month = math.floor(npf.pmt(0.01*per/12, period, -repay_amount*10000))
# 各種初期化
principal_acc = 0
# 空のデータフレーム
kihon = pd.DataFrame()
# 関数定義
def create_term(x):
if x % 12 == 0:
y = str(math.ceil(x/12))+'年目12ヶ月目'
else:
y = str(math.ceil(x/12))+'年目'+str(x%12)+'ヶ月目'
return y
# 償還表作成
for indx in range(1, period+1):
if indx == 1:
remain = repay_amount * 10000
# 毎月の利息
interest = math.floor(npf.ipmt(0.01*per/12, 1, period-indx+1, -remain))
# 毎月の元金
principal = repay_month - interest
# 返済済額
principal_acc += principal
# 残債
remain = repay_amount*10000 - principal_acc
kihon_new = pd.DataFrame([[indx, repay_month, interest, principal, principal_acc, remain]],
columns=["indx", "repay_month", "interest", "principal", "principal_acc", "remain"])
kihon = pd.concat([kihon, kihon_new])
# 最終月の処理
kihon['term'] = kihon['indx'].apply(create_term)
kihon.loc[kihon["indx"]==period, "principal"] = kihon.loc[kihon["indx"]==period-1, "remain"]
kihon.loc[kihon["indx"]==period, "repay_month"] = kihon.loc[kihon["indx"]==period, "principal"] + kihon.loc[kihon["indx"]==period, "interest"]
kihon.loc[kihon["indx"]==period, "principal_acc"] = repay_amount*10000
kihon.loc[kihon["indx"]==period, "remain"] = 0
print(kihon.iloc[0:12])
print(kihon.iloc[period-12:period])
print("#### 金利:", per, "%、返済期間:", period, "ヶ月、総返済額:", repay_amount, "万円によるシミュレーション結果 ####")
print("総返済額は", '¥{:,.0f}'.format(kihon["repay_month"].sum()), "です。")
print("総利息額は", '¥{:,.0f}'.format(kihon["interest"].sum()), "です。")
実行結果は以下になります。上から順番に返済開始1年間の償還表、返済終了間際の1年間の償還表、総返済額及び総利息額を出力致しました。
<実行結果>
indx repay_month interest principal principal_acc remain term
0 1 109224 26666 82558 82558 39917442 1年目1ヶ月目
0 2 109224 26611 82613 165171 39834829 1年目2ヶ月目
0 3 109224 26556 82668 247839 39752161 1年目3ヶ月目
0 4 109224 26501 82723 330562 39669438 1年目4ヶ月目
0 5 109224 26446 82778 413340 39586660 1年目5ヶ月目
0 6 109224 26391 82833 496173 39503827 1年目6ヶ月目
0 7 109224 26335 82889 579062 39420938 1年目7ヶ月目
0 8 109224 26280 82944 662006 39337994 1年目8ヶ月目
0 9 109224 26225 82999 745005 39254995 1年目9ヶ月目
0 10 109224 26169 83055 828060 39171940 1年目10ヶ月目
0 11 109224 26114 83110 911170 39088830 1年目11ヶ月目
0 12 109224 26059 83165 994335 39005665 1年目12ヶ月目
indx repay_month interest principal principal_acc remain term
0 409 109224 869 108355 38803378 1196622 35年目1ヶ月目
0 410 109224 797 108427 38911805 1088195 35年目2ヶ月目
0 411 109224 725 108499 39020304 979696 35年目3ヶ月目
0 412 109224 653 108571 39128875 871125 35年目4ヶ月目
0 413 109224 580 108644 39237519 762481 35年目5ヶ月目
0 414 109224 508 108716 39346235 653765 35年目6ヶ月目
0 415 109224 435 108789 39455024 544976 35年目7ヶ月目
0 416 109224 363 108861 39563885 436115 35年目8ヶ月目
0 417 109224 290 108934 39672819 327181 35年目9ヶ月目
0 418 109224 218 109006 39781825 218175 35年目10ヶ月目
0 419 109224 145 109079 39890904 109096 35年目11ヶ月目
0 420 109168 72 109096 40000000 0 35年目12ヶ月目
#### 金利: 0.8 %、返済期間: 420 ヶ月、総返済額: 4000 万円によるシミュレーション結果 ####
総返済額は ¥45,874,024 です。
総利息額は ¥5,874,024 です。
5.償還表(元金均等返済)の作成仕様
金利(%)、返済期間(月)、借入額(万円)をユーザーにて入力頂く必要がございます。返済期間の月数だけレコードを生成致します。
尚、毎月の返済額、毎月の元金の算出方法が元利均等返済とは異なります。
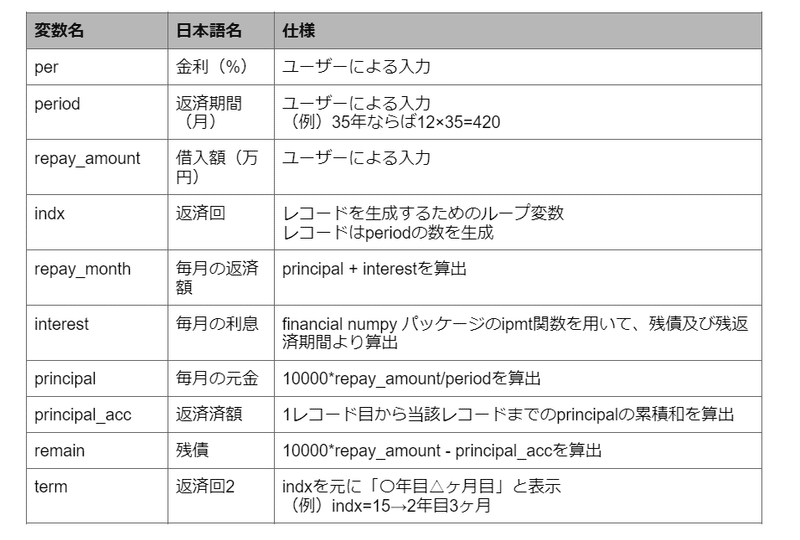
6.償還表(元金均等返済)の作成プログラム及び実行結果
import numpy as np
import numpy_financial as npf
import pandas as pd
import math
##############################
#### 基本情報(元金均等返済) ####
##############################
# 金利(%)
per = 0.8
# 返済期間(単位:月)
period = int(12*35)
# 借入額(単位:万)
repay_amount = 4000
##### 入力ここまで #####
# 毎月の元金
principal = int(repay_amount*10000 / period)
# 各種初期化
principal_acc = 0
kihon = pd.DataFrame()
# 関数定義
def create_term(x):
if x % 12 == 0:
y = str(math.ceil(x/12))+'年目12ヶ月目'
else:
y = str(math.ceil(x/12))+'年目'+str(x%12)+'ヶ月目'
return y
# 償還表作成
for indx in range(1, period+1):
if indx == 1:
remain = repay_amount * 10000
# 毎月の利息
interest = math.floor(npf.ipmt(0.01*per/12, 1, period-indx+1, -remain))
# 毎月の返済額
repay_month = principal + interest
# 返済済額
principal_acc += principal
# 残債
remain = repay_amount*10000 - principal_acc
kihon_new = pd.DataFrame([[indx, repay_month, interest, principal, principal_acc, remain]],
columns=["indx", "repay_month", "interest", "principal", "principal_acc", "remain"])
kihon = pd.concat([kihon, kihon_new])
# 最終月の処理
kihon['term'] = kihon['indx'].apply(create_term)
kihon.loc[kihon["indx"]==period, "principal"] = kihon.loc[kihon["indx"]==period-1, "remain"]
kihon.loc[kihon["indx"]==period, "repay_month"] = kihon.loc[kihon["indx"]==period, "principal"] + kihon.loc[kihon["indx"]==period, "interest"]
kihon.loc[kihon["indx"]==period, "principal_acc"] = repay_amount*10000
kihon.loc[kihon["indx"]==period, "remain"] = 0
print(kihon.iloc[0:12])
print(kihon.iloc[period-12:period])
print("#### 金利:", per, "%、返済期間:", period, "ヶ月、総返済額:", repay_amount, "万円によるシミュレーション結果 ####")
print("総返済額は", '¥{:,.0f}'.format(kihon["repay_month"].sum()), "です。")
print("総利息額は", '¥{:,.0f}'.format(kihon["interest"].sum()), "です。")
実行結果は以下になります。
上から順番に返済開始1年間の償還表、返済終了間際の1年間の償還表、総返済額及び総利息額を出力致しました。
元金均等返済よりも元利均等返済の方が約27万程度安くなっていることが分かります。
ただし、元金均等返済は金融機関によっては取り扱いがない場合があるため、注意が必要です。
<実行結果>
indx repay_month interest principal principal_acc remain term
0 1 121904 26666 95238 95238 39904762 1年目1ヶ月目
0 2 121841 26603 95238 190476 39809524 1年目2ヶ月目
0 3 121777 26539 95238 285714 39714286 1年目3ヶ月目
0 4 121714 26476 95238 380952 39619048 1年目4ヶ月目
0 5 121650 26412 95238 476190 39523810 1年目5ヶ月目
0 6 121587 26349 95238 571428 39428572 1年目6ヶ月目
0 7 121523 26285 95238 666666 39333334 1年目7ヶ月目
0 8 121460 26222 95238 761904 39238096 1年目8ヶ月目
0 9 121396 26158 95238 857142 39142858 1年目9ヶ月目
0 10 121333 26095 95238 952380 39047620 1年目10ヶ月目
0 11 121269 26031 95238 1047618 38952382 1年目11ヶ月目
0 12 121206 25968 95238 1142856 38857144 1年目12ヶ月目
indx repay_month interest principal principal_acc remain term
0 409 95999 761 95238 38952342 1047658 35年目1ヶ月目
0 410 95936 698 95238 39047580 952420 35年目2ヶ月目
0 411 95872 634 95238 39142818 857182 35年目3ヶ月目
0 412 95809 571 95238 39238056 761944 35年目4ヶ月目
0 413 95745 507 95238 39333294 666706 35年目5ヶ月目
0 414 95682 444 95238 39428532 571468 35年目6ヶ月目
0 415 95618 380 95238 39523770 476230 35年目7ヶ月目
0 416 95555 317 95238 39619008 380992 35年目8ヶ月目
0 417 95491 253 95238 39714246 285754 35年目9ヶ月目
0 418 95428 190 95238 39809484 190516 35年目10ヶ月目
0 419 95365 127 95238 39904722 95278 35年目11ヶ月目
0 420 95341 63 95278 40000000 0 35年目12ヶ月目
#### 金利: 0.8 %、返済期間: 420 ヶ月、総返済額: 4000 万円によるシミュレーション結果 ####
総返済額は ¥45,613,126 です。
総利息額は ¥5,613,126 です。
7.結言
住宅ローンの総返済額、総利息額のシミュレーションを元利均等返済、元金均等返済それぞれのケースで行いましたがいかがでしたでしょうか。
本プログラムが皆さんの予算にあったプラン設計の一助となれば幸いです。
今後については下記の内容の投稿を予定しております。
繰上げ返済のシミュレーション
繰上げ返済を実施することによって、総利息額は幾分か軽減することが可能です。繰上げ返済額やそのタイミングによりけりですので、そちらのシミュレーションを行います。金利上昇時のシミュレーション
住宅ローンを組む際の選択肢の一つとして固定金利、変動金利がございます。今後の経済情勢が不透明な状況であるため金利の上昇が不安な方もおられると思います。そのような方に向けて金利の上昇開始時、上昇幅を踏まえたシミュレーションを行います。
次回以降もご期待頂ければと存じます。
尚、本プログラムはご自身の責任においてご利用頂けますようお願いいたします。
免責事項は以下の記事に記載しております。
ここまでお読みいただき、ありがとうございました!
この記事が少しでも参考になりましたら「スキ」を押していただけると幸いです!
これまでの記事はこちら!
株式会社分析屋について
弊社が作成を行いました分析レポートを、鎌倉市観光協会様HPに掲載いただきました。
ホームページはこちら。
noteでの会社紹介記事はこちら。
【データ分析で日本を豊かに】
分析屋はシステム分野・ライフサイエンス分野・マーケティング分野の知見を生かし、多種多様な分野の企業様のデータ分析のご支援をさせていただいております。 「あなたの問題解決をする」をモットーに、お客様の抱える課題にあわせた解析・分析手法を用いて、問題解決へのお手伝いをいたします!
【マーケティング】
マーケティング戦略上の目的に向けて、各種のデータ統合及び加工ならびにPDCAサイクル運用全般を支援や高度なデータ分析技術により複雑な課題解決に向けての分析サービスを提供いたします。
【システム】
アプリケーション開発やデータベース構築、WEBサイト構築、運用保守業務などお客様の問題やご要望に沿ってご支援いたします。
【ライフサイエンス】
機械学習や各種アルゴリズムなどの解析アルゴリズム開発サービスを提供いたします。過去には医療系のバイタルデータを扱った解析が主でしたが、今後はそれらで培った経験・技術を工業など他の分野の企業様の問題解決にも役立てていく方針です。
【SES】
SESサービスも行っております。